node-skia-canvas
Advanced tools
Comparing version 0.1.0 to 0.1.1
const { Canvas: NativeCanvas } = require('bindings')('node-skia') | ||
const CanvasRenderingContext2D = require('./CanvasRenderingContext2D') | ||
const { createProxyToNative } = require('./utils') | ||
@@ -10,2 +9,18 @@ class Canvas { | ||
get width () { | ||
return this.nativeCanvas.width | ||
} | ||
set width (val) { | ||
this.nativeCanvas.width = val | ||
} | ||
get height () { | ||
return this.nativeCanvas.height | ||
} | ||
set height (val) { | ||
this.nativeCanvas.height = val | ||
} | ||
getContext (ctx) { | ||
@@ -18,7 +33,8 @@ if (ctx === '2d') { | ||
} | ||
toBuffer (...args) { | ||
return this.nativeCanvas.toBuffer(...args) | ||
} | ||
} | ||
module.exports = createProxyToNative( | ||
Canvas, | ||
(target) => target.nativeCanvas | ||
) | ||
module.exports = Canvas |
const { Canvas: NativeCanvas, CanvasPattern, CanvasGradient, Image, ImageData } = require('bindings')('node-skia') | ||
const { DOMMatrix } = require('./DOMMatrix') | ||
const parseFont = require('./parseFont') | ||
const { colorVectorToHex, createProxyToNative } = require('./utils') | ||
const { colorVectorToHex } = require('./utils') | ||
@@ -9,3 +9,3 @@ class CanvasRenderingContext2D { | ||
const nativeContext = nativeCanvas.getContext('2d') | ||
return new ProxyCanvasRenderingContext2D(nativeContext) | ||
return new CanvasRenderingContext2D(nativeContext) | ||
} | ||
@@ -20,2 +20,16 @@ | ||
_normalizeRadians (radians) { | ||
radians = radians / Math.PI * 180 | ||
if (Math.abs(radians) > 360) { | ||
radians = radians % 360 | ||
} | ||
if (radians < 0) { | ||
radians += 360 | ||
} | ||
return radians | ||
} | ||
get fillStyle () { | ||
@@ -34,27 +48,50 @@ return this._fillStyle || colorVectorToHex(this.nativeContext.fillStyle) | ||
get strokeStyle () { | ||
return this._strokeStyle || colorVectorToHex(this.nativeContext.strokeStyle) | ||
get font () { | ||
return this.nativeContext.font | ||
} | ||
set strokeStyle (val) { | ||
if (typeof val === 'string') { | ||
val = val.toLowerCase() | ||
} | ||
set font (str) { | ||
this.nativeContext.font = parseFont(str) | ||
} | ||
this._strokeStyle = typeof val === 'string' ? null : val | ||
this.nativeContext.strokeStyle = val | ||
get globalAlpha () { | ||
return this.nativeContext.globalAlpha | ||
} | ||
get shadowColor () { | ||
return colorVectorToHex(this.nativeContext.shadowColor) | ||
set globalAlpha (val) { | ||
this.nativeContext.globalAlpha = val | ||
} | ||
get font () { | ||
return this.nativeContext.font | ||
get imageSmoothingEnabled () { | ||
return this.nativeContext.imageSmoothingEnabled | ||
} | ||
set font (str) { | ||
this.nativeContext.font = parseFont(str) | ||
set imageSmoothingEnabled (val) { | ||
this.nativeContext.imageSmoothingEnabled = val | ||
} | ||
get lineCap () { | ||
return this.nativeContext.lineCap | ||
} | ||
set lineCap (val) { | ||
this.nativeContext.lineCap = val | ||
} | ||
get lineDashOffset () { | ||
return this.nativeContext.lineDashOffset | ||
} | ||
set lineDashOffset (val) { | ||
this.nativeContext.lineDashOffset = val | ||
} | ||
get lineJoin () { | ||
return this.nativeContext.lineJoin | ||
} | ||
set lineJoin (val) { | ||
this.nativeContext.lineJoin = val | ||
} | ||
get lineWidth () { | ||
@@ -77,2 +114,103 @@ return this.nativeContext.lineWidth | ||
get miterLimit () { | ||
return this.nativeContext.miterLimit | ||
} | ||
set miterLimit (val) { | ||
this.nativeContext.miterLimit = val | ||
} | ||
get shadowBlur () { | ||
return this.nativeContext.shadowBlur | ||
} | ||
set shadowBlur (val) { | ||
this.nativeContext.shadowBlur = val | ||
} | ||
get shadowColor () { | ||
return colorVectorToHex(this.nativeContext.shadowColor) | ||
} | ||
set shadowColor (val) { | ||
this.nativeContext.shadowColor = val | ||
} | ||
get shadowOffsetX () { | ||
return this.nativeContext.shadowOffsetX | ||
} | ||
set shadowOffsetX (val) { | ||
this.nativeContext.shadowOffsetX = val | ||
} | ||
get shadowOffsetY () { | ||
return this.nativeContext.shadowOffsetY | ||
} | ||
set shadowOffsetY (val) { | ||
this.nativeContext.shadowOffsetY = val | ||
} | ||
get strokeStyle () { | ||
return this._strokeStyle || colorVectorToHex(this.nativeContext.strokeStyle) | ||
} | ||
set strokeStyle (val) { | ||
if (typeof val === 'string') { | ||
val = val.toLowerCase() | ||
} | ||
this._strokeStyle = typeof val === 'string' ? null : val | ||
this.nativeContext.strokeStyle = val | ||
} | ||
get textAlign () { | ||
return this.nativeContext.textAlign | ||
} | ||
set textAlign (val) { | ||
this.nativeContext.textAlign = val | ||
} | ||
get textBaseline () { | ||
return this.nativeContext.textBaseline | ||
} | ||
set textBaseline (val) { | ||
this.nativeContext.textBaseline = val | ||
} | ||
arc (x, y, radius, startAngle, endAngle) { | ||
return this.nativeContext.arc( | ||
x, y, radius, | ||
this._normalizeRadians(startAngle), | ||
this._normalizeRadians(endAngle) | ||
) | ||
} | ||
arcTo (...args) { | ||
return this.nativeContext.arcTo(...args) | ||
} | ||
beginPath (...args) { | ||
return this.nativeContext.beginPath(...args) | ||
} | ||
bezierCurveTo (...args) { | ||
return this.nativeContext.bezierCurveTo(...args) | ||
} | ||
clearRect (...args) { | ||
return this.nativeContext.clearRect(...args) | ||
} | ||
clip (...args) { | ||
return this.nativeContext.clip(...args) | ||
} | ||
closePath (...args) { | ||
return this.nativeContext.closePath(...args) | ||
} | ||
createImageData (width, height) { | ||
@@ -96,2 +234,6 @@ if (typeof width === 'object') { | ||
createLinearGradient (x0, y0, x1, y1) { | ||
return new CanvasGradient('linear', x0, y0, x1, y1) | ||
} | ||
createPattern (image, repetition = 'repeat') { | ||
@@ -103,6 +245,2 @@ const dye = image.nativeCanvas || image | ||
createLinearGradient (x0, y0, x1, y1) { | ||
return new CanvasGradient('linear', x0, y0, x1, y1) | ||
} | ||
createRadialGradient (x0, y0, r0, x1, y1, r1) { | ||
@@ -161,2 +299,14 @@ return new CanvasGradient('radial', x0, y0, x1, y1, r0, r1) | ||
fill (...args) { | ||
return this.nativeContext.fill(...args) | ||
} | ||
fillRect (...args) { | ||
return this.nativeContext.fillRect(...args) | ||
} | ||
fillText (...args) { | ||
return this.nativeContext.fillText(...args) | ||
} | ||
getImageData (sx, sy, sw, sh) { | ||
@@ -170,2 +320,20 @@ if (sw <= 0 || sh <= 0) { | ||
getTransform () { | ||
return new DOMMatrix( | ||
this.nativeContext.getTransform() | ||
) | ||
} | ||
lineTo (...args) { | ||
return this.nativeContext.lineTo(...args) | ||
} | ||
measureText (...args) { | ||
return this.nativeContext.measureText(...args) | ||
} | ||
moveTo (...args) { | ||
return this.nativeContext.moveTo(...args) | ||
} | ||
putImageData (imageData, dx, dy) { | ||
@@ -179,14 +347,30 @@ if (!(imageData instanceof ImageData)) { | ||
getTransform () { | ||
return new DOMMatrix( | ||
this.nativeContext.getTransform() | ||
) | ||
quadraticCurveTo (...args) { | ||
return this.nativeContext.quadraticCurveTo(...args) | ||
} | ||
setTransform (matrix) { | ||
if (matrix instanceof DOMMatrix) { | ||
this.nativeContext.setTransform(matrix.a, matrix.b, matrix.c, matrix.d, matrix.e, matrix.f) | ||
} | ||
rect (...args) { | ||
return this.nativeContext.rect(...args) | ||
} | ||
resetTransform () { | ||
return this.nativeContext.resetTransform() | ||
} | ||
restore (...args) { | ||
return this.nativeContext.restore(...args) | ||
} | ||
rotate (angle) { | ||
return this.nativeContext.rotate(+angle) | ||
} | ||
save (...args) { | ||
return this.nativeContext.save(...args) | ||
} | ||
scale (...args) { | ||
return this.nativeContext.scale(...args) | ||
} | ||
setLineDash (pattern) { | ||
@@ -211,12 +395,29 @@ if (!Array.isArray(pattern)) { | ||
rotate (angle) { | ||
return this.nativeContext.rotate(+angle) | ||
setTransform (matrix) { | ||
if (matrix instanceof DOMMatrix) { | ||
this.nativeContext.setTransform(matrix.a, matrix.b, matrix.c, matrix.d, matrix.e, matrix.f) | ||
} | ||
} | ||
stroke (...args) { | ||
return this.nativeContext.stroke(...args) | ||
} | ||
strokeRect (...args) { | ||
return this.nativeContext.strokeRect(...args) | ||
} | ||
strokeText (...args) { | ||
return this.nativeContext.strokeText(...args) | ||
} | ||
transform (...args) { | ||
return this.nativeContext.transform(...args) | ||
} | ||
translate (...args) { | ||
return this.nativeContext.translate(...args) | ||
} | ||
} | ||
const ProxyCanvasRenderingContext2D = createProxyToNative( | ||
CanvasRenderingContext2D, | ||
(target) => target.nativeContext | ||
) | ||
module.exports = ProxyCanvasRenderingContext2D | ||
module.exports = CanvasRenderingContext2D |
{ | ||
"name": "node-skia-canvas", | ||
"version": "0.1.0", | ||
"version": "0.1.1", | ||
"description": "Skia based graphics canvas run on node.js, written in Node-API", | ||
@@ -11,3 +11,3 @@ "main": "lib/index.js", | ||
"build:pre": "rimraf prebuilds && prebuild --backend cmake-js --runtime napi", | ||
"test": "jest", | ||
"test": "mocha", | ||
"ci": "npm run build:skia:release && npm run build:pre", | ||
@@ -36,3 +36,3 @@ "ci:debug": "npm run build:skia:debug && npm run build:dev", | ||
"author": "", | ||
"license": "ISC", | ||
"license": "MIT", | ||
"bugs": { | ||
@@ -52,7 +52,5 @@ "url": "https://github.com/leozdgao/node-skia-canvas/issues" | ||
"eslint-plugin-import": "^2.24.2", | ||
"eslint-plugin-jest": "^24.4.0", | ||
"eslint-plugin-node": "^11.1.0", | ||
"eslint-plugin-promise": "^5.1.0", | ||
"jest": "^26.6.3", | ||
"jest-environment-node-single-context": "^26.2.0", | ||
"mocha": "^9.1.2", | ||
"prebuild": "^11.0.0", | ||
@@ -59,0 +57,0 @@ "rimraf": "^3.0.2", |
311
README.md
@@ -1,39 +0,306 @@ | ||
# node-skia | ||
<div align="center"> | ||
[](http://standardjs.com) | ||
<image src="https://z3.ax1x.com/2021/10/12/5eGh36.png" width=300 /> | ||
Canvas work on node.js base skia. | ||
`node-skia-canvas` is a W3C Canvas API implementation for Node.js. It is based on Google [skia](https://skia.org/), and written with <b>Node-API</b>, provide similar canvas development just like on the browser. | ||
https://skia.org/user/build | ||
[]() | ||
[](./package.json) | ||
[](https://standardjs.com) | ||
[]() | ||
deps for env: | ||
* libjpeg-turbo | ||
* libpng | ||
* libwebp | ||
* icu4c | ||
* harfbuzz | ||
</div> | ||
## Setup | ||
## Introduction | ||
#### Node header files | ||
This project build with cmake-js, so if your node header files has never been installed, you could command below to install header fils first: | ||
There is already a Canvas works on node, but here is some enhancement, and take this package as a replacemnt of `node-canvas`: | ||
* Using Node-API to solve the ABI issue | ||
* Some skia module extension, for example: skparagraph, pathkit, sklottie | ||
* Working in node **worker-thread** to provide the possibility of multi-thread processing (Working in progress) | ||
* GPU context support (Working in progress) | ||
The project is written by C++ style API with `node-addon-api`, and built with `cmake-js`, and use `prebuild` and `prebuild-install` to prevent user enviroment building. | ||
Consider about the complex host environment, so this package decide to let skia deps be static linked, compiling features could check the build script `scripts/build_tools/build_skia.js` in this project. | ||
## Install | ||
```bash | ||
npx node-gyp install | ||
npm install --save node-canvas-skia | ||
``` | ||
#### Cmake | ||
## Example | ||
```js | ||
const { createCanvas } = require('node-skia-canvas') | ||
const canvas = createCanvas(256, 256) | ||
const ctx = canvas.getContext('2d') | ||
#### Build skia | ||
This project deeply depends on skia, it needs to be built first. To build skia, you should have ninja first, to install ninja, check https://ninja-build.org/ please. Then you could use command: | ||
ctx.fillStyle = '#FFF' | ||
ctx.fillRect(0, 0, 256, 256) | ||
ctx.fillStyle = '#4285F4' | ||
ctx.fillRect(10, 10, 100, 160) | ||
ctx.fillStyle = '#0F9D58' | ||
ctx.beginPath() | ||
ctx.arc(180, 50, 25, 0, 2 * Math.PI) | ||
ctx.closePath() | ||
ctx.fill() | ||
ctx.fillStyle = '#DB4437' | ||
ctx.beginPath() | ||
ctx.ellipse(100, 160, 50, 80, 0, 0, 2 * Math.PI) | ||
ctx.closePath() | ||
ctx.fill() | ||
ctx.lineWidth = 5 | ||
ctx.strokeStyle = '#F4B400' | ||
ctx.strokeRect(80, 50, 100, 160) | ||
``` | ||
And get result: | ||
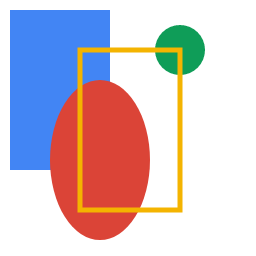 | ||
You could run example by the script in `scripts` folder: | ||
```bash | ||
cd skia | ||
python2 tools/git-sync-deps | ||
bin/gn gen out/Debug | ||
ninja -C out/Debug | ||
node scripts/run_example.js skia_showcase | ||
``` | ||
#### Use docker image | ||
## API Documentation | ||
Implement the Standard Canvas API: | ||
* `Canvas` | ||
* `CanvasRenderingContext2D` | ||
* `CanvasGradient` (not expose) | ||
* `CanvasPattern` (not expose) | ||
* `DOMMatrix` | ||
* `TextMetrix` (not expose) | ||
And also provide some useful module: | ||
* `Image`, for image use | ||
* `registerFont`, for font management | ||
### Canvas | ||
Differ from the creation of canvas in DOM, we create canvas by instantiation `Canvas`, or use `createCanvas`: | ||
```js | ||
// Instantication | ||
const canvas = new Canvas(300, 300) | ||
// or use factory method | ||
const canvas = createCanvas(300, 300) | ||
``` | ||
Properties: | ||
* [**width**][canvas_width] | ||
* [**height**][canvas_height] | ||
Methods: | ||
* [getContext()][getContext] | ||
* [toBuffer() ✨][toBuffer] | ||
* [toDataURL()][toDataURL_mdn] | ||
[canvas_width]: https://developer.mozilla.org/en-US/docs/Web/API/HTMLCanvasElement/width | ||
[canvas_height]: https://developer.mozilla.org/en-US/docs/Web/API/HTMLCanvasElement/height | ||
[getContext]: https://developer.mozilla.org/en-US/docs/Web/API/HTMLCanvasElement/getContext | ||
[toBuffer]: #tobufferformat | ||
[toDataURL_mdn]: https://developer.mozilla.org/en-US/docs/Web/API/HTMLCanvasElement/toDataURL | ||
##### ✨ `toBuffer(mimeType = 'image/png')` | ||
Not like canvas tag in browser, we need get real buffer information as result on server, so you could use this API, and it now support JPEG/PNG by pass mimeType, `image/png` will be used if format is not specified. | ||
```js | ||
const canvas = new Canvas(300, 300) | ||
const ctx = canvas.getContext('2d') | ||
// some canvas operation... | ||
canvas.toBuffer('image/png') // or image/jpeg | ||
``` | ||
### CanvasRenderingContext2D | ||
Use it just like in browser, the API is almost the same (only support `2d` for now, `gl` context is working in progress): | ||
```js | ||
const canvas = new Canvas(300, 300) | ||
const ctx = canvas.getContext('2d') | ||
ctx.fillStyle = '#FFF' | ||
ctx.fillRect(0, 0, 256, 256) | ||
``` | ||
Properties: | ||
* [fillStyle][fillStyle] | ||
* [font][font] | ||
* [globalAlpha][globalAlpha] | ||
* [globalCompositeOperation][globalCompositeOperation] | ||
* [imageSmoothingEnabled][imageSmoothingEnabled] | ||
* [lineCap][lineCap] | ||
* [lineDashOffset][lineDashOffset] | ||
* [lineJoin][lineJoin] | ||
* [lineWidth][lineWidth] | ||
* [miterLimit][miterLimit] | ||
* [shadowBlur][shadowBlur] | ||
* [shadowColor][shadowColor] | ||
* [shadowOffsetX][shadowOffsetX] | ||
* [shadowOffsetY][shadowOffsetY] | ||
* [strokeStyle][strokeStyle] | ||
* [textAlign][textAlign] | ||
* [textBaseline][textBaseline] | ||
Methods: | ||
* [arc()][arc()] | ||
* [arcTo()][arcTo()] | ||
* [beginPath()][beginPath()] | ||
* [bezierCurveTo()][bezierCurveTo()] | ||
* [clearRect()][clearRect()] | ||
* [clip()][clip()] | ||
* [closePath()][closePath()] | ||
* [createImageData()][createImageData()] | ||
* [createLinearGradient()][createLinearGradient()] | ||
* [createPattern()][createPattern()] | ||
* [createRadialGradient()][createRadialGradient()] | ||
* [drawImage()][drawImage()] | ||
* [ellipse()][ellipse()] | ||
* [fill()][fill()] | ||
* [fillRect()][fillRect()] | ||
* [fillText() ✨][fillText()] | ||
* [getImageData()][getImageData()] | ||
* [getLineDash()][getLineDash()] | ||
* [getTransform()][getTransform()] | ||
* [lineTo()][lineTo()] | ||
* [measureText()][measureText()] | ||
* [moveTo()][moveTo()] | ||
* [putImageData()][putImageData()] | ||
* [quadraticCurveTo()][quadraticCurveTo()] | ||
* [rect()][rect()] | ||
* [restore()][restore()] | ||
* [rotate()][rotate()] | ||
* [save()][save()] | ||
* [scale()][scale()] | ||
* [setLineDash()][setLineDash()] | ||
* [setTransform()][setTransform()] | ||
* [stroke()][stroke()] | ||
* [strokeRect()][strokeRect()] | ||
* [strokeText()][strokeText()] | ||
* [transform()][transform()] | ||
* [translate()][translate()] | ||
[fillStyle]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/fillStyle | ||
[font]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/font | ||
[globalAlpha]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/globalAlpha | ||
[globalCompositeOperation]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/globalCompositeOperation | ||
[imageSmoothingEnabled]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/imageSmoothingEnabled | ||
[lineCap]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/lineCap | ||
[lineDashOffset]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/lineDashOffset | ||
[lineJoin]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/lineJoin | ||
[lineWidth]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/lineWidth | ||
[miterLimit]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/miterLimit | ||
[shadowBlur]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/shadowBlur | ||
[shadowColor]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/shadowColor | ||
[shadowOffsetX]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/shadowOffsetX | ||
[shadowOffsetY]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/shadowOffsetY | ||
[strokeStyle]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/strokeStyle | ||
[textAlign]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/textAlign | ||
[textBaseline]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/textBaseline | ||
[arc()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/arc | ||
[arcTo()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/arcTo | ||
[beginPath()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/beginPath | ||
[bezierCurveTo()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/bezierCurveTo | ||
[clearRect()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/clearRect | ||
[clip()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/clip | ||
[closePath()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/closePath | ||
[createConicGradient()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/createConicGradient | ||
[createImageData()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/createImageData | ||
[createLinearGradient()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/createLinearGradient | ||
[createPattern()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/createPattern | ||
[createRadialGradient()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/createRadialGradient | ||
[drawFocusIfNeeded()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/drawFocusIfNeeded | ||
[drawImage()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/drawImage | ||
[ellipse()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/ellipse | ||
[fill()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/fill | ||
[fillRect()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/fillRect | ||
[fillText()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/fillText | ||
[getImageData()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/getImageData | ||
[getLineDash()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/getLineDash | ||
[getTransform()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/getTransform | ||
[isPointInPath()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/isPointInPath | ||
[isPointInStroke()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/isPointInStroke | ||
[lineTo()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/lineTo | ||
[measureText()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/measureText | ||
[moveTo()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/moveTo | ||
[putImageData()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/putImageData | ||
[quadraticCurveTo()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/quadraticCurveTo | ||
[rect()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/rect | ||
[resetTransform()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/resetTransform | ||
[restore()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/restore | ||
[rotate()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/rotate | ||
[save()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/save | ||
[scale()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/scale | ||
[setLineDash()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/setLineDash | ||
[setTransform()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/setTransform | ||
[stroke()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/stroke | ||
[strokeRect()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/strokeRect | ||
[strokeText()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/strokeText | ||
[transform()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/transform | ||
[translate()]: https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/translate | ||
##### ✨ `fillText(x, y, text, [maxWidth])` | ||
Provide extra parameter `maxWidth` to provide word-wrap typography, it is documented on [MDN](https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/fillText), but not implemented in most browser, but this library implemented this extra parameter. | ||
### Image | ||
Here is a helper class for image usage, and used in `drawImage` as parameter: | ||
```js | ||
const fs = require('fs') | ||
const { Image } = require('node-skia-canvas') | ||
const imgData = fs.readFileSync('./examples/leize.jpeg') | ||
const image = new Image() | ||
image.src = imgData | ||
const canvas = createCanvas(image.width, image.height) | ||
const ctx = canvas.getContext('2d') | ||
ctx.drawImage(img, 0, 0, image.width, image.height) | ||
``` | ||
Properties | ||
* [width](https://developer.mozilla.org/zh-CN/docs/Web/HTML/Element/img#attr-width) | ||
* [height](https://developer.mozilla.org/zh-CN/docs/Web/HTML/Element/img#attr-height) | ||
* [src](https://developer.mozilla.org/zh-CN/docs/Web/HTML/Element/img#attr-src) | ||
#### About loadImage | ||
There is an API `loadImage` provided by `node-canvas`, but you may have your own favorite network library like: `urllib`、`universal-fetch`, etc. So this `loadImage` helper api may not that helpful, so it will not be provided currently. | ||
### Font Management | ||
We provide font management API and compat with `node-canvas`: | ||
##### ✨ `registerFont(path, [{ family, weight }])` | ||
Register font to canvas, if `family` or `weight` is not provided, it will be parsed from font file automatically. | ||
```js | ||
const { registerFont } = require('node-skia-canvas') | ||
registerFont(path.join(__dirname, './OswaldRegular.ttf'), { | ||
family: 'Oswald' | ||
}) | ||
registerFont(path.join(__dirname, './OswaldLight.ttf')) | ||
registerFont(path.join(__dirname, './OswaldBold.ttf')) | ||
``` | ||
## Acknowledgements | ||
Thanks to `node-canvas` for their test cases. | ||
## License | ||
MIT |
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Mixed license
License(Experimental) Package contains multiple licenses.
Found 1 instance in 1 package
52272
10
0
1119
307