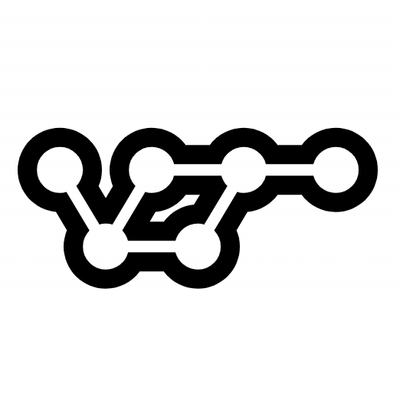
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
nodejs-file-downloader
Advanced tools
nodejs-file-downloader is a simple utility for downloading files. It hides the complexity of dealing with streams, paths and duplicate file names.
$ npm install nodejs-file-downloader
Download a large file with default configuration
const Downloader = require('nodejs-file-downloader');
(async () => {//Wrapping the code with an async function, just for the sake of example.
const downloader = new Downloader({
url: 'http://212.183.159.230/200MB.zip',//If the file name already exists, a new file with the name 200MB1.zip is created.
directory: "./downloads",//This folder will be created, if it doesn't exist.
})
await downloader.download();//Downloader.download() returns a promise.
console.log('All done');
})();
const Downloader = require('nodejs-file-downloader');
(async () => {
const downloader = new Downloader({
url: 'http://212.183.159.230/200MB.zip',
directory: "./downloads/2020/May",//Sub directories will also be automatically created if they do not exist.
})
downloader.on('progress',(percentage)=>{//Downloader is an event emitter. You can register a "progress" event.
console.log('% ',percentage)
})
await downloader.download();
})();
nodejs-file-downloader "deduces" the file name, from the URL or the response headers. If you want to overwrite it, supply a config.fileName property.
const downloader = new Downloader({
url: 'http://212.183.159.230/200MB.zip',
directory: "./downloads/2020/May",
fileName:'somename.zip'//This will be the file name.
})
By default, nodejs-file-downloader uses config.cloneFiles = true, which means that files with an existing name, will have a number appended to them.
const downloader = new Downloader({
url: 'http://212.183.159.230/200MB.zip',
directory: "./",
cloneFiles:false//This will cause the downloader to re-write an existing file.
})
FAQs
A file downloader for NodeJs
The npm package nodejs-file-downloader receives a total of 46,323 weekly downloads. As such, nodejs-file-downloader popularity was classified as popular.
We found that nodejs-file-downloader demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.