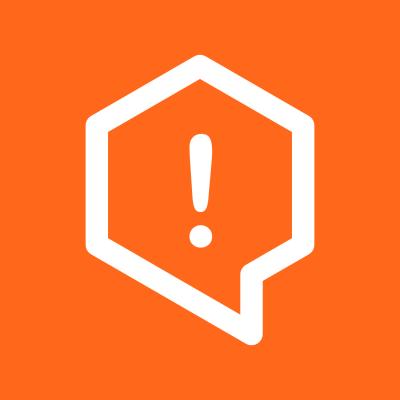
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
node-red-contrib-chromadb
Advanced tools
Chroma is the open-source embedding database. Chroma makes it easy to build LLM apps by making knowledge, facts, and skills pluggable for LLMs.
cd ~/.node-red
npm install node-red-contrib-chromadb
Restart your Node-RED instance.
▸ createCollection(params
): Promise
<Collection
>
Creates a new collection with the specified properties.
Throws
If there is an issue creating the collection.
Example
msg.collection = "my_collection";
msg.payload = {
metadata: {
description: "My first collection"
}
};
return msg;
Name | Type | Description |
---|---|---|
params | Object | The parameters for creating a new collection. |
params.embeddingFunction? | IEmbeddingFunction | Optional custom embedding function for the collection. |
params.metadata? | CollectionMetadata | Optional metadata associated with the collection. |
params.name | string | The name of the collection. |
Promise
<Collection
>
A promise that resolves to the created collection.
▸ deleteCollection(params
): Promise
<void
>
Deletes a collection with the specified name.
Throws
If there is an issue deleting the collection.
Example
msg.collection = "my_collection";
msg.payload = {};
return msg;
Name | Type | Description |
---|---|---|
params | Object | The parameters for deleting a collection. |
params.name | string | The name of the collection. |
Promise
<void
>
A promise that resolves when the collection is deleted.
▸ getCollection(params
): Promise
<Collection
>
Gets a collection with the specified name.
Throws
If there is an issue getting the collection.
Example
msg.collection = "my_collection";
msg.payload = {};
return msg;
Name | Type | Description |
---|---|---|
params | Object | The parameters for getting a collection. |
params.embeddingFunction? | IEmbeddingFunction | Optional custom embedding function for the collection. |
params.name | string | The name of the collection. |
Promise
<Collection
>
A promise that resolves to the collection.
▸ getOrCreateCollection(params
): Promise
<Collection
>
Gets or creates a collection with the specified properties.
Throws
If there is an issue getting or creating the collection.
Example
msg.collection = "my_collection";
msg.payload = {
metadata: {
description: "My first collection"
}
};
return msg;
Name | Type | Description |
---|---|---|
params | Object | The parameters for creating a new collection. |
params.embeddingFunction? | IEmbeddingFunction | Optional custom embedding function for the collection. |
params.metadata? | CollectionMetadata | Optional metadata associated with the collection. |
params.name | string | The name of the collection. |
Promise
<Collection
>
A promise that resolves to the got or created collection.
▸ heartbeat(): Promise
<number
>
Returns a heartbeat from the Chroma API.
Promise
<number
>
A promise that resolves to the heartbeat from the Chroma API.
▸ listCollections(): Promise
<CollectionType
[]>
Lists all collections.
Throws
If there is an issue listing the collections.
Promise
<CollectionType
[]>
A promise that resolves to a list of collection names.
▸ reset(): Promise
<Reset200Response
>
Resets the state of the object by making an API call to the reset endpoint.
Throws
If there is an issue resetting the state.
Promise
<Reset200Response
>
A promise that resolves when the reset operation is complete.
▸ version(): Promise
<string
>
Returns the version of the Chroma API.
Promise
<string
>
A promise that resolves to the version of the Chroma API.
▸ add(params
): Promise
<AddResponse
>
Add items to the collection
Example
msg.collection = "my_collection";
msg.payload = {
ids: ["id1", "id2"],
embeddings: [
[1, 2, 3],
[4, 5, 6],
],
metadatas: [{ key: "value" }, { key: "value" }],
documents: ["document1", "document2"],
};
return msg;
Name | Type | Description |
---|---|---|
params | Object | The parameters for the query. |
params.documents? | string | Documents | Optional documents of the items to add. |
params.embeddings? | Embedding | Embeddings | Optional embeddings of the items to add. |
params.ids | string | IDs | IDs of the items to add. |
params.metadatas? | Metadata | Metadatas | Optional metadata of the items to add. |
Promise
<AddResponse
>
▸ count(): Promise
<number
>
Count the number of items in the collection
Example
msg.collection = "my_collection";
msg.payload = {};
return msg;
Promise
<number
>
▸ delete(params?
): Promise
<string
[]>
Deletes items from the collection.
Throws
If there is an issue deleting items from the collection.
Example
msg.collection = "my_collection";
msg.payload = {
ids: "some_id",
where: { key: "value" },
whereDocument: { "$contains": "search_string" }
};
return msg;
Name | Type | Description |
---|---|---|
params | Object | The parameters for deleting items from the collection. |
params.ids? | string | IDs | Optional ID or array of IDs of items to delete. |
params.where? | Where | Optional query condition to filter items to delete based on metadata values. |
params.whereDocument? | WhereDocument | Optional query condition to filter items to delete based on document content. |
Promise
<string
[]>
A promise that resolves to the IDs of the deleted items.
▸ get(params?
): Promise
<GetResponse
>
Get items from the collection
Example
msg.collection = "my_collection";
msg.payload = {
ids: ["id1", "id2"],
where: { key: "value" },
limit: 10,
offset: 0,
include: ["embeddings", "metadatas", "documents"],
whereDocument: { $contains: "value" },
};
return msg;
Name | Type | Description |
---|---|---|
params | Object | The parameters for the query. |
params.ids? | string | IDs | Optional IDs of the items to get. |
params.include? | IncludeEnum [] | Optional list of items to include in the response. |
params.limit? | number | Optional limit on the number of items to get. |
params.offset? | number | Optional offset on the items to get. |
params.where? | Where | Optional where clause to filter items by. |
params.whereDocument? | WhereDocument | Optional where clause to filter items by. |
Promise
<GetResponse
>
▸ modify(params?
): Promise
<void
>
Modify the collection name or metadata
Example
msg.collection = "my_collection";
msg.payload = {
name: "new collection name",
metadata: { key: "value" },
};
return msg;
Name | Type | Description |
---|---|---|
params | Object | The parameters for the query. |
params.metadata? | CollectionMetadata | Optional new metadata for the collection. |
params.name? | string | Optional new name for the collection. |
Promise
<void
>
▸ peek(params?
): Promise
<GetResponse
>
Peek inside the collection
Throws
If there is an issue executing the query.
Example
msg.collection = "my_collection";
msg.payload = {
limit: 10,
};
return msg;
Name | Type | Description |
---|---|---|
params | Object | The parameters for the query. |
params.limit? | number | Optional number of results to return (default is 10). |
Promise
<GetResponse
>
A promise that resolves to the query results.
▸ query(params
): Promise
<QueryResponse
>
Performs a query on the collection using the specified parameters.
Throws
If there is an issue executing the query.
Example
// Query the collection using embeddings
msg.collection = "my_collection";
msg.payload = {
queryEmbeddings: [0.1, 0.2],
nResults: 10,
where: { key: "value" },
include: ["metadata", "document"]
};
return msg;
Example
// Query the collection using query text
msg.collection = "my_collection";
msg.payload = {
queryTexts: "some text",
nResults: 10,
where: { key: "value" },
include: ["metadata", "document"]
};
return msg;
Name | Type | Description |
---|---|---|
params | Object | The parameters for the query. |
params.include? | IncludeEnum [] | Optional array of fields to include in the result, such as "metadata" and "document". |
params.nResults? | number | Optional number of results to return (default is 10). |
params.queryEmbeddings? | Embedding | Embeddings | Optional query embeddings to use for the search. |
params.queryTexts? | string | string [] | Optional query text(s) to search for in the collection. |
params.where? | Where | Optional query condition to filter results based on metadata values. |
params.whereDocument? | WhereDocument | Optional query condition to filter results based on document content. |
Promise
<QueryResponse
>
A promise that resolves to the query results.
▸ update(params
): Promise
<boolean
>
Update the embeddings, documents, and/or metadatas of existing items
Example
msg.collection = "my_collection";
msg.payload = {
ids: ["id1", "id2"],
embeddings: [
[1, 2, 3],
[4, 5, 6],
],
metadatas: [{ key: "value" }, { key: "value" }],
documents: ["new document 1", "new document 2"],
};
return msg;
Name | Type | Description |
---|---|---|
params | Object | The parameters for the query. |
params.documents? | string | Documents | Optional documents to update. |
params.embeddings? | Embedding | Embeddings | Optional embeddings to update. |
params.ids | string | IDs | The IDs of the items to update. |
params.metadatas? | Metadata | Metadatas | Optional metadatas to update. |
Promise
<boolean
>
▸ upsert(params
): Promise
<boolean
>
Upsert items to the collection
Example
msg.collection = "my_collection";
msg.payload = {
ids: ["id1", "id2"],
embeddings: [
[1, 2, 3],
[4, 5, 6],
],
metadatas: [{ key: "value" }, { key: "value" }],
documents: ["document1", "document2"],
};
return msg;
Name | Type | Description |
---|---|---|
params | Object | The parameters for the query. |
params.documents? | string | Documents | Optional documents of the items to add. |
params.embeddings? | Embedding | Embeddings | Optional embeddings of the items to add. |
params.ids | string | IDs | IDs of the items to add. |
params.metadatas? | Metadata | Metadatas | Optional metadata of the items to add. |
Promise
<boolean
>
Please report any issues or feature requests at GitHub.
FAQs
ChromaDB Node for Node-RED
The npm package node-red-contrib-chromadb receives a total of 5 weekly downloads. As such, node-red-contrib-chromadb popularity was classified as not popular.
We found that node-red-contrib-chromadb demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.