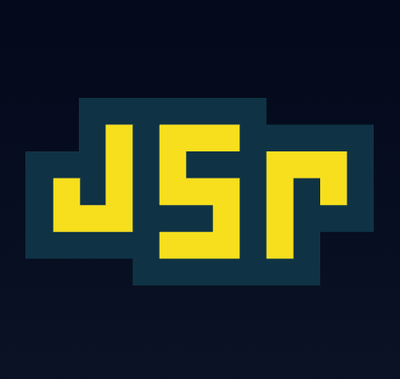
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
node-sass
Advanced tools
The node-sass npm package is a library that allows you to natively compile .scss files to CSS at incredible speed and automatically via a connect middleware. It provides a binding for Node.js to the Sass engine, which is written in C++ and allows for the translation of SCSS or SASS syntax into standard CSS that browsers can understand.
Compiling SCSS to CSS
This feature allows you to compile .scss files into .css files. The 'render' method takes an options object and a callback function. The options object specifies the input and output paths for the SCSS and CSS files, respectively. The callback is invoked after the compilation process, and you can handle the result or error accordingly.
const sass = require('node-sass');
sass.render({
file: 'path/to/input.scss',
outFile: 'path/to/output.css'
}, function(error, result) { // Node-style callback from v3.0.0 onwards
if(!error){
// No errors during the compilation, write this result on the disk
fs.writeFile('path/to/output.css', result.css, function(err){
if(!err){
//file written on disk
}
});
}
});
Watching files or directories
This feature allows you to watch .scss files or directories for changes and automatically recompile them to CSS when a change is detected. The example uses 'chokidar', an external library for watching files, to listen for changes on the specified SCSS file and then uses node-sass to compile the file to CSS.
const sass = require('node-sass');
const chokidar = require('chokidar');
chokidar.watch('path/to/input.scss').on('change', () => {
sass.render({
file: 'path/to/input.scss',
outFile: 'path/to/output.css'
}, function(error, result) {
if (!error) {
fs.writeFile('path/to/output.css', result.css, function(err){
if(!err){
console.log('SCSS file updated.');
}
});
}
});
});
Command Line Interface (CLI) usage
node-sass provides a CLI for compiling SCSS files to CSS directly from the command line. In this example, the '--output-style' option is used to specify the CSS output format (compressed in this case), '-o' is used to define the output directory for the compiled CSS, and the last argument is the input directory containing the SCSS files.
node-sass --output-style compressed -o dist/css src/scss
The 'sass' package is the primary implementation of Sass, which is written in Dart. It provides the same core functionality as node-sass but does not rely on a native C++ binding, making it more portable and easier to install across different environments. It is also the package recommended by the Sass team as node-sass has been deprecated.
PostCSS is a tool for transforming CSS with JavaScript plugins. While it is not a direct replacement for node-sass, it can be used with plugins like 'precss' to provide similar SCSS-like syntax and features. PostCSS is highly extensible and can be integrated with a large number of plugins to extend its capabilities beyond what node-sass offers.
Less is a backward-compatible language extension for CSS. It is similar to SCSS in terms of features like variables, mixins, and nesting, but it has its own syntax and compiles to CSS using the Less compiler. While it serves a similar purpose to node-sass, it is a different preprocessor language with its own community and ecosystem.
Stylus is a preprocessor that serves as an alternative to SCSS. It offers a flexible syntax with optional colons, semicolons, and braces, and provides powerful features like variable interpolation and functions. Stylus can be seen as a more expressive, but less widely adopted, alternative to node-sass.
##node-sass
Node-sass is a library that provides binding for Node.js to libsass, the C version of the popular stylesheet preprocessor, Sass.
It allows you to natively compile .scss files to css at incredible speed and automatically via a connect middleware.
Find it on npm: https://npmjs.org/package/node-sass
npm install node-sass
var sass = require('node-sass');
sass.render({
file: scss_filename,
success: callback
[, options..]
});
// OR
var css = sass.renderSync({
data: scss_content
[, options..]
});
The API for using node-sass has changed, so that now there is only one variable - an options hash. Some of these options are optional, and in some circumstances some are mandatory.
file
is a String
of the path to an scss
file for libsass to render. One of this or data
options are required, for both render and renderSync.
data
is a String
containing the scss to be rendered by libsass. One of this or file
options are required, for both render and renderSync. It is recommended that you use the includePaths
option in conjunction with this, as otherwise libsass may have trouble finding files imported via the @import
directive.
success
is a Function
to be called upon successful rendering of the scss to css. This option is required but only for the render function. If provided to renderSync it will be ignored.
error
is a Function
to be called upon occurance of an error when rendering the scss to css. This option is optional, and only applies to the render function. If provided to renderSync it will be ignored.
includePaths
is an Array
of path String
s to look for any @import
ed files. It is recommended that you use this option if you are using the data
option and have any @import
directives, as otherwise libsass may not find your depended-on files.
outputStyle
is a String
to determine how the final CSS should be rendered. Its value should be one of 'nested', 'expanded', 'compact', 'compressed'
.
[Important: currently the argument outputStyle
has some problem which may cause the output css becomes nothing because of the libsass, so you should not use it now!]
sourceComments
is a String
to determine what debug information is included in the output file. Its value should be one of 'none', 'normal', 'map'
. The default is 'none'
.
[Important: souceComments
is only supported when using the file
option, and does nothing when using data
flag.]
var sass = require('node-sass');
sass.render({
data: 'body{background:blue; a{color:black;}}',
success: function(css){
console.log(css)
},
error: function(error) {
console.log(error);
},
includePaths: [ 'lib/', 'mod/' ],
outputStyle: 'compressed'
});
// OR
console.log(sass.renderSync({
data: 'body{background:blue; a{color:black;}}'),
outputStyle: 'compressed'
});
file
and data
options are set, node-sass will only attempt to honour the file
directive.Recompile .scss
files automatically for connect and express based http servers
var server = connect.createServer(
sass.middleware({
src: __dirname
, dest: __dirname + '/public'
, debug: true
, outputStyle: 'compressed'
}),
connect.static(__dirname + '/public')
);
Heavily inspired by https://github.com/LearnBoost/stylus
@sindresorhus has created a set of grunt tasks based on node-sass: https://github.com/sindresorhus/grunt-sass
There is also an example connect app here: https://github.com/andrew/node-sass-example
Node-sass includes pre-compiled binaries for popular platforms, to add a binary for your platform follow these steps:
Check out the project:
git clone https://github.com/andrew/node-sass.git
cd node-sass
npm install
npm install -g node-gyp
git submodule init
git submodule update
node-gyp rebuild
Replace the prebuild binary with your newly generated one
cp build/Release/binding.node precompiled/*your-platform*/binding.node
The interface for command-line usage is fairly simplistic at this stage, as seen in the following usage section.
Output will be saved with the same name as input SASS file into the current working directory if it's omitted.
node-sass [options] <input.scss> [<output.css>]
Options:
--output-style CSS output style (nested|expanded|compact|compressed) [default: "nested"]
--source-comments Include debug info in output (none|normal|map) [default: "none"]
--include-path Path to look for @import-ed files [default: cwd]
--help, -h Print usage info
Special thanks to the following people for submitting patches:
Dean Mao Brett Wilkins litek gonghao
Copyright (c) 2013 Andrew Nesbitt. See LICENSE for details.
FAQs
Wrapper around libsass
The npm package node-sass receives a total of 843,154 weekly downloads. As such, node-sass popularity was classified as popular.
We found that node-sass demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.