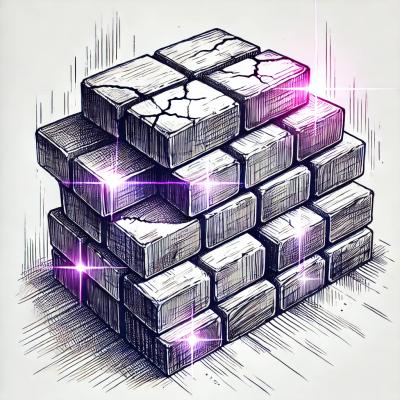
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
node-kraken-api
Advanced tools
Interfaces with the Kraken cryptocurrency exchange API. Observes rate limits. Parses response JSON, converts stringed numbers, and normalizes timestamps. Facilitates persistent data syncing.
Interfaces with the Kraken cryptocurrency exchange API. Observes rate limits. Parses response JSON, converts stringed numbers, and normalizes timestamps. Facilitates persistent data syncing.
Use of the syncing feature is advisable when concurrent realtime data is required. For public calls, data is repeatedly requested upon receipt of data; rate is limited automatically. For private calls, rate is managed (according to the rate limit specificiations listed in the Kraken API docs) such that calls may be performed continuously without triggering any rate limit violations.
Syncing is also useful for querying new updates only by modifying the call options upon each response. This can be done within a listener callback provided to the module. Some methods provide a 'since' parameter that can be used for querying only new data. See below for an example.
Additionally, sync instances can be used to store other kinds of data. See below for an example.
Node.JS version 8.7.0 or above.
npm i node-kraken-api
The following command will test the package for errors (using the jest library). Testing may take a few minutes to complete.
Note: In order for authenticated testing to occur, a valid auth.json file must be available in the root directory of the package. Please see the configuration instructions below.
Creating an auth.json file for authenticated testing:
NOTE: replace 'nano' with your preferred editor NOTE: use a read-only key to be safe
nano /path/to/node_modules/node-kraken-api/auth.json
Installing the jest library:
npm i --prefix /path/to/node_modules/node-kraken-api jest
Running the testing scripts:
npm test --prefix /path/to/node_modules/node-kraken-api
Loading the module:
const kraken = require('node-kraken-api')
Public client instantiation:
const api = kraken()
Private client instantiation (with authenticated configuration):
const api = kraken({ key: '****', secret: '****', tier: '****' })
Or:
/* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
* Note: In this example, OTP is set during instantiation. *
* This is advisable only if the two-factor password for *
* this API key is static. Otherwise, use api.setOTP() *
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * */
const api = kraken({
key: '****',
secret: '****',
tier: '****',
otp: '****'
})
Instantiation with any number of configuration settings (see configuration):
const api = kraken(require('./config.json'))
Or:
const api = kraken({
key: '****',
secret: '****',
tier: '****',
parse: { dates: false }
})
Making a single call:
Using promises:
api.call('Time')
.then(data => console.log(data))
.catch(err => console.error(err))
Using callbacks:
api.call('Time', (err, data) => {
if (err) console.error(err)
else console.log(data)
})
Using promises (with Kraken method options):
api.call('Depth', { pair: 'XXBTZUSD', count: 1 })
.then(data => console.log(data))
.catch(err => console.error(err))
Using callbacks (with Kraken method options):
api.call('Depth', { pair: 'XXBTZUSD', count: 1 },
(err, data) => {
if (err) console.error(err)
else console.log(data)
}
)
Using a one-time password (if enabled):
/* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
* NOTE: due to call queueing functionality and rate limiting, *
* OTP may need to be set again if the call has not been executed *
* before the password decays. This shouldn't be a problem unless *
* there have been a very large volume of calls sent to the queue. *
* *
* Additionally, depending on settings.retryCt, calls with errors *
* will be re-queued. *
* *
* As such, it is best to continuously call setOTP with each new *
* password until an error or response has been received. *
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * */
api.setOTP(158133)
api.call('AddOrder', {
pair: 'XXBTZUSD',
type: 'buy',
price: 5000,
volume: 1
}).then(console.log).catch(console.log)
Creating a sync object:
const timeSync = api.sync('Time')
// logs {}
console.log(timeSync.data)
// logs { unixtime: 1528607559000, rfc1123: 1528607559000 }
setTimeout(() => console.log(timeSync.data), 5000)
Creating a sync object with a custom update interval:
// updates every second
const timeSync = api.sync('Time', 1000)
// logs {}
console.log(timeSync.data)
// logs { unixtime: 1528607559000, rfc1123: 1528607559000 }
setTimeout(() => console.log(timeSync.data), 5000)
Using syncing promises:
const api = require('./')()
const timeSync = api.sync('Time')
let i = 0
const logUpdates = async () => {
while(i++ < 20) {
try {
console.log(await timeSync.once())
} catch(e) {
console.error(e)
}
}
}
logUpdates()
Creating a sync object (using a listener callback):
const timeSync = api.sync('Time',
(err, data) => {
if (err) console.error(err)
else if (data) console.log(data)
}
)
Adding a listener callback after creation:
const timeSync = api.sync('Time')
timeSync.addListener((err, data) => {
if (err) console.error(err)
else if (data) console.log(data)
})
Adding a once listener via Promises:
const timeSync = api.sync('Time')
timeSync.once()
.then(data => console.log(data))
.catch(err => console.error(err))
Adding a once listener callback:
const timeSync = api.sync('Time')
timeSync.once((err, data) => {
if (err) console.error(err)
else if (data) console.log(data)
})
Closing a sync operation:
const timeSync = api.sync('Time')
timeSync.addListener(
(err, data) => {
// stops executing (and syncing) after ~5000 ms
if (err) console.error(err)
else if (data) console.log(data)
}
)
setTimeout(timeSync.close, 5000)
Re-opening a sync operation:
const timeSync = api.sync('Time')
timeSync.addListener(
(err, data) => {
// stops executing (and syncing) after ~5000 ms
// starts executing (and syncing) again after ~10000 ms
if (err) console.error(err)
else if (data) console.log(data)
}
)
setTimeout(timeSync.close, 5000)
setTimeout(timeSync.open, 10000)
Removing a sync listener callback:
const timeSync = api.sync('Time')
const listener = (err, data) => {
// stops executing after ~5000 ms
// (timeSync will continue to stay up to date)
if (err) console.error(err)
else if (data) console.log(data)
}
timeSync.addListener(listener)
setTimeout(() => timeSync.removeListener(listener), 5000)
Using a listener within a sync instance may be used for tracking new data only. For example, methods such as 'Trades' or 'OHLC' respond with a 'last' property to allow for querying new updates.
Logging new trades only:
const tradesSync = api.sync(
'Trades',
{ pair: 'XXBTZUSD' },
(err, data, instance) => {
// logs only new trades
if (err) {
console.error(err)
} else if (data) {
console.log(data)
instance.options.since = data.last
}
}
)
Sync object data may be custom tailored for various use cases by using an event listener. Event listeners are provided with a reference to the instance, so they can be defined to transform received data in any way and store it within a custom property.
Creating a realtime historical trades tracker:
const tradesHistory = api.sync(
'Trades',
{ pair: 'XXBTZUSD' },
(err, data, instance) => {
if (data) {
if (!instance.hist) {
instance.hist = []
}
if (data.last !== instance.options.since) {
if (data.XXBTZUSD.forEach) {
data.XXBTZUSD.forEach(trade => instance.hist.push(trade))
}
instance.options.since = data.last
}
}
}
)
tradesHistory.once()
.then(data => console.log(tradesHistory.hist))
.catch(err => console.error(err))
Creating a realtime simple moving average (with safe float operations):
NOTE: OHLC calls are set to a 60s sync interval by default. This may be changed either in the settings configuration, during instance creation, or by changing the instance's interval
property.
const twentyPeriodSMA = api.sync(
'OHLC',
{ pair: 'XXBTZUSD' },
(err, data, instance) => {
if (data) {
if (!instance.bars) instance.bars = []
if (data.last !== instance.options.since) {
if (data.XXBTZUSD.forEach) {
data.XXBTZUSD.forEach(bar => instance.bars.push(bar))
}
instance.options.since = data.last
}
instance.bars = instance.bars.slice(-20)
instance.value = instance.bars.reduce(
(sum, bar) => (
sum + (
(
Math.floor(bar[1] * 100) +
Math.floor(bar[2] * 100) +
Math.floor(bar[3] * 100) +
Math.floor(bar[4] * 100)
) / 4
)
),
0
) / instance.bars.length / 100
}
}
)
twentyPeriodSMA.once()
.then(data => console.log(twentyPeriodSMA.value))
.catch(err => console.error(err))
During creation of the API instance, a configuration object may be provided for authenticated calls and other options. This may be provided by using require('./config.json')
if this file has been prepared already, or by simply providing an object programmatically.
Configuration specifications are detailed in the documentation here
Please browse the Kraken API docs for information pertaining to call types and options.
Method names are found within the 'URL' subtitle in the Kraken API docs. For example: under 'Get server time', the text 'URL: https://api.kraken.com/0/public/Time' shows that the method name is 'Time'.
Alternatively, refer to the default settings in the node-kraken-api documentation. Default method types are listed here (under the 'pubMethods' and 'privMethods' properties).
Method options are found under the 'Input:' section. For example, 'Get order book' lists the following:
pair = asset pair to get market depth for
count = maximum number of asks/bids (optional)
This translates to an object such as { pair: 'XXBTZUSD', count: 10 }
, which should be used when passing method options to API calls.
You may learn more about the types of options and response data by probing the API. Use the methods 'Assets' and 'AssetPairs' to discover the naming scheme for the assets tradable via Kraken.
Versioned using SemVer. For available versions, see the Changelog.
Please raise an issue if you find any. Pull requests are welcome!
Created using npm-kraken-api (nothingisdead) for reference.
BTC donation address:
3KZw9KTCo3T5MksE7byasq3J8btmLt5BTz
This project is licensed under the MIT License - see the LICENSE file for details
0.1.0 (2018-06-24)
| Release Notes | README | | --- | --- |
| Source Code (zip) | Source Code (tar.gz) | | --- | --- |
Features:
FAQs
a typed REST/WS Node.JS client for the Kraken cryptocurrency exchange
The npm package node-kraken-api receives a total of 1,171 weekly downloads. As such, node-kraken-api popularity was classified as popular.
We found that node-kraken-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.