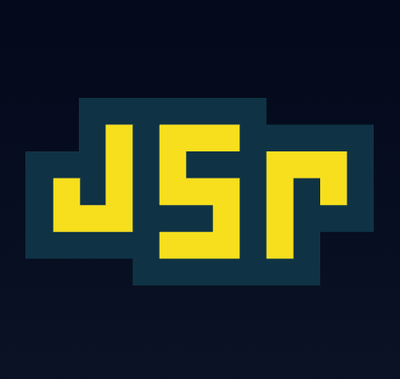
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
node-hid
supports Node.js v6 and upwards. For versions 0.10 and 0.12 and v4,
you will need to build from source. The platforms, architectures and node versions node-hid
supports are the following.
Those with checks we provide pre-built binaries, for the others you will need to compile.
We strive to make node-hid
cross-platform so there's a good chance any
combination not listed here will compile and work.
Platform / Arch | Node v6.x | Node v7.x | Node v8.x | Node v9.x | Node v10.x |
---|---|---|---|---|---|
Windows / x86 | ☑ | ☑ | ☑ | ☑ | ☑ |
Windows / x64 | ☑ | ☑ | ☑ | ☑ | ☑ |
Mac OSX / x64 | ☑ | ☑ | ☑ | ☑ | ☑ |
Linux / x64 | ☑ | ☑ | ☑ | ☑ | ☑ |
Linux / ia32¹ | ☐ | ☐ | ☐ | ☐ | ☐ |
Linux / ARM v6¹ | ☐ | ☐ | ☐ | ☐ | ☐ |
Linux / ARM v7¹ | ☐ | ☐ | ☐ | ☐ | ☐ |
Linux / ARM v8¹ | ☐ | ☐ | ☐ | ☐ | ☐ |
Linux / MIPSel¹ | ☐ | ☐ | ☐ | ☐ | ☐ |
Linux / PPC64¹ | ☐ | ☐ | ☐ | ☐ | ☐ |
¹ ia32, ARM, MIPSel and PPC64 platforms are known to work but are not currently part of our test or build matrix. ARM v4 and v5 was dropped from Node.js after Node v0.10.
Platform / Arch | v1.0 | v1.2 | v1.3 | v1.4 | v1.5 | v1.6 | v1.7 | v1.8² |
---|---|---|---|---|---|---|---|---|
Windows / x86 | ☑ | ☑ | ☑ | ☑ | ☑ | ☑ | ☑ | ☑ |
Windows / x64 | ☑ | ☑ | ☑ | ☑ | ☑ | ☑ | ☑ | ☑ |
Mac OSX / x64 | ☑ | ☑ | ☑ | ☑ | ☑ | ☑ | ☑ | ☑ |
Linux / x64 | ☑ | ☑ | ☑ | ☑ | ☑ | ☑ | ☑ | ☑ |
Linux / ia32¹ | ☐ | ☐ | ☐ | ☐ | ☐ | ☐ | ☐ | ☐ |
Linux / ARM v6¹ | ☐ | ☐ | ☐ | ☐ | ☐ | ☐ | ☐ | ☐ |
Linux / ARM v7¹ | ☐ | ☐ | ☐ | ☐ | ☐ | ☐ | ☐ | ☐ |
Linux / ARM v8¹ | ☐ | ☐ | ☐ | ☐ | ☐ | ☐ | ☐ | ☐ |
Linux / MIPSel¹ | ☐ | ☐ | ☐ | ☐ | ☐ | ☐ | ☐ | ☐ |
Linux / PPC64¹ | ☐ | ☐ | ☐ | ☐ | ☐ | ☐ | ☐ | ☐ |
² Electron v1.8 currently has issues but prebuilt binaries are provided.
For most "standard" use cases (node v4.x on mac, linux, windows on a x86 or x64 processor), node-hid
will install nice and easy with a standard:
npm install node-hid
We are using prebuild to compile and post binaries of the library for most common use cases (linux, mac, windows on standard processor platforms). If you are on a special case, node-hid
will work, but it will compile the binary when you install.
If node-hid
doesn't have a pre-built binary for your system
(e.g. Linux on Raspberry Pi),
node-gyp
is used to compile node-hid
locally. It will need the pre-requisites
listed in Compling from source below.
In the src/
directory, various JavaScript programs can be found
that talk to specific devices in some way. Some interesting ones:
show-devices.js
- display all HID devices in the systemtest-ps3-rumbleled.js
- Read PS3 joystick and control its LED & rumblerstest-powermate.js
- Read Griffin PowerMate knob and change its LEDtest-blink1.js
- Fade colors on blink(1) RGB LEDtest-bigredbutton.js
- Read Dreamcheeky Big Red Buttontest-teensyrawhid.js
- Read/write Teensy running RawHID "Basic" Arduino sketchTo try them out, run them like node src/showdevices.js
from within the node-hid directory.
var HID = require('node-hid');
var devices = HID.devices();
devices
will contain an array of objects, one for each HID device
available. Of particular interest are the vendorId
and
productId
, as they uniquely identify a device, and the
path
, which is needed to open a particular device.
Sample output:
HID.devices();
{ vendorId: 10168,
productId: 493,
path: 'IOService:/AppleACPIPl...HIDDevice@14210000,0',
serialNumber: '20002E8C',
manufacturer: 'ThingM',
product: 'blink(1) mk2',
release: 2,
interface: -1,
usagePage: 65280,
usage: 1 },
{ vendorId: 1452,
productId: 610,
path: 'IOService:/AppleACPIPl...Keyboard@14400000,0',
serialNumber: '',
manufacturer: 'Apple Inc.',
product: 'Apple Internal Keyboard / Trackpad',
release: 549,
interface: -1,
usagePage: 1,
usage: 6 },
<and more>
HID.devices()
and new HID.HID()
for detecting device plug/unplugBoth HID.devices()
and new HID.HID()
are relatively costly, each causing a USB (and potentially Bluetooth) enumeration. This takes time and OS resources. Doing either can slow down the read/write that you do in parallel with a device, and cause other USB devices to slow down too. This is how USB works.
If you are polling HID.devices()
or doing repeated new HID.HID(vid,pid)
to detect device plug / unplug, consider instead using node-usb-detection. node-usb-detection
uses OS-specific, non-bus enumeration ways to detect device plug / unplug.
Before a device can be read from or written to, it must be opened.
The path
can be determined by a prior HID.devices() call.
Use either the path
from the list returned by a prior call to HID.devices()
:
var device = new HID.HID(path);
or open the first device matching a VID/PID pair:
var device = new HID.HID(vid,pid);
The device
variable will contain a handle to the device.
If an error occurs opening the device, an exception will be thrown.
A node-hid
device is an EventEmitter
.
While it shares some method names and usage patterns with
Readable
and Writable
streams, it is not a stream and the semantics vary.
For example, device.write
does not take encoding or callback args and
device.pause
does not do the same thing as readable.pause
.
There is also no pipe
method.
If you need to filter down the HID.devices()
list, you can use
standard Javascript array techniques:
var devices = HID.devices();
var deviceInfo = devices.find( function(d) {
var isTeensy = d.vendorId===0x16C0 && d.productId===0x0486;
return isTeensy && d.usagePage===0xFFAB && d.usage===0x200;
});
if( deviceInfo ) {
var device = new HID.HID( deviceInfo.path );
// ... use device
}
To receive FEATURE reports, use device.getFeatureReport()
.
To receive INPUT reports, use device.on("data",...)
.
A node-hid
device is an EventEmitter.
Reading from a device is performed by registering a "data" event handler:
device.on("data", function(data) {});
You can also listen for errors like this:
device.on("error", function(err) {});
For FEATURE reports:
var buf = device.getFeatureReport(reportId, reportLength)
Notes:
device.on("data")
are asynchronousdevice.getFeatureReport()
are synchronousdevice.close()
To send FEATURE reports, use device.sendFeatureReport()
.
To send OUTPUT reports, use device.write()
.
All writing is synchronous.
device.write([0x00, 0x01, 0x01, 0x05, 0xff, 0xff]);
device.sendFeatureReport( [0x01, 'c', 0, 0xff,0x33,0x00, 70,0, 0] );
Notes:
device.write()
and device.sendFeatureReport()
return number of bytes writtenwrite()
should be the reportId.devices = HID.devices()
HID.setDriverType(type)
type
can be "hidraw"
or "libusb"
, defaults to "hidraw"
node-hid@^0.7.1
due to incompatibilities with prebuild
.device = new HID.HID(path)
device = new HID.HID(vid,pid)
device.on('data', function(data) {} )
data
- Buffer - the data read from the devicedevice.on('error, function(error) {} )
error
- The error Object emitteddevice.write(data)
data
- the data to be synchronously written to the devicedevice.close()
device.pause()
data
events.device.resume()
This method will cause the HID device to resume emmitting data
events.
If no listeners are registered for the data
event, data will be lost.
When a data
event is registered for this HID device, this method will
be automatically called.
device.read(callback)
callback
is of the form callback(err, data)
device.readSync()
device.readTimeout(time_out)
time_out
- timeout in millisecondsdevice.sendFeatureReport(data)
data
- data of HID feature report, with 0th byte being report_id ([report_id,...]
)device.getFeatureReport(report_id, report_length)
report_id
- HID feature report id to getreport_length
- length of reportIn general you cannot access USB HID keyboards or mice.
The OS owns these devices.
For reasons similar to mice & keyboards it appears you can't access this controller on Windows 10.
hid_write()
Because of a limitation in the underlying hidapi
library, if you are using hid_write()
you should prepend a byte to any data buffer, e.g.
var device = new HID.HID(vid,pid);
var buffer = Array(64).fill(0x33); // device has 64-byte report
if(os.platform === 'win32') {
buffer.unshift(0); // prepend throwaway byte
}
(NOTE: The multi-driver feature of HID.setDriverType()
is currently disabled. See issue #242 )
By default as of node-hid@0.7.0
, the hidraw driver is used to talk to HID devices. Before node-hid@0.7.0
, the more older but less capable libusb driver was used. With hidraw
Linux apps can now see usage
and usagePage
attributes of devices.
If you would still like to use the libusb
driver, then you can do either:
npm install node-hid@0.5.7
or:
npm install node-hid --build-from-source --driver=libusb
Most Linux distros use udev
to manage access to physical devices,
and USB HID devices are normally owned by the root
user.
To allow non-root access, you must create a udev rule for the device,
based on the devices vendorId and productId.
This rule is a text file placed in /etc/udev/rules.d
.
For an example HID device (say a blink(1) light with vendorId = 0x27b8 and productId = 0x01ed,
the rules file to support both hidraw
and libusb
would look like:
SUBSYSTEM=="input", GROUP="input", MODE="0666"
SUBSYSTEM=="usb", ATTRS{idVendor}=="27b8", ATTRS{idProduct}=="01ed", MODE:="666", GROUP="plugdev"
KERNEL=="hidraw*", ATTRS{idVendor}=="27b8", ATTRS{idProduct}=="01ed", MODE="0666", GROUP="plugdev"
Note that the values for idVendor and idProduct must be in hex and lower-case.
Save this file as /etc/udev/rules.d/51-blink1.rules
, unplug the HID device,
and reload the rules with:
sudo udevadm control --reload-rules
For a complete example, see the blink1 udev rules.
To compile & develop locally or if prebuild
cannot download a pre-built
binary for you, you will need the following tools:
All OSes:
node-gyp
installed globally: npm install -g node-gyp
Linux (kernel 2.6+) : install examples shown for Ubuntu
apt install build-essential git
apt install gcc-4.8 g++-4.8 && export CXX=g++-4.8
sudo apt install libusb-1.0-0 libusb-1.0-0-dev
yum install libusbx-devel
Mac OS X 10.8+
Windows XP, 7, 8, 10
npm install --global windows-build-tools
%USERPROFILE%\.windows-build-tools\python27
to PATH
,
like PowerShell: $env:Path += ";$env:USERPROFILE\.windows-build-tools\python27"
node-hid
from source for your project:npm install node-hid --build-from-source
node-hid
for development:For example:
git clone https://github.com/node-hid/node-hid.git
cd node-hid # must change into node-hid directory
npm run prepublish # get the needed hidapi submodule
npm install --build-from-source # rebuilds the module with C code
node ./src/show-devices.js
You will see some warnings from the C compiler as it compiles
hidapi (the underlying C library node-hid
uses).
This is expected.
For ease of development, there are also the scripts:
npm run gypclean # "node-gyp clean" clean gyp build directory
npm run gypconfigure # "node-gyp configure" configure makefiles
npm run gypbuild # "node-gyp build" build native code
node-hid
in Electron projectsIn your electron project, add electron-rebuild
to your devDependencies
.
Then in your package.json scripts
add:
"postinstall": "electron-rebuild --force"
This will cause npm
to rebuild node-hid
for the version of Node that is in Electron.
If you get an error similar to The module "HID.node" was compiled against a differnt version of Node.js
then electron-rebuild
hasn't been run and Electron is trying to use node-hid
not built for it.
If you want a specific version of electron, do something like:
"postinstall": "electron-rebuild -v 0.36.5 --force -m . -w node-hid"
If using node-hid
with webpack
, you may find it useful to list node-hid
as an external in your webpack-config.js
:
externals: {
"node-hid": 'commonjs node-hid'
}
(You can see an example of this in Blink1Control2's webpack-config.js
node-hid
in NW.js projects(TBD)
Please use the node-hid github issues page for support questions and issues.
FAQs
USB HID device access library
The npm package node-hid receives a total of 73,782 weekly downloads. As such, node-hid popularity was classified as popular.
We found that node-hid demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.