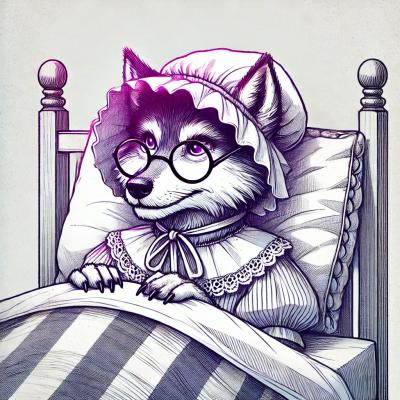
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The node-ssh npm package is a lightweight wrapper for SSH2, providing a simple and easy-to-use API for performing SSH operations. It allows you to connect to remote servers, execute commands, transfer files, and manage SSH connections programmatically.
Connecting to a remote server
This feature allows you to establish an SSH connection to a remote server using the provided host, username, and private key.
const { NodeSSH } = require('node-ssh');
const ssh = new NodeSSH();
ssh.connect({
host: 'example.com',
username: 'user',
privateKey: '/path/to/private/key'
}).then(() => {
console.log('Connected to the server');
}).catch(err => {
console.error('Error connecting to the server:', err);
});
Executing commands on a remote server
This feature allows you to execute shell commands on the connected remote server and retrieve the output.
ssh.execCommand('ls -la', { cwd: '/home/user' }).then(result => {
console.log('STDOUT: ' + result.stdout);
console.log('STDERR: ' + result.stderr);
}).catch(err => {
console.error('Error executing command:', err);
});
Transferring files to a remote server
This feature allows you to transfer files from your local machine to the remote server.
ssh.putFile('/local/path/to/file.txt', '/remote/path/to/file.txt').then(() => {
console.log('File transferred successfully');
}).catch(err => {
console.error('Error transferring file:', err);
});
Transferring files from a remote server
This feature allows you to download files from the remote server to your local machine.
ssh.getFile('/local/path/to/file.txt', '/remote/path/to/file.txt').then(() => {
console.log('File downloaded successfully');
}).catch(err => {
console.error('Error downloading file:', err);
});
The ssh2 package is a more low-level SSH client for Node.js, providing a comprehensive set of features for SSH connections, including command execution, file transfers, and tunneling. It offers more control and customization options compared to node-ssh but requires more boilerplate code.
The simple-ssh package is another lightweight SSH client for Node.js, focusing on simplicity and ease of use. It provides basic SSH functionalities like command execution and file transfers but lacks some of the advanced features and flexibility offered by node-ssh.
The ssh2-sftp-client package is a wrapper around the ssh2 package, specifically designed for SFTP operations. It simplifies file transfer operations over SFTP, making it easier to use for file management tasks compared to node-ssh, which provides a broader range of SSH functionalities.
Node-SSH is an extremely lightweight Promise wrapper for ssh2.
const fs = require('fs')
const path = require('path')
const {NodeSSH} = require('node-ssh')
const ssh = new NodeSSH()
ssh.connect({
host: 'localhost',
username: 'steel',
privateKey: '/home/steel/.ssh/id_rsa'
})
/*
Or
ssh.connect({
host: 'localhost',
username: 'steel',
privateKey: fs.readFileSync('/home/steel/.ssh/id_rsa', 'utf8')
})
if you want to use the raw string as private key
*/
.then(function() {
// Local, Remote
ssh.putFile('/home/steel/Lab/localPath/fileName', '/home/steel/Lab/remotePath/fileName').then(function() {
console.log("The File thing is done")
}, function(error) {
console.log("Something's wrong")
console.log(error)
})
// Array<Shape('local' => string, 'remote' => string)>
ssh.putFiles([{ local: '/home/steel/Lab/localPath/fileName', remote: '/home/steel/Lab/remotePath/fileName' }]).then(function() {
console.log("The File thing is done")
}, function(error) {
console.log("Something's wrong")
console.log(error)
})
// Local, Remote
ssh.getFile('/home/steel/Lab/localPath', '/home/steel/Lab/remotePath').then(function(Contents) {
console.log("The File's contents were successfully downloaded")
}, function(error) {
console.log("Something's wrong")
console.log(error)
})
// Putting entire directories
const failed = []
const successful = []
ssh.putDirectory('/home/steel/Lab', '/home/steel/Lab', {
recursive: true,
concurrency: 10,
// ^ WARNING: Not all servers support high concurrency
// try a bunch of values and see what works on your server
validate: function(itemPath) {
const baseName = path.basename(itemPath)
return baseName.substr(0, 1) !== '.' && // do not allow dot files
baseName !== 'node_modules' // do not allow node_modules
},
tick: function(localPath, remotePath, error) {
if (error) {
failed.push(localPath)
} else {
successful.push(localPath)
}
}
}).then(function(status) {
console.log('the directory transfer was', status ? 'successful' : 'unsuccessful')
console.log('failed transfers', failed.join(', '))
console.log('successful transfers', successful.join(', '))
})
// Command
ssh.execCommand('hh_client --json', { cwd:'/var/www' }).then(function(result) {
console.log('STDOUT: ' + result.stdout)
console.log('STDERR: ' + result.stderr)
})
// Command with escaped params
ssh.exec('hh_client', ['--json'], { cwd: '/var/www', stream: 'stdout', options: { pty: true } }).then(function(result) {
console.log('STDOUT: ' + result)
})
// With streaming stdout/stderr callbacks
ssh.exec('hh_client', ['--json'], {
cwd: '/var/www',
onStdout(chunk) {
console.log('stdoutChunk', chunk.toString('utf8'))
},
onStderr(chunk) {
console.log('stderrChunk', chunk.toString('utf8'))
},
})
})
// API reference in Typescript typing format:
import { Client, ConnectConfig, ClientChannel, SFTPWrapper, ExecOptions, PseudoTtyOptions | ShellOptions } from 'ssh2';
import { Prompt, TransferOptions } from 'ssh2-streams';
// ^ You do NOT need to import these package, these are here for reference of where the
// types are coming from.
declare type Config = ConnectConfig & {
host?: string;
port?: number;
username?: string;
password?: string;
privateKey?: string;
passphrase?: string;
tryKeyboard?: boolean;
onKeyboardInteractive?: (
name: string,
instructions: string,
lang: string,
prompts: Prompt[],
finish: (responses: string[]) => void
) => void;
};
interface SSHExecCommandOptions {
cwd?: string;
stdin?: string;
execOptions?: ExecOptions;
encoding?: BufferEncoding;
onChannel?: (clientChannel: ClientChannel) => void;
onStdout?: (chunk: Buffer) => void;
onStderr?: (chunk: Buffer) => void;
}
interface SSHExecCommandResponse {
stdout: string;
stderr: string;
code: number | null;
signal: string | null;
}
interface SSHExecOptions extends SSHExecCommandOptions {
stream?: 'stdout' | 'stderr' | 'both';
}
interface SSHPutFilesOptions {
sftp?: SFTPWrapper | null;
concurrency?: number;
transferOptions?: TransferOptions;
}
interface SSHGetPutDirectoryOptions extends SSHPutFilesOptions {
tick?: (localFile: string, remoteFile: string, error: Error | null) => void;
validate?: (path: string) => boolean;
recursive?: boolean;
}
class NodeSSH {
connection: Client | null;
connect(config: Config): Promise<this>;
isConnected(): boolean;
requestShell(
options?: PseudoTtyOptions | ShellOptions | false
): Promise<ClientChannel>;
withShell(
callback: (channel: ClientChannel) => Promise<void>,
options?: PseudoTtyOptions | ShellOptions | false
): Promise<void>;
requestSFTP(): Promise<SFTPWrapper>;
withSFTP(
callback: (sftp: SFTPWrapper) => Promise<void>
): Promise<void>;
execCommand(
command: string,
options?: SSHExecCommandOptions
): Promise<SSHExecCommandResponse>;
exec(
command: string,
parameters: string[],
options?: SSHExecOptions & {
stream?: 'stdout' | 'stderr';
}
): Promise<string>;
exec(
command: string,
parameters: string[],
options?: SSHExecOptions & {
stream: 'both';
}
): Promise<SSHExecCommandResponse>;
mkdir(
path: string,
method?: 'sftp' | 'exec',
sftp?: SFTPWrapper | null
): Promise<void>;
getFile(
localFile: string,
remoteFile: string,
sftp?: SFTPWrapper | null,
transferOptions?: TransferOptions | null
): Promise<void>;
putFile(
localFile: string,
remoteFile: string,
sftp?: SFTPWrapper | null,
transferOptions?: TransferOptions | null
): Promise<void>;
putFiles(files: Array<{
local: string;
remote: string;
}>, options?: SSHPutFilesOptions): Promise<void>;
putDirectory(
localDirectory: string,
remoteDirectory: string,
options?: SSHGetPutDirectoryOptions
): Promise<boolean>;
getDirectory(
localDirectory: string,
remoteDirectory: string,
options?: SSHGetPutDirectoryOptions
): Promise<boolean>;
dispose(): void;
}
module.exports = NodeSSH;
In some cases you have to enable keyboard-interactive user authentication. Otherwise you will get an All configured authentication methods failed
error.
const password = 'test'
ssh.connect({
host: 'localhost',
username: 'steel',
port: 22,
password,
tryKeyboard: true,
})
// Or if you want to add some custom keyboard-interactive logic:
ssh.connect({
host: 'localhost',
username: 'steel',
port: 22,
tryKeyboard: true,
onKeyboardInteractive(name, instructions, instructionsLang, prompts, finish) {
if (prompts.length > 0 && prompts[0].prompt.toLowerCase().includes('password')) {
finish([password])
}
}
})
For further information see: https://github.com/mscdex/ssh2/issues/604
This project is licensed under the terms of MIT license. See the LICENSE file for more info.
FAQs
SSH2 with Promises
The npm package node-ssh receives a total of 45,559 weekly downloads. As such, node-ssh popularity was classified as popular.
We found that node-ssh demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.