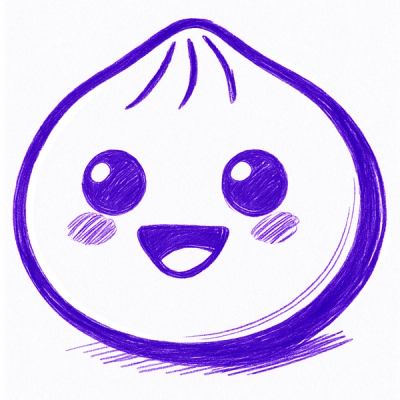
Security News
Bun 1.2.19 Adds Isolated Installs for Better Monorepo Support
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.
node-vk-bot-api
Advanced tools
🤖 VK bot framework for Node.js, based on Bots Long Poll API and Callback API
🤖 VK bot framework for Node.js, based on Bots Long Poll API and Callback API.
$ npm i node-vk-bot-api -S
const VkBot = require('node-vk-bot-api');
const bot = new VkBot(process.env.TOKEN);
bot.command('/start', (ctx) => {
ctx.reply('Hello!');
});
bot.startPolling();
const express = require('express');
const bodyParser = require('body-parser');
const VkBot = require('node-vk-bot-api');
const app = express();
const bot = new VkBot({
token: process.env.TOKEN,
confirmation: process.env.CONFIRMATION,
});
bot.on((ctx) => {
ctx.reply('Hello!');
});
app.use(bodyParser.json());
app.post('/', bot.webhookCallback);
app.listen(process.env.PORT);
There's a few simple examples.
Any questions you can ask in the telegram chat. [russian/english]
$ npm test
const api = require('node-vk-bot-api/lib/api');
api('users.get', {
user_ids: 1,
access_token: process.env.TOKEN,
}); // => Promise
// bad
bot.command('/start', (ctx) => {
ctx.reply('Hello, world!');
});
// not bad
bot.command('/start', async (ctx) => {
try {
await ctx.reply('Hello, world!');
} catch (e) {
console.error(e);
}
});
// good
bot.use(async (ctx, next) => {
try {
await next();
} catch (e) {
console.error(e);
}
});
bot.command('/start', async (ctx) => {
await ctx.reply('Hello, world!');
});
// bad
bot.startPolling();
// good
bot.startPolling((err) => {
if (err) {
console.error(err);
}
});
Create bot.
// Simple usage
const bot = new VkBot(process.env.TOKEN);
// Advanced usage
const bot = new VkBot({
token: process.env.TOKEN,
group_id: process.env.GROUP_ID,
execute_timeout: process.env.EXECUTE_TIMEOUT, // in ms (50 by default)
polling_timeout: process.env.POLLING_TIMEOUT, // in secs (25 by default)
// webhooks options only
secret: process.env.SECRET, // secret key (optional)
confirmation: process.env.CONFIRMATION, // confirmation string
});
Execute request to the VK API.
const response = await bot.execute('users.get', {
user_ids: 1,
});
Add simple middleware.
bot.use((ctx, next) => {
ctx.message.timestamp = new Date().getTime();
return next();
});
Add middlewares with triggers for message_new
event.
bot.command('start', (ctx) => {
ctx.reply('Hello!');
});
Add middlewares with triggers for selected events.
bot.event('message_edit', (ctx) => {
ctx.reply('Your message was editted');
});
Add reserved middlewares without triggers.
bot.on((ctx) => {
ctx.reply('No commands for you.');
});
Send message to user.
// Simple usage
bot.sendMessage(145003487, 'Hello!', 'photo1_1');
// Multiple recipients
bot.sendMessage([145003487, 145003488], 'Hello!', 'photo1_1');
// Advanced usage
bot.sendMessage(145003487, {
message: 'Hello!',
lat: 59.939095,
lng: 30.315868,
});
Start polling with optional callback.
bot.startPolling((err) => {
if (err) {
console.error(err);
}
});
Get webhook callback.
// express
bot.webhookCallback(req, res, next);
// koa
bot.webhookCallback(ctx, next);
Stop the bot. Disables any receiving updates from Long Poll or Callback APIs.
bot.stop();
Start the bot after it was turned off via .stop() method. When you are using Long Poll API, you need to call .startPolling([callback])
again.
bot.start();
message
- received message (pure object from VK API)
type
- received type event (e.g. message_new)eventId
- callback's eventIdgroupId
- callback's groupIdmatch?
- regexp match of your triggerclientInfo?
- received client info (pure object from VK API)bot
- instance of bot, you can call any methods via this instanceHelper method for reply to the current user.
bot.command('start', (ctx) => {
ctx.reply('Hello!');
});
Markup.keyboard(buttons, options)
: Create keyboardMarkup.button(label, color, payload)
: Create custom buttonMarkup.oneTime()
: Set oneTime to keyboardctx.reply('Select your sport', null, Markup
.keyboard([
'Football',
'Basketball',
])
.oneTime(),
);
// custom buttons
ctx.reply('Hey!', null, Markup
.keyboard([
Markup.button({
action: {
type: 'open_link',
link: 'https://google.com',
label: 'Open Google',
payload: JSON.stringify({
url: 'https://google.com',
}),
},
color: 'default',
}),
]),
);
// default buttons
ctx.reply('How are you doing?', null, Markup
.keyboard([
[
Markup.button('Normally', 'primary'),
],
[
Markup.button('Fine', 'positive'),
Markup.button('Bad', 'negative'),
],
]),
);
Create keyboard with optional settings.
/*
Each string has maximum 2 columns.
| one | two |
| three | four |
| five | six |
*/
Markup.keyboard([
'one',
'two',
'three',
'four',
'five',
'six',
], { columns: 2 });
/*
By default, columns count for each string is 4.
| one | two | three |
*/
Markup.keyboard([
'one',
'two',
'three',
]);
Create custom button.
Markup.button('Start', 'positive', {
foo: 'bar',
});
Helper method for create one time keyboard.
Markup
.keyboard(['Start', 'Help'])
.oneTime();
Helpers method for create inline keyboard.
Markup
.keyboard(['Start', 'Help'])
.inline();
Store anything for current user in local (or redis) memory.
const VkBot = require('node-vk-bot-api');
const Session = require('node-vk-bot-api/lib/session');
const bot = new VkBot(process.env.TOKEN);
const session = new Session();
bot.use(session.middleware());
bot.on((ctx) => {
ctx.session.counter = ctx.session.counter || 0;
ctx.session.counter++;
ctx.reply(`You wrote ${ctx.session.counter} messages.`);
});
bot.startPolling();
key
: Context property name (default: session
)getSessionKey
: Getter for session keygetSessionKey(ctx)
const getSessionKey = (ctx) => {
const userId = ctx.message.from_id || ctx.message.user_id;
return `${userId}:${userId}`;
};
Scene manager.
const VkBot = require('node-vk-bot-api');
const Scene = require('node-vk-bot-api/lib/scene');
const Session = require('node-vk-bot-api/lib/session');
const Stage = require('node-vk-bot-api/lib/stage');
const bot = new VkBot(process.env.TOKEN);
const scene = new Scene('meet',
(ctx) => {
ctx.scene.next();
ctx.reply('How old are you?');
},
(ctx) => {
ctx.session.age = +ctx.message.text;
ctx.scene.next();
ctx.reply('What is your name?');
},
(ctx) => {
ctx.session.name = ctx.message.text;
ctx.scene.leave();
ctx.reply(`Nice to meet you, ${ctx.session.name} (${ctx.session.age} years old)`);
},
);
const session = new Session();
const stage = new Stage(scene);
bot.use(session.middleware());
bot.use(stage.middleware());
bot.command('/meet', (ctx) => {
ctx.scene.enter('meet');
});
bot.startPolling();
constructor(...scenes)
: Register scenesconstructor(name, ...middlewares)
: Create scene.command(triggers, ...middlewares)
: Create commands for scenectx.scene.enter(name, [step]) // Enter in scene
ctx.scene.leave() // Leave from scene
ctx.scene.next() // Go to the next step in scene
ctx.scene.step // Getter for step in scene
ctx.scene.step= // Setter for step in scene
MIT.
FAQs
🤖 VK bot framework for Node.js, based on Bots Long Poll API and Callback API
The npm package node-vk-bot-api receives a total of 198 weekly downloads. As such, node-vk-bot-api popularity was classified as not popular.
We found that node-vk-bot-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.
Security News
Popular npm packages like eslint-config-prettier were compromised after a phishing attack stole a maintainer’s token, spreading malicious updates.
Security News
/Research
A phishing attack targeted developers using a typosquatted npm domain (npnjs.com) to steal credentials via fake login pages - watch out for similar scams.