node-mocks-http
Advanced tools
Comparing version 1.2.7 to 1.3.0
@@ -61,2 +61,2 @@ 'use strict'; | ||
}; | ||
}; |
@@ -51,2 +51,2 @@ 'use strict'; | ||
}; | ||
}; |
@@ -0,1 +1,3 @@ | ||
'use strict'; | ||
/** | ||
@@ -8,6 +10,6 @@ * Module: http-mock | ||
var request = require('./mockRequest'); | ||
var request = require('./mockRequest'); | ||
var response = require('./mockResponse'); | ||
exports.createRequest = request.createRequest; | ||
exports.createResponse= response.createResponse; | ||
exports.createResponse = response.createResponse; |
@@ -0,1 +1,3 @@ | ||
'use strict'; | ||
/* | ||
@@ -7,13 +9,13 @@ * http://nodejs.org/api/events.html | ||
EventEmitter.prototype.addListener = function (event, listener) {}; | ||
EventEmitter.prototype.on = function (event, listener) {}; | ||
EventEmitter.prototype.once = function (event, listener) {}; | ||
EventEmitter.prototype.removeListener = function (event, listener) {}; | ||
EventEmitter.prototype.removeAllListeners = function (event) {}; | ||
EventEmitter.prototype.addListener = function () {}; | ||
EventEmitter.prototype.on = function () {}; | ||
EventEmitter.prototype.once = function () {}; | ||
EventEmitter.prototype.removeListener = function () {}; | ||
EventEmitter.prototype.removeAllListeners = function () {}; | ||
// EventEmitter.prototype.removeAllListeners = function([event]) | ||
EventEmitter.prototype.setMaxListeners = function (n) {}; | ||
EventEmitter.prototype.listeners = function (event) {}; | ||
EventEmitter.prototype.emit = function (event) {}; | ||
EventEmitter.prototype.setMaxListeners = function () {}; | ||
EventEmitter.prototype.listeners = function () {}; | ||
EventEmitter.prototype.emit = function () {}; | ||
// EventEmitter.prototype.emit = function(event, [arg1], [arg2], [...]){} | ||
module.exports = EventEmitter; | ||
module.exports = EventEmitter; |
@@ -25,6 +25,7 @@ 'use strict'; | ||
* | ||
* method - The method value, see <mockRequest._setMethod> | ||
* url - The url value, see <mockRequest._setURL> | ||
* params - The parameters, see <mockRequest._setParam> | ||
* body - The body values, , see <mockRequest._setBody> | ||
* method - The method value, see <mockRequest._setMethod> | ||
* url - The url value, see <mockRequest._setURL> | ||
* originalUrl - The originalUrl value, see <mockRequest._setOriginalUrl> | ||
* params - The parameters, see <mockRequest._setParam> | ||
* body - The body values, , see <mockRequest._setBody> | ||
*/ | ||
@@ -46,2 +47,3 @@ | ||
mockRequest.url = (options.url) ? options.url : ''; | ||
mockRequest.originalUrl = options.originalUrl || mockRequest.url; | ||
mockRequest.path = (options.url) ? url.parse(options.url).pathname : ''; | ||
@@ -114,12 +116,8 @@ mockRequest.params = (options.params) ? options.params : {}; | ||
return mockRequest.params[parameterName]; | ||
} | ||
else if (mockRequest.body[parameterName]) { | ||
} else if (mockRequest.body[parameterName]) { | ||
return mockRequest.body[parameterName]; | ||
} | ||
else if (mockRequest.query[parameterName]) { | ||
} else if (mockRequest.query[parameterName]) { | ||
return mockRequest.query[parameterName]; | ||
} | ||
else { | ||
return null; | ||
} | ||
return null; | ||
}; | ||
@@ -223,3 +221,3 @@ | ||
* | ||
* url - The request path, e.g. /my-route/452 | ||
* value - The request path, e.g. /my-route/452 | ||
* | ||
@@ -229,7 +227,24 @@ * Note: We don't validate the string. We just return it. Typically, these | ||
*/ | ||
mockRequest._setURL = function(url) { | ||
mockRequest.url = url; | ||
mockRequest._setURL = function(value) { | ||
mockRequest.url = value; | ||
}; | ||
/** | ||
* Function: _setOriginalUrl | ||
* | ||
* Sets the URL value that the client gets when the called the 'originalUrl' | ||
* property. | ||
* | ||
* Parameters: | ||
* | ||
* value - The request path, e.g. /my-route/452 | ||
* | ||
* Note: We don't validate the string. We just return it. Typically, these | ||
* do not include hostname, port or that part of the URL. | ||
*/ | ||
mockRequest._setOriginalUrl = function(value) { | ||
mockRequest.originalUrl = value; | ||
}; | ||
/** | ||
* Function: _setBody | ||
@@ -236,0 +251,0 @@ * |
@@ -30,2 +30,4 @@ 'use strict'; | ||
var EventEmitter = require('./mockEventEmitter'); | ||
var mime = require('mime'); | ||
var http = require('./node/http'); | ||
@@ -47,4 +49,10 @@ function createResponse(options) { | ||
var writableStream = options.writableStream ? new options.writableStream() : new WritableStream(); | ||
var eventEmitter = options.eventEmitter ? new options.eventEmitter() : new EventEmitter(); | ||
if (options.writableStream) { | ||
WritableStream = options.writableStream; | ||
} | ||
if (options.eventEmitter) { | ||
EventEmitter = options.eventEmitter; | ||
} | ||
var writableStream = new WritableStream(); | ||
var eventEmitter = new EventEmitter(); | ||
@@ -58,7 +66,7 @@ // create mockResponse | ||
mockResponse.cookie = function(name, value, options) { | ||
mockResponse.cookie = function(name, value, opt) { | ||
mockResponse.cookies[name] = { | ||
value: value, | ||
options: options | ||
options: opt | ||
}; | ||
@@ -114,20 +122,20 @@ | ||
var _formatData = function(a) { | ||
var _formatData = function(data) { | ||
if (typeof a === 'object') { | ||
if (typeof data === 'object') { | ||
if (a.statusCode) { | ||
mockResponse.statusCode = a.statusCode; | ||
} else if (a.httpCode) { | ||
mockResponse.statusCode = a.statusCode; | ||
if (data.statusCode) { | ||
mockResponse.statusCode = data.statusCode; | ||
} else if (data.httpCode) { | ||
mockResponse.statusCode = data.statusCode; | ||
} | ||
if (a.body) { | ||
_data = a.body; | ||
if (data.body) { | ||
_data = data.body; | ||
} else { | ||
_data = a; | ||
_data = data; | ||
} | ||
} else { | ||
_data += a; | ||
_data += data; | ||
} | ||
@@ -185,2 +193,27 @@ | ||
/** | ||
* Send given HTTP status code. | ||
* | ||
* Sets the response status to `statusCode` and the body of the | ||
* response to the standard description from node's http.STATUS_CODES | ||
* or the statusCode number if no description. | ||
* | ||
* Examples: | ||
* | ||
* mockResponse.sendStatus(200); | ||
* | ||
* @param {number} statusCode | ||
* @api public | ||
*/ | ||
mockResponse.sendStatus = function sendStatus(statusCode) { | ||
var body = http.STATUS_CODES[statusCode] || String(statusCode); | ||
mockResponse.statusCode = statusCode; | ||
mockResponse.type('txt'); | ||
return mockResponse.send(body); | ||
}; | ||
/** | ||
* Function: json | ||
@@ -219,2 +252,22 @@ * | ||
/** | ||
* Set "Content-Type" response header with `type` through `mime.lookup()` | ||
* when it does not contain "/", or set the Content-Type to `type` otherwise. | ||
* | ||
* Examples: | ||
* | ||
* res.type('.html'); | ||
* res.type('html'); | ||
* res.type('json'); | ||
* res.type('application/json'); | ||
* res.type('png'); | ||
* | ||
* @param {String} type | ||
* @return {ServerResponse} for chaining | ||
* @api public | ||
*/ | ||
mockResponse.contentType = mockResponse.type = function(type){ | ||
return mockResponse.set('Content-Type', type.indexOf('/') >= 0 ? type : mime.lookup(type)); | ||
}; | ||
/** | ||
* Function: write | ||
@@ -290,4 +343,3 @@ * | ||
val = val.map(String); | ||
} | ||
else { | ||
} else { | ||
val = String(val); | ||
@@ -384,3 +436,3 @@ } | ||
*/ | ||
mockResponse.render = function(a, b, c) { | ||
mockResponse.render = function(a, b) { | ||
@@ -424,31 +476,31 @@ _renderView = a; | ||
mockResponse.addListener = function(event, listener) { | ||
mockResponse.addListener = function() { | ||
return eventEmitter.addListener.apply(this, arguments); | ||
}; | ||
mockResponse.on = function(event, listener) { | ||
mockResponse.on = function() { | ||
return eventEmitter.on.apply(this, arguments); | ||
}; | ||
mockResponse.once = function(event, listener) { | ||
mockResponse.once = function() { | ||
return eventEmitter.once.apply(this, arguments); | ||
}; | ||
mockResponse.removeListener = function(event, listener) { | ||
mockResponse.removeListener = function() { | ||
return eventEmitter.removeListener.apply(this, arguments); | ||
}; | ||
mockResponse.removeAllListeners = function(event) { | ||
mockResponse.removeAllListeners = function() { | ||
return eventEmitter.removeAllListeners.apply(this, arguments); | ||
}; | ||
mockResponse.setMaxListeners = function(n) { | ||
mockResponse.setMaxListeners = function() { | ||
return eventEmitter.setMaxListeners.apply(this, arguments); | ||
}; | ||
mockResponse.listeners = function(event) { | ||
mockResponse.listeners = function() { | ||
return eventEmitter.listeners.apply(this, arguments); | ||
}; | ||
mockResponse.emit = function(event) { | ||
mockResponse.emit = function() { | ||
return eventEmitter.emit.apply(this, arguments); | ||
@@ -455,0 +507,0 @@ }; |
@@ -0,1 +1,3 @@ | ||
'use strict'; | ||
/* | ||
@@ -16,2 +18,2 @@ * http://nodejs.org/api/stream.html#stream_writable_stream | ||
module.exports = WritableStream; | ||
module.exports = WritableStream; |
@@ -5,17 +5,32 @@ { | ||
"description": "Mock 'http' objects for testing Express routing functions", | ||
"version": "1.2.7", | ||
"homepage": "http://www.github.com/howardabrams/node-mocks-http", | ||
"license" : "MIT", | ||
"version": "1.3.0", | ||
"homepage": "https://github.com/howardabrams/node-mocks-http", | ||
"bugs": { | ||
"url" : "https://github.com/howardabrams/node-mocks-http/issues" | ||
}, | ||
"contributors": [ | ||
{ | ||
"name" : "Howard Abrams", | ||
"email" : "howard.abrams@gmail.com", | ||
"url" : "https://github.com/howardabrams" | ||
}, | ||
{ | ||
"name" : "Johnny Estilles", | ||
"email" : "johnny.estilles@agentia.asia", | ||
"url" : "https://github.com/JohnnyEstilles" | ||
} | ||
], | ||
"license": "MIT", | ||
"keywords": [ | ||
"mock", | ||
"stub", | ||
"dummy", | ||
"nodejs", | ||
"js", | ||
"testing", | ||
"test", | ||
"http", | ||
"http mock" | ||
], | ||
"repository": { | ||
"mock", | ||
"stub", | ||
"dummy", | ||
"nodejs", | ||
"js", | ||
"testing", | ||
"test", | ||
"http", | ||
"http mock" | ||
], | ||
"repository": { | ||
"type": "git", | ||
@@ -28,9 +43,14 @@ "url": "git://github.com/howardabrams/node-mocks-http.git" | ||
}, | ||
"devDependencies":{ | ||
"nodeunit":"", | ||
"node-restify-errors":"git://github.com/m9dfukc/node-restify-errors.git" | ||
"dependencies": { | ||
"mime": "^1.3.4" | ||
}, | ||
"scripts":{ | ||
"test": "./run-tests" | ||
"devDependencies": { | ||
"eslint": "^0.18.0", | ||
"node-restify-errors": "git://github.com/m9dfukc/node-restify-errors.git", | ||
"nodeunit": "^0.9.1" | ||
}, | ||
"scripts": { | ||
"lint": "DEBUG=eslint:cli-engine node_modules/eslint/bin/eslint.js ./lib ./examples ./test", | ||
"test": "npm run lint && ./run-tests" | ||
} | ||
} |
@@ -1,4 +0,8 @@ | ||
node-mocks-http | ||
[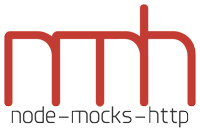](https://github.com/howardabrams/node-mocks-http) | ||
--- | ||
[](https://www.npmjs.com/package/node-mocks-http) | ||
[](https://travis-ci.org/howardabrams/node-mocks-http) | ||
[](https://gitter.im/howardabrams/node-mocks-http) | ||
Mock 'http' objects for testing [Express](http://expressjs.com/) | ||
@@ -9,4 +13,3 @@ routing functions, but could be used for testing any | ||
Installation | ||
--- | ||
## Installation | ||
@@ -17,5 +20,7 @@ This project is available as a | ||
```bash | ||
$ npm install node-mocks-http | ||
$ npm install --save-dev node-mocks-http | ||
``` | ||
> Our example includes `--save-dev` based on the assumption that **node-mocks-http** will be used as a development dependency.. | ||
After installing the package include the following in your test files: | ||
@@ -27,4 +32,3 @@ | ||
Usage | ||
--- | ||
## Usage | ||
@@ -76,4 +80,3 @@ Suppose you have the following Express route: | ||
Design Decisions | ||
--- | ||
## Design Decisions | ||
@@ -86,4 +89,3 @@ We wanted some simple mocks without a large framework. | ||
For Developers | ||
--- | ||
## For Developers | ||
@@ -98,25 +100,7 @@ We are looking for more volunteers to bring value to this project, | ||
After making any changes, please verify your works. | ||
If you wish to contribute please read our [Contributing Guidelines](CONTRIBUTING.md). | ||
Running Tests | ||
--- | ||
Install `jshint` globally. | ||
## Release Notes | ||
```bash | ||
npm install -g jshint | ||
``` | ||
Navigate to the project folder and run `npm install` to install the | ||
project's dependencies. | ||
Then simply run the tests. | ||
```bash | ||
./run-tests | ||
``` | ||
Release Notes | ||
--- | ||
Most releases fix bugs with our mocks or add features similar to the | ||
@@ -126,8 +110,7 @@ actual `Request` and `Response` objects offered by Node.js and extended | ||
* v 1.2.5 | ||
[Most Recent Releast Notes](https://github.com/howardabrams/node-mocks-http/releases) | ||
* Add `path` to request similar to how express does `req.path` | ||
* Emit send and end events for json response too | ||
* res.set() works like Express when passed an object #33 | ||
* Set mockResponse.statusCode default to 200, instead of -1 | ||
* [v1.2.7](https://github.com/howardabrams/node-mocks-http/releases/tag/v1.2.7) - March 24, 2015 | ||
* [v1.2.6](https://github.com/howardabrams/node-mocks-http/releases/tag/v1.2.6) - March 19, 2015 | ||
* [v1.2.5](https://github.com/howardabrams/node-mocks-http/releases/tag/v1.2.5) - March 5, 2015 | ||
@@ -134,0 +117,0 @@ |
@@ -0,1 +1,3 @@ | ||
'use strict'; | ||
/** | ||
@@ -50,2 +52,32 @@ * Test: test-mockRequest | ||
exports['originalUrl - Default value'] = function(test) { | ||
var request = httpMocks.createRequest(); | ||
test.equal(request.url, request.originalUrl); | ||
test.done(); | ||
}; | ||
exports['originalUrl - Default value (with url option set)'] = function(test) { | ||
var expected = 'http://localhost:5732/blah'; | ||
var request = httpMocks.createRequest({ url: expected }); | ||
test.equal(request.url, request.originalUrl); | ||
test.done(); | ||
}; | ||
exports['originalUrl - Default value (with url and originalUrl options set)'] = function(test) { | ||
var url = 'http://localhost:5732/blah'; | ||
var originalUrl = 'http://original/blah'; | ||
var request = httpMocks.createRequest({ url: url, originalUrl: originalUrl }); | ||
test.equal(url, request.url); | ||
test.equal(originalUrl, request.originalUrl); | ||
test.done(); | ||
}; | ||
exports['originalUrl - Setting using ._setOriginalUrl()'] = function(test) { | ||
var request = httpMocks.createRequest(); | ||
var expected = 'http://localhost:5732/blah'; | ||
request._setOriginalUrl(expected); | ||
test.equal(expected, request.originalUrl); | ||
test.done(); | ||
}; | ||
exports['get/header - Setting a header using options'] = function(test) { | ||
@@ -138,3 +170,3 @@ var name = 'accept'; | ||
var urlValue = 'http://localhost:6522/blingbling'; | ||
var usernameValue = "mittens"; | ||
var usernameValue = 'mittens'; | ||
@@ -166,2 +198,3 @@ var request = httpMocks.createRequest({ | ||
test.equal(request.url, urlValue); | ||
test.equal(request.originalUrl, urlValue); | ||
test.equal(request.params.id, idValue); | ||
@@ -248,5 +281,5 @@ test.deepEqual(request.session, {}); | ||
test.equal(request.query['a'], '1'); | ||
test.equal(request.query['b'], '2'); | ||
test.equal(request.query['c'], '3'); | ||
test.equal(request.query.a, '1'); | ||
test.equal(request.query.b, '2'); | ||
test.equal(request.query.c, '3'); | ||
@@ -266,5 +299,5 @@ test.done(); | ||
test.equal(request.query['a'], '7'); | ||
test.equal(request.query['b'], '8'); | ||
test.equal(request.query['c'], '9'); | ||
test.equal(request.query.a, '7'); | ||
test.equal(request.query.b, '8'); | ||
test.equal(request.query.c, '9'); | ||
@@ -271,0 +304,0 @@ test.done(); |
@@ -0,1 +1,3 @@ | ||
'use strict'; | ||
/** | ||
@@ -11,349 +13,349 @@ * Test: test-mockResponse | ||
exports['object - Simple verification'] = function (test) { | ||
var response = httpMocks.createResponse(); | ||
var response = httpMocks.createResponse(); | ||
response.send("Hello", 'utf8'); | ||
response.send("World"); | ||
test.equal("HelloWorld", response._getData()); | ||
test.ok(response._isUTF8()); | ||
test.ok(!response._isEndCalled()); | ||
test.done(); | ||
response.send('Hello', 'utf8'); | ||
response.send('World'); | ||
test.equal('HelloWorld', response._getData()); | ||
test.ok(response._isUTF8()); | ||
test.ok(!response._isEndCalled()); | ||
test.done(); | ||
}; | ||
exports['object - Data Initialization'] = function (test) { | ||
var response = httpMocks.createResponse(); | ||
test.equal(200, response.statusCode); | ||
test.equal("", response._getData()); | ||
test.ok(!response._isUTF8()); | ||
test.ok(!response._isEndCalled()); | ||
test.done(); | ||
var response = httpMocks.createResponse(); | ||
test.equal(200, response.statusCode); | ||
test.equal('', response._getData()); | ||
test.ok(!response._isUTF8()); | ||
test.ok(!response._isEndCalled()); | ||
test.done(); | ||
}; | ||
exports['end - Simple Verification'] = function (test) { | ||
var response = httpMocks.createResponse(); | ||
var response = httpMocks.createResponse(); | ||
response.send("Hello"); | ||
response.end("World"); | ||
response.send('Hello'); | ||
response.end('World'); | ||
test.equal("HelloWorld", response._getData()); | ||
test.equal('HelloWorld', response._getData()); | ||
test.ok(response._isEndCalled()); | ||
test.done(); | ||
test.ok(response._isEndCalled()); | ||
test.done(); | ||
}; | ||
exports['end - No Data Called'] = function (test) { | ||
var response = httpMocks.createResponse(); | ||
var response = httpMocks.createResponse(); | ||
response.end("Hello World"); | ||
response.end('Hello World'); | ||
test.equal("Hello World", response._getData()); | ||
test.equal('Hello World', response._getData()); | ||
test.ok(response._isEndCalled()); | ||
test.done(); | ||
test.ok(response._isEndCalled()); | ||
test.done(); | ||
}; | ||
exports['write - Simple verification'] = function (test) { | ||
var response = httpMocks.createResponse(); | ||
var response = httpMocks.createResponse(); | ||
response.write("Hello", 'utf8'); | ||
response.end("World"); | ||
response.write('Hello', 'utf8'); | ||
response.end('World'); | ||
test.equal("HelloWorld", response._getData()); | ||
test.equal('HelloWorld', response._getData()); | ||
test.ok(response._isUTF8()); | ||
test.ok(response._isEndCalled()); | ||
test.done(); | ||
test.ok(response._isUTF8()); | ||
test.ok(response._isEndCalled()); | ||
test.done(); | ||
}; | ||
exports['setHeader - Simple verification'] = function (test) { | ||
var response = httpMocks.createResponse(); | ||
var response = httpMocks.createResponse(); | ||
response.setHeader('foo', 'bar'); | ||
response.setHeader('bling', 'blang'); | ||
response.setHeader('foo', 'bar'); | ||
response.setHeader('bling', 'blang'); | ||
test.equal('bar', response.getHeader('foo')); | ||
test.equal('blang', response.getHeader('bling')); | ||
test.equal('bar', response.getHeader('foo')); | ||
test.equal('blang', response.getHeader('bling')); | ||
response.removeHeader('bling'); | ||
test.ok(!response.getHeader('bling')); | ||
response.removeHeader('bling'); | ||
test.ok(!response.getHeader('bling')); | ||
test.done(); | ||
test.done(); | ||
}; | ||
exports['setHeader - Can not call after end'] = function (test) { | ||
var response = httpMocks.createResponse(); | ||
var response = httpMocks.createResponse(); | ||
var body = 'hello world'; | ||
response.end(body); | ||
var body = 'hello world'; | ||
response.end(body); | ||
test.throws(function () { | ||
response.setHead('Content-Length', body.length); | ||
}); | ||
test.done(); | ||
test.throws(function () { | ||
response.setHead('Content-Length', body.length); | ||
}); | ||
test.done(); | ||
}; | ||
exports['set - Can set multiple headers with object'] = function (test) { | ||
var response = httpMocks.createResponse(); | ||
var response = httpMocks.createResponse(); | ||
response.set({ | ||
'foo': 'bar', | ||
'bling': 'blang' | ||
}); | ||
response.set({ | ||
'foo': 'bar', | ||
'bling': 'blang' | ||
}); | ||
test.equal('bar', response.getHeader('foo')); | ||
test.equal('blang', response.getHeader('bling')); | ||
test.equal('bar', response.getHeader('foo')); | ||
test.equal('blang', response.getHeader('bling')); | ||
test.done(); | ||
test.done(); | ||
}; | ||
exports['writeHead - Simple verification'] = function (test) { | ||
var response = httpMocks.createResponse(); | ||
var response = httpMocks.createResponse(); | ||
var body = 'hello world'; | ||
response.writeHead(200, { | ||
'Content-Length': body.length, | ||
'Content-Type': 'text/plain' | ||
}); | ||
response.end(body); | ||
var body = 'hello world'; | ||
response.writeHead(200, { | ||
'Content-Length': body.length, | ||
'Content-Type': 'text/plain' | ||
}); | ||
response.end(body); | ||
test.equal(200, response._getStatusCode()); | ||
test.equal(body, response._getData()); | ||
test.ok(response._isDataLengthValid()); | ||
test.ok(response._isEndCalled()); | ||
test.ok(!response._isJSON()); | ||
test.done(); | ||
test.equal(200, response._getStatusCode()); | ||
test.equal(body, response._getData()); | ||
test.ok(response._isDataLengthValid()); | ||
test.ok(response._isEndCalled()); | ||
test.ok(!response._isJSON()); | ||
test.done(); | ||
}; | ||
exports['writeHead - Can not call after end'] = function (test) { | ||
var response = httpMocks.createResponse(); | ||
var response = httpMocks.createResponse(); | ||
var body = 'hello world'; | ||
response.end(body); | ||
var body = 'hello world'; | ||
response.end(body); | ||
test.throws(function () { | ||
response.writeHead(200, { | ||
'Content-Length': body.length, | ||
'Content-Type': 'text/plain' | ||
test.throws(function () { | ||
response.writeHead(200, { | ||
'Content-Length': body.length, | ||
'Content-Type': 'text/plain' | ||
}); | ||
}); | ||
}); | ||
test.done(); | ||
test.done(); | ||
}; | ||
exports['status - Set the status code'] = function (test) { | ||
var response = httpMocks.createResponse(); | ||
response.status(401); | ||
test.equal(401, response._getStatusCode()); | ||
test.done(); | ||
var response = httpMocks.createResponse(); | ||
response.status(401); | ||
test.equal(401, response._getStatusCode()); | ||
test.done(); | ||
}; | ||
exports['send - Status code at the beginning'] = function (test) { | ||
var s = 123; | ||
var t = 'This is a weird status code'; | ||
var s = 123; | ||
var t = 'This is a weird status code'; | ||
var response = httpMocks.createResponse(); | ||
response.send(s, t); | ||
var response = httpMocks.createResponse(); | ||
response.send(s, t); | ||
test.equal(s, response._getStatusCode()); | ||
test.equal(t, response._getData()); | ||
test.done(); | ||
test.equal(s, response._getStatusCode()); | ||
test.equal(t, response._getData()); | ||
test.done(); | ||
}; | ||
exports['send - Status code at the end'] = function (test) { | ||
var s = 543; | ||
var t = 'This is a weird status code'; | ||
var s = 543; | ||
var t = 'This is a weird status code'; | ||
var response = httpMocks.createResponse(); | ||
response.send(t, s); | ||
var response = httpMocks.createResponse(); | ||
response.send(t, s); | ||
test.equal(s, response._getStatusCode()); | ||
test.equal(t, response._getData()); | ||
test.done(); | ||
test.equal(s, response._getStatusCode()); | ||
test.equal(t, response._getData()); | ||
test.done(); | ||
}; | ||
exports['implement - WriteableStream'] = function (test) { | ||
var response = httpMocks.createResponse(); | ||
test.equal(typeof (response.writable), 'function'); | ||
test.equal(typeof (response.destroy), 'function'); | ||
test.equal(typeof (response.destroySoon), 'function'); | ||
test.done(); | ||
var response = httpMocks.createResponse(); | ||
test.equal(typeof (response.writable), 'function'); | ||
test.equal(typeof (response.destroy), 'function'); | ||
test.equal(typeof (response.destroySoon), 'function'); | ||
test.done(); | ||
}; | ||
exports['implement - EventEmitter'] = function (test) { | ||
var response = httpMocks.createResponse(); | ||
test.equal(typeof (response.addListener), 'function'); | ||
test.equal(typeof (response.on), 'function'); | ||
test.equal(typeof (response.once), 'function'); | ||
test.equal(typeof (response.removeListener), 'function'); | ||
test.equal(typeof (response.removeAllListeners), 'function'); | ||
test.equal(typeof (response.setMaxListeners), 'function'); | ||
test.equal(typeof (response.listeners), 'function'); | ||
test.equal(typeof (response.emit), 'function'); | ||
test.done(); | ||
var response = httpMocks.createResponse(); | ||
test.equal(typeof (response.addListener), 'function'); | ||
test.equal(typeof (response.on), 'function'); | ||
test.equal(typeof (response.once), 'function'); | ||
test.equal(typeof (response.removeListener), 'function'); | ||
test.equal(typeof (response.removeAllListeners), 'function'); | ||
test.equal(typeof (response.setMaxListeners), 'function'); | ||
test.equal(typeof (response.listeners), 'function'); | ||
test.equal(typeof (response.emit), 'function'); | ||
test.done(); | ||
}; | ||
exports['cookies - Cookies creation'] = function (test) { | ||
var response = httpMocks.createResponse(); | ||
test.deepEqual(response.cookies, {}); | ||
test.done(); | ||
var response = httpMocks.createResponse(); | ||
test.deepEqual(response.cookies, {}); | ||
test.done(); | ||
}; | ||
exports['cookies - Cookies assignment'] = function (test) { | ||
var response = httpMocks.createResponse(); | ||
response.cookie("egg", "chicken", { | ||
maxAge: 1000 | ||
}); | ||
test.deepEqual(response.cookies, { | ||
egg: { | ||
value: 'chicken', | ||
options: { | ||
var response = httpMocks.createResponse(); | ||
response.cookie('egg', 'chicken', { | ||
maxAge: 1000 | ||
} | ||
} | ||
}); | ||
test.done(); | ||
}); | ||
test.deepEqual(response.cookies, { | ||
egg: { | ||
value: 'chicken', | ||
options: { | ||
maxAge: 1000 | ||
} | ||
} | ||
}); | ||
test.done(); | ||
}; | ||
exports['cookies - Cookie deletion'] = function (test) { | ||
var response = httpMocks.createResponse(); | ||
response.cookie("egg", "chicken", { | ||
maxAge: 1000 | ||
}); | ||
response.clearCookie("egg"); | ||
test.deepEqual(response.cookies, {}); | ||
test.done(); | ||
var response = httpMocks.createResponse(); | ||
response.cookie('egg', 'chicken', { | ||
maxAge: 1000 | ||
}); | ||
response.clearCookie('egg'); | ||
test.deepEqual(response.cookies, {}); | ||
test.done(); | ||
}; | ||
exports['redirect - Redirect to a url with response code'] = function (test) { | ||
var response = httpMocks.createResponse(); | ||
var url = '/index'; | ||
var responseCode = 200; | ||
response.redirect(responseCode, url); | ||
test.equal(response._getRedirectUrl(), url); | ||
test.equal(response._getStatusCode(), responseCode); | ||
test.done(); | ||
var response = httpMocks.createResponse(); | ||
var url = '/index'; | ||
var responseCode = 200; | ||
response.redirect(responseCode, url); | ||
test.equal(response._getRedirectUrl(), url); | ||
test.equal(response._getStatusCode(), responseCode); | ||
test.done(); | ||
}; | ||
exports['redirect - Redirect to a url without response code'] = function (test) { | ||
var response = httpMocks.createResponse(); | ||
var url = '/index'; | ||
response.redirect(url); | ||
test.equal(response._getRedirectUrl(), url); | ||
test.equal(response._getStatusCode(), 302); | ||
test.done(); | ||
var response = httpMocks.createResponse(); | ||
var url = '/index'; | ||
response.redirect(url); | ||
test.equal(response._getRedirectUrl(), url); | ||
test.equal(response._getStatusCode(), 302); | ||
test.done(); | ||
}; | ||
exports['render - Render to a view with data'] = function (test) { | ||
var response = httpMocks.createResponse(); | ||
var view = 'index'; | ||
var data = { | ||
'name': 'bob' | ||
}; | ||
var callback = function () {}; | ||
response.render(view, data, callback); | ||
test.equal(response._getRenderView(), view); | ||
test.deepEqual(response._getRenderData(), data); | ||
test.done(); | ||
var response = httpMocks.createResponse(); | ||
var view = 'index'; | ||
var data = { | ||
'name': 'bob' | ||
}; | ||
var callback = function () {}; | ||
response.render(view, data, callback); | ||
test.equal(response._getRenderView(), view); | ||
test.deepEqual(response._getRenderData(), data); | ||
test.done(); | ||
}; | ||
exports['render - Render to a view without data'] = function (test) { | ||
var response = httpMocks.createResponse(); | ||
var view = 'index'; | ||
var callback = function () {}; | ||
response.render(view, callback); | ||
test.equal(response._getRenderView(), view); | ||
test.done(); | ||
var response = httpMocks.createResponse(); | ||
var view = 'index'; | ||
var callback = function () {}; | ||
response.render(view, callback); | ||
test.equal(response._getRenderView(), view); | ||
test.done(); | ||
}; | ||
exports['json - Without status code'] = function (test) { | ||
var response = httpMocks.createResponse(), | ||
data = { | ||
hello: 'there' | ||
}; | ||
var response = httpMocks.createResponse(), | ||
data = { | ||
hello: 'there' | ||
}; | ||
response.json(data); | ||
test.equal(response._isJSON(), true); | ||
test.equal(response._getData(), JSON.stringify(data)); | ||
test.equal(response.statusCode, 200); | ||
test.done(); | ||
response.json(data); | ||
test.equal(response._isJSON(), true); | ||
test.equal(response._getData(), JSON.stringify(data)); | ||
test.equal(response.statusCode, 200); | ||
test.done(); | ||
}; | ||
exports['json - With status code'] = function (test) { | ||
var response = httpMocks.createResponse(), | ||
data = { | ||
hello: 'there' | ||
}; | ||
response.json(201, data); | ||
test.equal(response._isJSON(), true); | ||
test.equal(response._getData(), JSON.stringify(data)); | ||
test.equal(response.statusCode, 201); | ||
test.done(); | ||
var response = httpMocks.createResponse(), | ||
data = { | ||
hello: 'there' | ||
}; | ||
response.json(201, data); | ||
test.equal(response._isJSON(), true); | ||
test.equal(response._getData(), JSON.stringify(data)); | ||
test.equal(response.statusCode, 201); | ||
test.done(); | ||
}; | ||
exports['json - With status code reverse'] = function (test) { | ||
var response = httpMocks.createResponse(), | ||
data = { | ||
hello: 'there' | ||
}; | ||
var response = httpMocks.createResponse(), | ||
data = { | ||
hello: 'there' | ||
}; | ||
response.json(data, 201); | ||
test.equal(response._isJSON(), true); | ||
test.equal(response._getData(), JSON.stringify(data)); | ||
test.equal(response.statusCode, 201); | ||
test.done(); | ||
response.json(data, 201); | ||
test.equal(response._isJSON(), true); | ||
test.equal(response._getData(), JSON.stringify(data)); | ||
test.equal(response.statusCode, 201); | ||
test.done(); | ||
}; | ||
exports['json - With status code - chain'] = function (test) { | ||
var response = httpMocks.createResponse(), | ||
data = { | ||
hello: 'there' | ||
}; | ||
response.status(201).json(data); | ||
test.equal(response._isJSON(), true); | ||
test.equal(response._getData(), JSON.stringify(data)); | ||
test.equal(response.statusCode, 201); | ||
test.done(); | ||
var response = httpMocks.createResponse(), | ||
data = { | ||
hello: 'there' | ||
}; | ||
response.status(201).json(data); | ||
test.equal(response._isJSON(), true); | ||
test.equal(response._getData(), JSON.stringify(data)); | ||
test.equal(response.statusCode, 201); | ||
test.done(); | ||
}; | ||
exports['events - end'] = function (test) { | ||
var response = httpMocks.createResponse({ | ||
eventEmitter: EventEmitter | ||
}); | ||
var response = httpMocks.createResponse({ | ||
eventEmitter: EventEmitter | ||
}); | ||
response.on('end', function () { | ||
test.ok(response._isEndCalled()); | ||
test.done(); | ||
}); | ||
response.on('end', function () { | ||
test.ok(response._isEndCalled()); | ||
test.done(); | ||
}); | ||
response.end(); | ||
response.end(); | ||
}; | ||
exports['events - send'] = function (test) { | ||
var response = httpMocks.createResponse({ | ||
eventEmitter: EventEmitter | ||
}); | ||
var response = httpMocks.createResponse({ | ||
eventEmitter: EventEmitter | ||
}); | ||
response.on('send', function () { | ||
test.equal(response.statusCode, 200); | ||
test.done(); | ||
}); | ||
response.on('send', function () { | ||
test.equal(response.statusCode, 200); | ||
test.done(); | ||
}); | ||
response.send(200); | ||
response.send(200); | ||
}; | ||
exports['events - render'] = function (test) { | ||
var response = httpMocks.createResponse({ | ||
eventEmitter: EventEmitter | ||
}); | ||
var view = 'index'; | ||
var data = { | ||
'name': 'bob' | ||
}; | ||
var callback = function () {}; | ||
var response = httpMocks.createResponse({ | ||
eventEmitter: EventEmitter | ||
}); | ||
var view = 'index'; | ||
var data = { | ||
'name': 'bob' | ||
}; | ||
var callback = function () {}; | ||
response.on('render', function () { | ||
test.equal(response._getRenderView(), view); | ||
test.deepEqual(response._getRenderData(), data); | ||
test.done(); | ||
}); | ||
response.on('render', function () { | ||
test.equal(response._getRenderView(), view); | ||
test.deepEqual(response._getRenderData(), data); | ||
test.done(); | ||
}); | ||
response.render(view, data, callback); | ||
response.render(view, data, callback); | ||
}; | ||
@@ -363,21 +365,70 @@ | ||
exports['send - sending response objects a.k.a restifyError with statusCode'] = function(test) { | ||
var errors = require('node-restify-errors') | ||
var response = httpMocks.createResponse(); | ||
response.send(409, new errors.InvalidArgumentError("I just dont like you")); | ||
var errors = require('node-restify-errors'); | ||
var response = httpMocks.createResponse(); | ||
response.send(409, new errors.InvalidArgumentError('I just dont like you')); | ||
test.equal(409, response._getStatusCode()); | ||
test.equal('InvalidArgument', response._getData().code); | ||
test.equal('I just dont like you', response._getData().message); | ||
test.done(); | ||
test.equal(409, response._getStatusCode()); | ||
test.equal('InvalidArgument', response._getData().code); | ||
test.equal('I just dont like you', response._getData().message); | ||
test.done(); | ||
}; | ||
exports['send - sending response objects a.k.a restifyError without statusCode'] = function(test) { | ||
var errors = require('node-restify-errors') | ||
var response = httpMocks.createResponse(); | ||
response.send(new errors.InvalidArgumentError("I just dont like you")); | ||
var errors = require('node-restify-errors'); | ||
var response = httpMocks.createResponse(); | ||
response.send(new errors.InvalidArgumentError('I just dont like you')); | ||
test.equal(409, response._getStatusCode()); | ||
test.equal('InvalidArgument', response._getData().code); | ||
test.equal('I just dont like you', response._getData().message); | ||
test.done(); | ||
test.equal(409, response._getStatusCode()); | ||
test.equal('InvalidArgument', response._getData().code); | ||
test.equal('I just dont like you', response._getData().message); | ||
test.done(); | ||
}; | ||
exports['type - set "Content-Type" response header with .type()'] = function(test) { | ||
var response = httpMocks.createResponse(); | ||
response.type('.html'); | ||
test.equal('text/html', response.getHeader('Content-Type')); | ||
response.type('html'); | ||
test.equal('text/html', response.getHeader('Content-Type')); | ||
response.type('json'); | ||
test.equal('application/json', response.getHeader('Content-Type')); | ||
response.type('application/json'); | ||
test.equal('application/json', response.getHeader('Content-Type')); | ||
response.type('png'); | ||
test.equal('image/png', response.getHeader('Content-Type')); | ||
test.done(); | ||
}; | ||
exports['type - set "Content-Type" response header with .contentType()'] = function(test) { | ||
var response = httpMocks.createResponse(); | ||
response.contentType('.html'); | ||
test.equal('text/html', response.getHeader('Content-Type')); | ||
response.contentType('html'); | ||
test.equal('text/html', response.getHeader('Content-Type')); | ||
response.contentType('json'); | ||
test.equal('application/json', response.getHeader('Content-Type')); | ||
response.contentType('application/json'); | ||
test.equal('application/json', response.getHeader('Content-Type')); | ||
response.contentType('png'); | ||
test.equal('image/png', response.getHeader('Content-Type')); | ||
test.done(); | ||
}; | ||
exports['send - response "Status Code" with .sendStatus()'] = function(test) { | ||
var http = require('../lib/node/http'); | ||
var response, statusCode; | ||
for (statusCode in http.STATUS_CODES) { | ||
response = httpMocks.createResponse(); | ||
response.sendStatus(statusCode); | ||
test.equal(response._getStatusCode(), statusCode); | ||
test.equal(response._getData(), http.STATUS_CODES[statusCode]); | ||
// uncomment after fixing bug in .send() | ||
// test.ok(response._isEndCalled()); | ||
response = null; | ||
} | ||
test.done(); | ||
}; |
Sorry, the diff of this file is not supported yet
No bug tracker
MaintenancePackage does not have a linked bug tracker in package.json.
Found 1 instance in 1 package
79024
22
1582
0
1
3
115
+ Addedmime@^1.3.4
+ Addedmime@1.6.0(transitive)