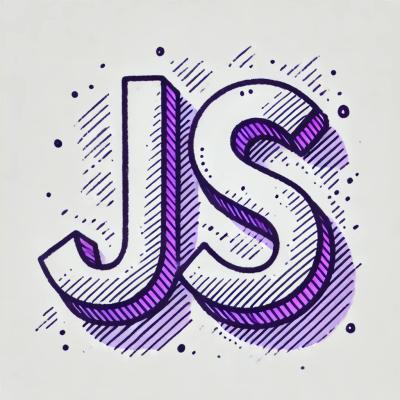
Security News
JavaScript Leaders Demand Oracle Release the JavaScript Trademark
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
node-pre-gyp
Advanced tools
The node-pre-gyp package is a tool that allows developers to publish and install Node.js native add-ons from binaries. This eliminates the need for developers to compile their native add-ons from source during installation, simplifying the deployment process and reducing setup time.
Publishing binaries
This command allows developers to publish pre-compiled binary files to a hosting service, making them available for installation. This is useful for distributing Node.js native add-ons without requiring users to compile the code themselves.
node-pre-gyp publish
Installing binaries
This command facilitates the installation of pre-compiled binaries from a remote source. It checks for compatible binaries and downloads them, which speeds up the installation process by avoiding the need for compilation.
node-pre-gyp install
Rebuilding binaries
This command is used to rebuild the native add-on binaries from source. It is useful when pre-compiled binaries are not available or when custom modifications to the binary are needed.
node-pre-gyp rebuild
node-gyp is a cross-platform command-line tool written in Node.js for compiling native addon modules for Node.js. It provides a similar functionality to node-pre-gyp but requires compilation from source, unlike node-pre-gyp which can download pre-compiled binaries.
prebuild is a tool that helps in prebuilding native modules for Node.js. Similar to node-pre-gyp, it supports the installation of pre-compiled binaries. However, prebuild often works in conjunction with prebuild-install and offers a slightly different workflow for managing binary deployments.
prebuildify focuses on creating local prebuilds for native Node.js modules. Unlike node-pre-gyp, which can download binaries from a remote location, prebuildify is designed for bundling the binaries directly with the module, which can be useful for applications that need to work offline or have restricted network access.
node-pre-gyp
is a Node.js native add-on install tool.
No: it plays nicely with them.
binding.gyp
to compile your module with node-gyp
What node-pre-gyp
does is stand between npm
and node-gyp
.
Developers of C++ modules can use node-pre-gyp
to package and publish the binary .node
before running npm publish
.
Users can then npm install
your module from a binary and node-pre-gyp
does the work to make this seamless.
node-pre-gyp
:For more examples see the test apps.
1) Add a custom install
script to package.json
"scripts": {
"install": "node-pre-gyp install --fallback-to-build",
}
2) Add a binary
property to package.json
It must provide these properties:
module_name
: The name of your native node module.module_path
: The location your native module is placed after a build. This should be an empty directory without other javascript files.remote_uri
: A url to the remote location where you've published tarball binariestemplate
: A string describing the tarball versioning scheme for your binariesAnd example from node-sqlite3
looks like:
"binary": {
"module_name": "node_sqlite3",
"module_path": "./lib/binding/",
"remote_uri": "http://node-sqlite3.s3.amazonaws.com",
"template": "{configuration}/{module_name}-v{version}-{node_abi}-{platform}-{arch}.tar.gz"
},
3) Build and package your app
node-pre-gyp build package
4) Publish the tarball
node-pre-gyp publish
Currently the publish
command pushes your binary to S3. This requires:
remote-uri
points to an S3 http or https endpoint.You can also host your binaries elsewhere. To do this requires:
package
command.build/stage/
directory.remote_uri
value to it.5) Automating builds
Now you need to publish builds for all the platforms and node versions you wish to support. This is best automated. See Travis Automation for how to auto-publish builds on OS X and Linux. On windows consider using a script like this to quickly create and publish binaries.
6) You're done!
Now publish your package to the npm registry. Users will now be able to install your module from a binary.
What will happen is this:
npm install <your package>
will pull from the npm registryinstall
script which will call out to node-pre-gyp
node-pre-gyp
will fetch the binary .node
module and unpack in the right placeIf a failure occurred and --fallback-to-build
was used then node-gyp rebuild
will be called to try to source compile the module.
You can host wherever you choose but S3 is cheap, node-pre-gyp publish
expects it, and S3 can be integrated well with travis.ci to automate builds for OS X and Ubuntu. Here is an approach to do this:
First, get setup locally and test the workflow:
1) Create an S3 bucket
And have your key and secret key ready for writing to the bucket.
2) Install node-pre-gyp
Either install it globally:
npm install node-pre-gyp -g
Or put the local version on your PATH
export PATH=`pwd`/node_modules/.bin/:$PATH
3) Create an ~/.node_pre_gyprc
Or pass options in any way supported by RC
A ~/.node_pre_gyprc
looks like:
{
"accessKeyId": "xxx",
"secretAccessKey": "xxx"
}
Another way is to use your environment:
export node_pre_gyp_accessKeyId=xxx
export node_pre_gyp_secretAccessKey=xxx
You may also need to specify the region
if it is not explicit in the remote_uri
value you use. The bucket
can also be specified but it is optional because node-pre-gyp
will detect it from the remote_uri
value.
4) Package and publish your build
node-pre-gyp package publish
Note: if you hit an error like Hostname/IP doesn't match certificate's altnames
it may mean that you need to provide the region
option in your config.
Travis can push to S3 after a successful build and supports both:
This enables you to cheaply auto-build and auto-publish binaries for (likely) the majority of your users.
1) Install the travis gem
gem install travis
2) Create secure global
variables
Make sure you run this command from within the directory of your module.
Use travis-encrypt
like:
travis encrypt node_pre_gyp_accessKeyId=${node_pre_gyp_accessKeyId}
travis encrypt node_pre_gyp_secretAccessKey=${node_pre_gyp_secretAccessKey}
Then put those values in your .travis.yml
like:
env:
global:
- secure: F+sEL/v56CzHqmCSSES4pEyC9NeQlkoR0Gs/ZuZxX1ytrj8SKtp3MKqBj7zhIclSdXBz4Ev966Da5ctmcTd410p0b240MV6BVOkLUtkjZJyErMBOkeb8n8yVfSoeMx8RiIhBmIvEn+rlQq+bSFis61/JkE9rxsjkGRZi14hHr4M=
- secure: o2nkUQIiABD139XS6L8pxq3XO5gch27hvm/gOdV+dzNKc/s2KomVPWcOyXNxtJGhtecAkABzaW8KHDDi5QL1kNEFx6BxFVMLO8rjFPsMVaBG9Ks6JiDQkkmrGNcnVdxI/6EKTLHTH5WLsz8+J7caDBzvKbEfTux5EamEhxIWgrI=
More details on travis encryption at http://about.travis-ci.org/docs/user/encryption-keys/.
3) Hook up publishing
Just put node-pre-gyp package publish
in your .travis.yml
after npm install
.
If you want binaries for OS X change your .travis.yml
to use:
language: objective-c
Perhaps keep that change in a different git branch and sync that when you want binaries published.
Note: using language: objective-c
instead of language: nodejs
looses node.js specific travis sugar like a matrix for multiple node.js versions.
But you can replicate the lost behavior by replacing:
node_js:
- "0.8"
- "0.10"
With:
env:
matrix:
- export NODE_VERSION="0.8"
- export NODE_VERSION="0.10"
before_install:
- git clone https://github.com/creationix/nvm.git ./.nvm
- source ./.nvm/nvm.sh
- nvm install $NODE_VERSION
- nvm use $NODE_VERSION
4) Publish when you want
You might wish to publish binaries only on a specific commit. To do this you could borrow from the travis.ci idea of commit keywords and add special handling for commit messages with [publish]
:
COMMIT_MESSAGE=$(git show -s --format=%B $TRAVIS_COMMIT | tr -d '\n')
if test "${COMMIT_MESSAGE#*[publish]}" != "$COMMIT_MESSAGE"; then node-pre-gyp publish; fi;
Or you could automatically detect if the git branch is a tag:
if [[ $TRAVIS_BRANCH == `git describe --tags --always HEAD` ]] ; then node-pre-gyp publish; fi
Remember this publishing is not the same as npm publish
. We're just talking about the
binary module here and not your entire npm package. To automate the publishing of your entire package to npm on travis see http://about.travis-ci.org/docs/user/deployment/npm/
View all possible commands:
node-pre-gyp --help
node-pre-gyp unpublish
node-pre-gyp clean
node-pre-gyp reinstall # runs "clean" and "install"
Options include:
--build-from-source
--fallback-to-build
Both of these options can be passed alone or they can provide values. So, in addition to being able to pass --build-from-source
you can also pass --build-from-source=myapp
where myapp
is the name of your module.
For example: npm install --build-from-source=myapp
. This is useful if:
myapp
is referenced in the package.json of a larger app and therefore myapp
is being installed as a dependent with npm install
.node-pre-gyp
myapp
and the other modules.FAQs
Node.js native addon binary install tool
The npm package node-pre-gyp receives a total of 657,312 weekly downloads. As such, node-pre-gyp popularity was classified as popular.
We found that node-pre-gyp demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 8 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.