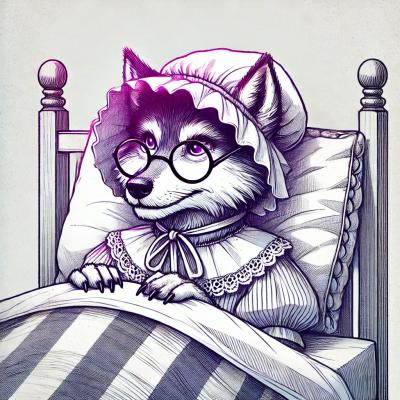
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
node-watch
Advanced tools
The node-watch package is a simple and efficient file system watcher for Node.js. It allows you to monitor changes in files and directories, making it useful for tasks such as live-reloading, automated testing, and more.
Watch a single file
This feature allows you to watch a single file for changes. The callback function is triggered whenever the file is modified.
const watch = require('node-watch');
watch('file.txt', { recursive: false }, function(evt, name) {
console.log('%s changed.', name);
});
Watch a directory recursively
This feature allows you to watch a directory and all its subdirectories for changes. The callback function is triggered whenever any file within the directory or its subdirectories is modified.
const watch = require('node-watch');
watch('directory', { recursive: true }, function(evt, name) {
console.log('%s changed.', name);
});
Filter files to watch
This feature allows you to specify a filter to watch only certain types of files. In this example, only JavaScript files within the directory are monitored for changes.
const watch = require('node-watch');
watch('directory', { filter: /\.js$/ }, function(evt, name) {
console.log('%s changed.', name);
});
Chokidar is a highly efficient and feature-rich file system watcher for Node.js. It supports recursive watching, filtering, and more advanced features like debouncing and throttling. Compared to node-watch, Chokidar offers more configuration options and better performance for large-scale projects.
Gaze is another file watcher for Node.js that supports recursive watching and filtering. It is known for its simplicity and ease of use. While it offers similar functionalities to node-watch, it may not be as performant or feature-rich as Chokidar.
The watch package is a simple file watcher for Node.js. It provides basic functionality for monitoring file changes but lacks some of the advanced features found in Chokidar and node-watch. It is suitable for smaller projects or simpler use cases.
A wrapper and enhancements for fs.watch.
npm install node-watch
var watch = require('node-watch');
watch('file_or_dir', { recursive: true }, function(evt, name) {
console.log('%s changed.', name);
});
Now it's fast to watch deep directories on macOS and Windows, since the recursive
option is natively supported except on Linux.
// watch the whole disk
watch('/', { recursive: true }, console.log);
The usage and options of node-watch
are compatible with fs.watch.
persistent: Boolean
(default true)recursive: Boolean
(default false)encoding: String
(default 'utf8')Extra options
filter: RegExp | Function
Return that matches the filter expression.
// filter with regular expression
watch('./', { filter: /\.json$/ });
// filter with custom function
watch('./', { filter: f => !/node_modules/.test(f) });
Each file and directory will be passed to the filter to determine whether
it will then be passed to the callback function. Like Array.filter
does in JavaScript
.
There are three kinds of return values for filter function:
true
: Will be passed to callback.false
: Will not be passed to callback.skip
: Same with false
, and skip to watch all its subdirectories.On Linux, where the recursive
option is not natively supported,
it is more efficient to skip ignored directories by returning the skip
flag:
watch('./', {
resursive: true,
filter(f, skip) {
// skip node_modules
if (/\/node_modules/.test(f)) return skip;
// skip .git folder
if (/\.git/.test(f)) return skip;
// only watch for js files
return /\.js$/.test(f);
}
});
If you prefer glob patterns you can use minimatch or picomatch together with filter:
const pm = require('picomatch');
let isMatch = pm('*.js');
watch('./', {
filter: f => isMatch(f)
});
delay: Number
(in ms, default 200)
Delay time of the callback function.
// log after 5 seconds
watch('./', { delay: 5000 }, console.log);
The events provided by the callback function is either update
or remove
, which is less confusing to fs.watch
's rename
or change
.
watch('./', function(evt, name) {
if (evt == 'update') {
// on create or modify
}
if (evt == 'remove') {
// on delete
}
});
The watch function returns a fs.FSWatcher like object as the same as fs.watch
(>= v0.4.0).
let watcher = watch('./', { recursive: true });
watcher.on('change', function(evt, name) {
// callback
});
watcher.on('error', function(err) {
// handle error
});
watcher.on('ready', function() {
// the watcher is ready to respond to changes
});
// close
watcher.close();
// is closed?
watcher.isClosed()
.on
.once
.emit
.close
.listeners
.setMaxListeners
.getMaxListeners
.isClosed
detect if the watcher is closed.getWatchedPaths
get all the watched pathsWindows, node < v4.2.5
remove
eventMacOS, node 0.10.x
watch(['file1', 'file2'], console.log);
#!/usr/bin/env node
// https://github.com/nodejs/node-v0.x-archive/issues/3211
require('epipebomb')();
let watcher = require('node-watch')(
process.argv[2] || './', { recursive: true }, console.log
);
process.on('SIGINT', watcher.close);
Monitoring chrome from disk:
$ watch / | grep -i chrome
If you get ENOSPC error, but you actually have free disk space - it means that your OS watcher limit is too low and you probably want to recursively watch a big tree of files.
Follow this description to increase the limit: https://confluence.jetbrains.com/display/IDEADEV/Inotify+Watches+Limit
Thanks goes to these wonderful people (emoji key):
Yuan Chuan 💻 📖 ⚠️ | Greg Thornton 💻 🤔 | Amir Arad 💻 📖 ⚠️ | Gary Burgess 💻 | Peter deHaan 💻 | kcliu 💻 | Hoovinator 💬 |
---|---|---|---|---|---|---|
Steve Shreeve 💻 | Blake Regalia 💻 | Mike Collins 💻 | Timo Tijhof 💻 | Bailey Herbert 💻 | Anthony Rey 💻 |
This project follows the all-contributors specification. Contributions of any kind welcome!
MIT
Copyright (c) 2020 yuanchuan
FAQs
A wrapper and enhancements for fs.watch
The npm package node-watch receives a total of 106,546 weekly downloads. As such, node-watch popularity was classified as popular.
We found that node-watch demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.