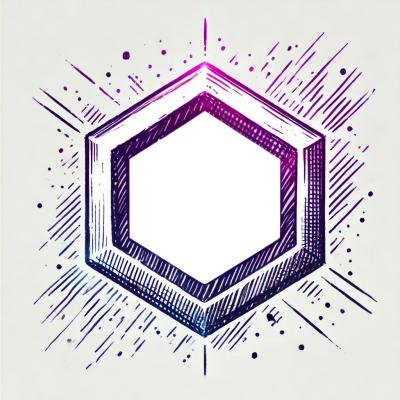
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
node-hashtable
Advanced tools
This is a simple hashtable, all written in node, to help you access and store your data over multiple workers or modules. It will provide the abstraction to access it through workers (cluster).
A simple hashtable written in node (not meant for production). It will help you share data over your node clusters or modules.
npm install node-hashtable
With cluster:
var hashTable = require("node-hashtable");
var cluster = require("cluster");
var numCPUs = require('os').cpus().length;
if(cluster.isMaster) {
hashTable.set("test", "Hello World");
// Fork workers.
for (var i = 0; i < numCPUs; i++) {
cluster.fork();
}
}
else if(cluster.isWorker) {
hashTable.get("test", function(data) { //Callback is required!
console.log(data); //output -> "Hello World"
});
}
Without cluster:
var hashTable = require("node-hashtable");
hashTable.set("test", "Hello World");
var data = hashTable.get("test"); //In this case, callback is not required.
console.log(data); //output -> "Hello World"
This function will set data to a key. If your using a cluster, you should create the callback function!
hashTable.set("key", data, function(){
//Do stuff
});
This function will return data. In this case, if using with a cluster, you NEED to create the callback!
hashTable.get("key", function(data){
//Do stuff
});
Diferent from .set, this will append data to the key. Be carefull when appending objects.
hashTable.add("key", "more stuff", function(){
//Do stuff
});
This will find the property you want to update inside the key, and update it.
hashTable.update("key", {prop: 'new'}, function(){
//Do stuff
});
No explanations needed...
hashTable.delete("key", function(){
//Do stuff
});
It will return a hash for your data.
hashTable.createHash(data, function(hash){
hashTable.set(hash, data, function(){
//Do stuff
});
});
It will return a random hash key.
hashTable.createKey(data, function(key){
hashTable.set(key, data, function(){
//Memo your key!
});
});
This function will clear the entire hash table.
hashTable.createKey(function(){
//Do stuff
});
FAQs
This is a simple hashtable, all written in node, to help you access and store your data over multiple workers or modules. It will provide the abstraction to access it through workers (cluster).
The npm package node-hashtable receives a total of 0 weekly downloads. As such, node-hashtable popularity was classified as not popular.
We found that node-hashtable demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.