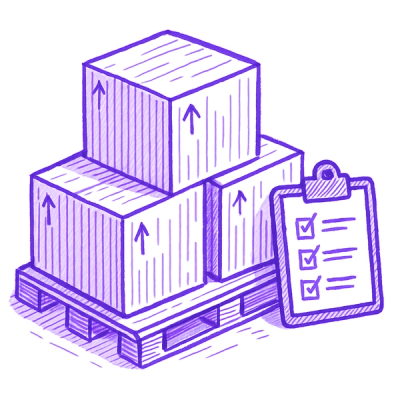
Security News
Open Source Maintainers Feeling the Weight of the EU’s Cyber Resilience Act
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
nodejs-itunes-api
Advanced tools
Support manage app certificate, devices and provisioning-profile by nodejs
npm install nodejs-itunes-api
let privateKey = fs.readFileSync(/* file of p8 key */));
let itunes = new iTunes({
"apiKey": "API_KEY",
"issuerId": "ISSUER_ID",
"privateKey": privateKey
});
itunes.getAllCertificate()
.then(function(response) {
/* Query certificate result */
})
.catch(error => {
console.error(error);
});
IOS_DEVELOPMENT
, IOS_DISTRIBUTION
, DEVELOPMENT
, DISTRIBUTION
const CertificateType = itunes.CertificateType;
itunes.getCertificate(CertificateType.DEVELOPMENT)
.then(function(response) {
/* Query certificate result */
})
.catch(error => {
console.error(error);
});
const DEVICE_NAME = "DEVICE NAME";
const UDID = "UDID";
const DeviceType = itunes.DeviceType;
itunes.registerDevice(DEVICE_NAME, UDID, DeviceType.IOS)
.then(function(response) {
/* register result */
})
.catch(error => {
console.error(error);
});
itunes.getDeviceList()
.then(function(response) {
/* Query device list */
})
.catch(error => {
console.error(error);
});
const BUNDLE_ID_NAME = "BUNDLE_ID_NAME";
const BUNDLE_ID = "BUNDLE_ID";
const DeviceType = itunes.DeviceType;
itunes.createBundleId(BUNDLE_ID_NAME, BUNDLE_ID, DeviceType.IOS)
.then(function(response) {
/* Create bundle id result*/
})
.catch(error => {
console.error(error);
});
const BUNDLE_ID_NAME = "BUNDLE_ID_NAME";
const BUNDLE_ID = "BUNDLE_ID";
const DeviceType = itunes.DeviceType;
const Capability = itunes.CapabilityType;
itunes.createBundleId(BUNDLE_ID_NAME, BUNDLE_ID, DeviceType.IOS, Capability.PUSH_NOTIFICATIONS)
.then(function(response) {
/* Create bundle id result*/
})
.catch(error => {
console.error(error);
});
const BUNDLE_ID_NAME = "BUNDLE_ID_NAME";
const BUNDLE_ID = "BUNDLE_ID";
const DeviceType = itunes.DeviceType;
const Capability = itunes.CapabilityType;
itunes.createBundleId(BUNDLE_ID_NAME, BUNDLE_ID, DeviceType.IOS, [Capability.PUSH_NOTIFICATIONS, Capability.ICLOUD])
.then(function(response) {
/* Create bundle id result*/
})
.catch(error => {
console.error(error);
});
const BUNDLE_ID = "ID of Bundle-ID";
itunes.deleleBundleId(BUNDLE_ID)
.then(function() {
/* delete bundle-id success */
}).catch(error => {
console.error(error);
});
IOS_APP_DEVELOPMENT
, IOS_APP_STORE
, IOS_APP_ADHOC
, IOS_APP_INHOUSE
, MAC_APP_DEVELOPMENT
, MAC_APP_STORE
, MAC_APP_DIRECT
, TVOS_APP_DEVELOPMENT
, TVOS_APP_STORE
, TVOS_APP_ADHOC
, TVOS_APP_INHOUSE
, MAC_CATALYST_APP_DEVELOPMENT
, MAC_CATALYST_APP_STORE
and MAC_CATALYST_APP_DIRECT
const ProfileType = itunes.ProfileType;
const CERT_ID = "CERTIFICATE_ID";
const BUNDLE_ID = "ID of Bundle-ID";
const PROFILE_NAME = "PROFILE_NAME";
itunes.getDeviceList()
.then(function(response) {
let deviceIdList = response.data.map(function(info) {
return info.id;
});
return itunes.createProfile(PROFILE_NAME, CERT_ID, BUNDLE_ID, deviceIdList, ProfileType.IOS_APP_DEVELOPMENT)
.then(function(response) {
/* create profile result */
})
.catch(error => {
console.error(error);
});
})
.catch(error => {
console.error(error);
});
const ProfileType = itunes.ProfileType;
const CERT_ID = "CERTIFICATE_ID";
const BUNDLE_ID = "ID of Bundle-ID";
const PROFILE_NAME = "PROFILE_NAME";
itunes.getDeviceList()
.then(function(response) {
let deviceIdList = response.data.map(function(info) {
return info.id;
});
return itunes.createProfile(PROFILE_NAME, CERT_ID, BUNDLE_ID, deviceIdList, ProfileType.IOS_APP_DEVELOPMENT);
})
.then(function(response) {
let profileId = response.data.id;
return itunes.deleteProfile(profileId);
})
.then(function() {
/* delete profile success */
})
.catch(error => {
console.error(error);
});
let ProfileType = itunes.ProfileType;
let ProfileState = itunes.ProfileState;
itunes.getProfile(ProfileType.IOS_APP_DEVELOPMENT, ProfileState.ACTIVE)
.then(function(result) {
let dirPath = /* directory path */;
for (let i = 0; i < result.data.length; i += 1) {
let profile = result.data[i];
let fileName = `${profile.getName()}.mobileprovision`;
let filePath = path.join(dirPath, fileName);
profile.save(filePath);
}
})
.catch(error => {
console.error(error);
});
Fixed device rename by UDID
Support create bundle-id
Support bundle-id capabilities
Support delete bundle-id
Support create profile
Support delete profile
Support modify profile (delete original profile and create new profile)
Support bundle-id filter
FAQs
Support manage app certificate, devices and provisioning-profile by nodejs
The npm package nodejs-itunes-api receives a total of 0 weekly downloads. As such, nodejs-itunes-api popularity was classified as not popular.
We found that nodejs-itunes-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.