nodejs-polars
Advanced tools
Comparing version 0.0.5 to 0.0.6
@@ -1278,11 +1278,14 @@ /// <reference types="node" /> | ||
*/ | ||
export declare function DataFrame(): DataFrame; | ||
export declare function DataFrame(data: Record<string, any>): DataFrame; | ||
export declare function DataFrame(data: Record<string, any>[]): DataFrame; | ||
export declare function DataFrame(data: Series<any>[]): DataFrame; | ||
export declare function DataFrame(data: any[][]): DataFrame; | ||
export declare function DataFrame(data: any[][], options: { | ||
declare function _DataFrame(): DataFrame; | ||
declare function _DataFrame(data: Record<string, any>): DataFrame; | ||
declare function _DataFrame(data: Record<string, any>[]): DataFrame; | ||
declare function _DataFrame(data: Series<any>[]): DataFrame; | ||
declare function _DataFrame(data: any[][]): DataFrame; | ||
declare function _DataFrame(data: any[][], options: { | ||
columns?: any[]; | ||
orient?: "row" | "col"; | ||
}): DataFrame; | ||
export declare const DataFrame: typeof _DataFrame & { | ||
isDataFrame: (ty: any) => ty is DataFrame; | ||
}; | ||
export {}; |
@@ -12,5 +12,7 @@ "use strict"; | ||
const functions_1 = require("./functions"); | ||
const expr_1 = require("./lazy/expr"); | ||
const error_1 = require("./error"); | ||
const series_1 = require("./series"); | ||
const stream_1 = require("stream"); | ||
const types_1 = require("util/types"); | ||
const datatypes_1 = require("./datatypes"); | ||
@@ -20,3 +22,3 @@ const utils_1 = require("./utils"); | ||
function prepareOtherArg(anyValue) { | ||
if ((0, utils_1.isSeries)(anyValue)) { | ||
if (series_1.Series.isSeries(anyValue)) { | ||
return anyValue; | ||
@@ -47,3 +49,3 @@ } | ||
let start = 0; | ||
let len = this.height; | ||
let len = this.width; | ||
while (start < len) { | ||
@@ -74,3 +76,3 @@ const s = this.toSeries(start); | ||
const describeCast = (df) => { | ||
return DataFrame(df.getColumns().map(s => { | ||
return (0, exports.DataFrame)(df.getColumns().map(s => { | ||
if (s.isNumeric() || s.isBoolean()) { | ||
@@ -114,10 +116,9 @@ return s.cast(datatypes_1.DataType.Float64); | ||
}, | ||
dropDuplicates(opts = true, subset) { | ||
if (opts?.maintainOrder !== undefined) { | ||
return this.dropDuplicates(opts.maintainOrder, opts.subset); | ||
dropDuplicates(opts = false, subset) { | ||
const maintainOrder = opts?.maintainOrder ?? opts; | ||
subset = opts?.subset ?? subset; | ||
if (typeof subset === "string") { | ||
subset = [subset]; | ||
} | ||
if (subset) { | ||
subset = [subset].flat(2); | ||
} | ||
return wrap("drop_duplicates", { maintainOrder: opts.maintainOrder, subset }); | ||
return wrap("drop_duplicates", { maintainOrder, subset }); | ||
}, | ||
@@ -269,3 +270,3 @@ explode(...columns) { | ||
select(...selection) { | ||
const hasExpr = selection.flat().some(s => (0, utils_1.isExpr)(s)); | ||
const hasExpr = selection.flat().some(s => expr_1.Expr.isExpr(s)); | ||
if (hasExpr) { | ||
@@ -308,3 +309,3 @@ return (0, exports.dfWrapper)(_df) | ||
} | ||
if (Array.isArray(arg) || (0, utils_1.isExpr)(arg)) { | ||
if (Array.isArray(arg) || expr_1.Expr.isExpr(arg)) { | ||
return (0, exports.dfWrapper)(_df).lazy() | ||
@@ -417,3 +418,3 @@ .sort(arg, reverse) | ||
withColumn(column) { | ||
if ((0, utils_1.isSeries)(column)) { | ||
if (series_1.Series.isSeries(column)) { | ||
return wrap("with_column", { _series: column._series }); | ||
@@ -451,9 +452,6 @@ } | ||
return new Proxy(df, { | ||
get: function (target, prop, receiver) { | ||
get(target, prop, receiver) { | ||
if (typeof prop === "string" && target.columns.includes(prop)) { | ||
return target.getColumn(prop); | ||
} | ||
if (Array.isArray(prop) && target.columns.includes(prop[0])) { | ||
return target.select(prop); | ||
} | ||
if (typeof prop !== "symbol" && !Number.isNaN(Number(prop))) { | ||
@@ -466,4 +464,29 @@ return target.row(Number(prop)); | ||
}, | ||
has: function (target, p) { | ||
set(target, prop, receiver) { | ||
if (series_1.Series.isSeries(receiver)) { | ||
if (typeof prop === "string" && target.columns.includes(prop)) { | ||
const idx = target.columns.indexOf(prop); | ||
target.replaceAtIdx(idx, receiver.alias(prop)); | ||
return true; | ||
} | ||
if (typeof prop !== "symbol" && !Number.isNaN(Number(prop))) { | ||
target.replaceAtIdx(Number(prop), receiver); | ||
return true; | ||
} | ||
} | ||
Reflect.set(target, prop, receiver); | ||
return true; | ||
}, | ||
has(target, p) { | ||
return target.columns.includes(p); | ||
}, | ||
ownKeys(target) { | ||
return target.columns; | ||
}, | ||
getOwnPropertyDescriptor(target, prop) { | ||
return { | ||
configurable: true, | ||
enumerable: true, | ||
value: target.getColumn(prop) | ||
}; | ||
} | ||
@@ -475,3 +498,3 @@ }); | ||
exports._wrapDataFrame = _wrapDataFrame; | ||
function DataFrame(data, options) { | ||
function _DataFrame(data, options) { | ||
if (!data) { | ||
@@ -485,6 +508,12 @@ return (0, exports.dfWrapper)(objToDF({})); | ||
} | ||
exports.DataFrame = DataFrame; | ||
function objToDF(obj) { | ||
const columns = Object.entries(obj).map(([key, value]) => (0, series_1.Series)(key, value)._series); | ||
const columns = Object.entries(obj).map(([name, values]) => { | ||
if (series_1.Series.isSeries(values)) { | ||
return values.rename(name)._series; | ||
} | ||
return (0, series_1.Series)(name, values)._series; | ||
}); | ||
return polars_internal_1.default.df.read_columns({ columns }); | ||
} | ||
const isDataFrame = (ty) => (0, types_1.isExternal)(ty?._df); | ||
exports.DataFrame = Object.assign(_DataFrame, { isDataFrame }); |
/// <reference types="node" /> | ||
import { Stream } from "stream"; | ||
export declare type DtypeToPrimitive<T> = T extends DataType.Bool ? boolean : T extends DataType.Utf8 ? string : T extends DataType.Datetime ? number | Date : T extends DataType.Date ? Date : T extends DataType.UInt64 ? bigint : T extends DataType.Int64 ? bigint : number; | ||
export declare type DtypeToPrimitive<T> = T extends DataType.Bool ? boolean : T extends DataType.Utf8 ? string : T extends DataType.Categorical ? string : T extends DataType.Datetime ? number | Date : T extends DataType.Date ? Date : T extends DataType.UInt64 ? bigint : T extends DataType.Int64 ? bigint : number; | ||
export declare type PrimitiveToDtype<T> = T extends boolean ? DataType.Bool : T extends string ? DataType.Utf8 : T extends Date ? DataType.Datetime : T extends number ? DataType.Float64 : T extends bigint ? DataType.Int64 : T extends ArrayLike<any> ? DataType.List : DataType.Object; | ||
@@ -5,0 +5,0 @@ export declare type TypedArray = Int8Array | Int16Array | Int32Array | BigInt64Array | Uint8Array | Uint16Array | Uint32Array | BigInt64Array | Float32Array | Float64Array; |
@@ -64,2 +64,3 @@ "use strict"; | ||
Utf8: "new_str", | ||
Categorical: "new_str", | ||
Object: "new_object", | ||
@@ -66,0 +67,0 @@ List: "new_list", |
@@ -113,2 +113,5 @@ "use strict"; | ||
} | ||
if (dtype === datatypes_1.DataType.Categorical) { | ||
series = polars_internal_1.default.series.cast({ _series: series, dtype, strict: false }); | ||
} | ||
return series; | ||
@@ -115,0 +118,0 @@ } |
@@ -7,4 +7,4 @@ "use strict"; | ||
// eslint-disable-next-line no-undef | ||
const up1 = (0, path_1.join)(__dirname, "../"); | ||
const bindings = (0, helper_1.loadBinding)(up1, "nodejs-polars", "nodejs-polars"); | ||
const up2 = (0, path_1.join)(__dirname, "../", "../"); | ||
const bindings = (0, helper_1.loadBinding)(up2, "nodejs-polars", "nodejs-polars"); | ||
exports.default = bindings; |
@@ -35,10 +35,9 @@ "use strict"; | ||
drop: (...cols) => wrap("dropColumns", { cols: cols.flat(2) }), | ||
dropDuplicates(opts = true, subset) { | ||
if (opts?.maintainOrder !== undefined) { | ||
return this.dropDuplicates(opts.maintainOrder, opts.subset); | ||
dropDuplicates(opts = false, subset) { | ||
const maintainOrder = opts?.maintainOrder ?? opts; | ||
subset = opts?.subset ?? subset; | ||
if (typeof subset === "string") { | ||
subset = [subset]; | ||
} | ||
if (subset) { | ||
subset = [subset].flat(2); | ||
} | ||
return wrap("dropDuplicates", { maintainOrder: opts.maintainOrder, subset }); | ||
return wrap("dropDuplicates", { maintainOrder, subset }); | ||
}, | ||
@@ -45,0 +44,0 @@ dropNulls(...subset) { |
@@ -912,4 +912,6 @@ import { DataType } from "../datatypes"; | ||
} | ||
export declare const Expr: (_expr: JsExpr) => Expr; | ||
export declare const Expr: ((_expr: JsExpr) => Expr) & { | ||
isExpr: (anyVal: any) => anyVal is Expr; | ||
}; | ||
export declare const exprToLitOrExpr: (expr: any, stringToLit?: boolean) => Expr; | ||
export {}; |
@@ -11,2 +11,3 @@ "use strict"; | ||
const utils_1 = require("../utils"); | ||
const types_1 = require("util/types"); | ||
const series_1 = require("../series"); | ||
@@ -130,3 +131,3 @@ const inspect = Symbol.for("nodejs.util.inspect.custom"); | ||
}; | ||
const Expr = (_expr) => { | ||
const _Expr = (_expr) => { | ||
const wrap = (method, args) => { | ||
@@ -322,3 +323,4 @@ return (0, exports.Expr)(polars_internal_1.default.expr[method]({ _expr, ...args })); | ||
}; | ||
exports.Expr = Expr; | ||
const isExpr = (anyVal) => (0, types_1.isExternal)(anyVal?._expr); | ||
exports.Expr = Object.assign(_Expr, { isExpr }); | ||
const exprToLitOrExpr = (expr, stringToLit = true) => { | ||
@@ -328,3 +330,3 @@ if (typeof expr === "string" && !stringToLit) { | ||
} | ||
else if ((0, utils_1.isExpr)(expr)) { | ||
else if (exports.Expr.isExpr(expr)) { | ||
return expr; | ||
@@ -331,0 +333,0 @@ } |
@@ -1296,6 +1296,9 @@ import { DataType, DtypeToPrimitive, Optional } from "./datatypes"; | ||
export declare const seriesWrapper: <T>(_s: JsSeries) => Series<T>; | ||
export declare function Series<V extends ArrayLike<any>>(values: V): ValueOrNever<V>; | ||
export declare function Series<V extends ArrayLike<any>>(name: string, values: V): ValueOrNever<V>; | ||
export declare function Series<T extends DataType, U extends ArrayLikeDataType<T>>(name: string, values: U, dtype: T): Series<DtypeToPrimitive<T>>; | ||
export declare function Series<T extends DataType, U extends boolean, V extends ArrayLikeOrDataType<T, U>>(name: string, values: V, dtype?: T, strict?: U): Series<DataTypeOrValue<T, U>>; | ||
declare function _Series<V extends ArrayLike<any>>(values: V): ValueOrNever<V>; | ||
declare function _Series<V extends ArrayLike<any>>(name: string, values: V): ValueOrNever<V>; | ||
declare function _Series<T extends DataType, U extends ArrayLikeDataType<T>>(name: string, values: U, dtype: T): Series<DtypeToPrimitive<T>>; | ||
declare function _Series<T extends DataType, U extends boolean, V extends ArrayLikeOrDataType<T, U>>(name: string, values: V, dtype?: T, strict?: U): Series<DataTypeOrValue<T, U>>; | ||
export declare const Series: typeof _Series & { | ||
isSeries: <T>(anyVal: any) => anyVal is Series<T>; | ||
}; | ||
export {}; |
@@ -15,4 +15,4 @@ "use strict"; | ||
const error_1 = require("./error"); | ||
const utils_1 = require("./utils"); | ||
const lazy_functions_1 = require("./lazy/lazy_functions"); | ||
const types_1 = require("util/types"); | ||
const inspect = Symbol.for("nodejs.util.inspect.custom"); | ||
@@ -243,3 +243,3 @@ const seriesWrapper = (_s) => { | ||
filter(predicate) { | ||
return (0, utils_1.isSeries)(predicate) ? | ||
return exports.Series.isSeries(predicate) ? | ||
wrap("filter", { filter: predicate._series }) : | ||
@@ -305,5 +305,5 @@ wrap("filter", { filter: predicate.predicate._series }); | ||
isIn(other) { | ||
return (0, utils_1.isSeries)(other) ? | ||
return exports.Series.isSeries(other) ? | ||
wrap("is_in", { other: other._series }) : | ||
wrap("is_in", { other: Series(other)._series }); | ||
wrap("is_in", { other: (0, exports.Series)(other)._series }); | ||
}, | ||
@@ -454,3 +454,3 @@ isInfinite() { | ||
} | ||
indices = (0, utils_1.isSeries)(indices) ? indices.cast(datatypes_1.DataType.UInt32).toArray() : indices; | ||
indices = exports.Series.isSeries(indices) ? indices.cast(datatypes_1.DataType.UInt32).toArray() : indices; | ||
unwrap(`set_at_idx_${dt}`, { indices, value }); | ||
@@ -525,6 +525,15 @@ return this; | ||
}, | ||
set: function (series, prop, input) { | ||
if (typeof prop !== "symbol" && !Number.isNaN(Number(prop))) { | ||
series.setAtIdx([Number(prop)], input); | ||
return true; | ||
} | ||
else { | ||
return Reflect.set(series, prop, input); | ||
} | ||
} | ||
}); | ||
}; | ||
exports.seriesWrapper = seriesWrapper; | ||
function Series(arg0, arg1, dtype, strict) { | ||
function _Series(arg0, arg1, dtype, strict) { | ||
if (typeof arg0 === "string") { | ||
@@ -534,4 +543,5 @@ const _s = (0, construction_1.arrayToJsSeries)(arg0, arg1, dtype, strict); | ||
} | ||
return Series("", arg0); | ||
return _Series("", arg0); | ||
} | ||
exports.Series = Series; | ||
const isSeries = (anyVal) => (0, types_1.isExternal)(anyVal?._series); | ||
exports.Series = Object.assign(_Series, { isSeries }); |
{ | ||
"name": "nodejs-polars", | ||
"version": "0.0.5", | ||
"version": "0.0.6", | ||
"repository": "https://github.com/pola-rs/polars.git", | ||
@@ -26,3 +26,2 @@ "license": "SEE LICENSE IN LICENSE", | ||
"aarch64-linux-android", | ||
"x86_64-unknown-freebsd", | ||
"x86_64-unknown-linux-musl", | ||
@@ -35,3 +34,3 @@ "aarch64-unknown-linux-musl", | ||
"engines": { | ||
"node": ">= 10" | ||
"node": ">= 10.20" | ||
}, | ||
@@ -45,6 +44,4 @@ "publishConfig": { | ||
"bench": "node -r @swc-node/register benchmark/bench.ts", | ||
"build": "napi build --platform --release artifacts/", | ||
"build:debug": "napi build --platform polars/", | ||
"build:all": "tsc -p tsconfig.build.json && napi build --platform --release bin/", | ||
"build:all:slim": "tsc -p tsconfig.build.json && RUSTFLAGS='-C codegen-units=16' napi build --platform --release bin/", | ||
"build": "napi build --platform --release polars", | ||
"build:debug": "napi build --platform", | ||
"build:ts": "tsc -p tsconfig.build.json", | ||
@@ -54,3 +51,3 @@ "format:rs": "cargo fmt", | ||
"format:yaml": "prettier --parser yaml --write './**/*.{yml,yaml}'", | ||
"lint:ts": "eslint -c ./.eslintrc.json 'polars/**/*.{ts,tsx,js}'", | ||
"lint:ts": "eslint -c ./.eslintrc.json 'polars/**/*.{ts,tsx,js}' '__tests__/*.ts'", | ||
"prepublishOnly": "napi prepublish -t npm", | ||
@@ -88,13 +85,6 @@ "test": "jest", | ||
"*.@(js|ts|tsx)": [ | ||
"prettier --write", | ||
"eslint -c .eslintrc.yml --fix" | ||
], | ||
"*.@(yml|yaml)": [ | ||
"*.@(js|ts|tsx|yml|yaml|md|json)": [ | ||
"prettier --parser yaml --write" | ||
], | ||
"*.md": [ | ||
"prettier --parser markdown --write" | ||
], | ||
"*.json": [ | ||
"prettier --parser json --write" | ||
] | ||
@@ -107,19 +97,17 @@ }, | ||
"singleQuote": true, | ||
"arrowParens": "always", | ||
"parser": "typescript" | ||
"arrowParens": "always" | ||
}, | ||
"optionalDependencies": { | ||
"nodejs-polars-win32-x64-msvc": "0.0.5", | ||
"nodejs-polars-darwin-x64": "0.0.5", | ||
"nodejs-polars-linux-x64-gnu": "0.0.5", | ||
"nodejs-polars-win32-ia32-msvc": "0.0.5", | ||
"nodejs-polars-linux-arm64-gnu": "0.0.5", | ||
"nodejs-polars-linux-arm-gnueabihf": "0.0.5", | ||
"nodejs-polars-darwin-arm64": "0.0.5", | ||
"nodejs-polars-android-arm64": "0.0.5", | ||
"nodejs-polars-freebsd-x64": "0.0.5", | ||
"nodejs-polars-linux-x64-musl": "0.0.5", | ||
"nodejs-polars-linux-arm64-musl": "0.0.5", | ||
"nodejs-polars-win32-arm64-msvc": "0.0.5" | ||
"nodejs-polars-win32-x64-msvc": "0.0.6", | ||
"nodejs-polars-darwin-x64": "0.0.6", | ||
"nodejs-polars-linux-x64-gnu": "0.0.6", | ||
"nodejs-polars-win32-ia32-msvc": "0.0.6", | ||
"nodejs-polars-linux-arm64-gnu": "0.0.6", | ||
"nodejs-polars-linux-arm-gnueabihf": "0.0.6", | ||
"nodejs-polars-darwin-arm64": "0.0.6", | ||
"nodejs-polars-android-arm64": "0.0.6", | ||
"nodejs-polars-linux-x64-musl": "0.0.6", | ||
"nodejs-polars-linux-arm64-musl": "0.0.6", | ||
"nodejs-polars-win32-arm64-msvc": "0.0.6" | ||
} | ||
} |
135
README.md
@@ -8,28 +8,63 @@ # Polars | ||
## Usage | ||
### Importing | ||
```js | ||
import pl from 'nodejs-polars'; | ||
const pl = require('nodejs-polars'); | ||
``` | ||
import pl from "polars" | ||
### Series | ||
const df = pl.DataFrame( | ||
{ | ||
A: [1, 2, 3, 4, 5], | ||
fruits: ["banana", "banana", "apple", "apple", "banana"], | ||
B: [5, 4, 3, 2, 1], | ||
cars: ["beetle", "audi", "beetle", "beetle", "beetle"], | ||
} | ||
) | ||
const df2 = df | ||
.sort("fruits") | ||
.select( | ||
"fruits", | ||
"cars", | ||
pl.lit("fruits").alias("literal_string_fruits"), | ||
pl.col("B").filter(pl.col("cars").eq(lit("beetle"))).sum(), | ||
pl.col("A").filter(pl.col("B").gt(2)).sum().over("cars").alias("sum_A_by_cars"), | ||
pl.col("A").sum().over("fruits").alias("sum_A_by_fruits"), | ||
pl.col("A").reverse().over("fruits").flatten().alias("rev_A_by_fruits") | ||
) | ||
console.log(df2) | ||
```js | ||
>>> const fooSeries = pl.Series("foo", [1, 2, 3]) | ||
>>> fooSeries.sum() | ||
6 | ||
// a lot operations support both positional and named arguments | ||
// you can see the full specs in the docs or the type definitions | ||
>>> fooSeries.sort(true) | ||
>>> fooSeries.sort({reverse: true}) | ||
shape: (3,) | ||
Series: 'foo' [f64] | ||
[ | ||
3 | ||
2 | ||
1 | ||
] | ||
>>> fooSeries.toArray() | ||
[1, 2, 3] | ||
// Series are 'Iterables' so you can use javascript iterable syntax on them | ||
>>> [...fooSeries] | ||
[1, 2, 3] | ||
>>> fooSeries[0] | ||
1 | ||
``` | ||
### DataFrame | ||
```js | ||
>>> const df = pl.DataFrame( | ||
... { | ||
... A: [1, 2, 3, 4, 5], | ||
... fruits: ["banana", "banana", "apple", "apple", "banana"], | ||
... B: [5, 4, 3, 2, 1], | ||
... cars: ["beetle", "audi", "beetle", "beetle", "beetle"], | ||
... } | ||
... ) | ||
>>> df | ||
... .sort("fruits") | ||
... .select( | ||
... "fruits", | ||
... "cars", | ||
... pl.lit("fruits").alias("literal_string_fruits"), | ||
... pl.col("B").filter(pl.col("cars").eq(lit("beetle"))).sum(), | ||
... pl.col("A").filter(pl.col("B").gt(2)).sum().over("cars").alias("sum_A_by_cars"), | ||
... pl.col("A").sum().over("fruits").alias("sum_A_by_fruits"), | ||
... pl.col("A").reverse().over("fruits").flatten().alias("rev_A_by_fruits") | ||
... ) | ||
shape: (5, 8) | ||
@@ -52,3 +87,15 @@ ┌──────────┬──────────┬──────────────┬─────┬─────────────┬─────────────┬─────────────┐ | ||
└──────────┴──────────┴──────────────┴─────┴─────────────┴─────────────┴─────────────┘ | ||
``` | ||
```js | ||
>>> df["cars"] // or df.getColumn("cars") | ||
shape: (5,) | ||
Series: 'cars' [str] | ||
[ | ||
"beetle" | ||
"beetle" | ||
"beetle" | ||
"audi" | ||
"beetle" | ||
] | ||
``` | ||
@@ -58,3 +105,2 @@ | ||
Install the latest polars version with: | ||
@@ -73,3 +119,2 @@ | ||
## Documentation | ||
@@ -81,14 +126,15 @@ | ||
* Installation guide: `$ pip3 install polars` | ||
* [Python documentation](https://pola-rs.github.io/polars/py-polars/html/reference/index.html) | ||
* [User guide](https://pola-rs.github.io/polars-book/) | ||
- Installation guide: `$ pip3 install polars` | ||
- [Python documentation](https://pola-rs.github.io/polars/py-polars/html/reference/index.html) | ||
- [User guide](https://pola-rs.github.io/polars-book/) | ||
#### Rust | ||
* [Rust documentation (master branch)](https://pola-rs.github.io/polars/polars/index.html) | ||
* [User guide](https://pola-rs.github.io/polars-book/) | ||
- [Rust documentation (master branch)](https://pola-rs.github.io/polars/polars/index.html) | ||
- [User guide](https://pola-rs.github.io/polars-book/) | ||
### Node | ||
* COMING SOON! | ||
- **COMING SOON!** | ||
## Contribution | ||
@@ -101,18 +147,15 @@ | ||
If you want a bleeding edge release or maximal performance you should compile **polars** from source. | ||
1. Install the latest [Rust compiler](https://www.rust-lang.org/tools/install) | ||
2. Run `npm|yarn install` | ||
3. Choose any of: | ||
* Fastest binary, very long compile times: | ||
```bash | ||
$ cd nodejs-polars && yarn build:all # this will generate a /bin directory with the compiles TS code, as well as the rust binary | ||
``` | ||
* Fast binary, Shorter compile times: | ||
```bash | ||
$ cd nodejs-polars && yarn build:all:slim # this will generate a /bin directory with the compiles TS code, as well as the rust binary | ||
``` | ||
### Debugging | ||
for an unoptimized build, you can run `yarn build:debug` | ||
1. Install the latest [Rust compiler](https://www.rust-lang.org/tools/install) | ||
2. Run `npm|yarn install` | ||
3. Choose any of: | ||
- Fastest binary, very long compile times: | ||
```bash | ||
$ cd nodejs-polars && yarn build && yarn build:ts # this will generate a /bin directory with the compiles TS code, as well as the rust binary | ||
``` | ||
- Debugging, fastest compile times but slow & large binary: | ||
```bash | ||
$ cd nodejs-polars && yarn build:debug && yarn build:ts # this will generate a /bin directory with the compiles TS code, as well as the rust binary | ||
``` | ||
## Acknowledgements | ||
@@ -122,8 +165,6 @@ | ||
[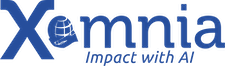](https://www.xomnia.com/) | ||
## Sponsors | ||
[<img src="https://raw.githubusercontent.com/pola-rs/polars-static/master/sponsors/xomnia.png" height="40" />](https://www.xomnia.com/)   [<img src="https://www.jetbrains.com/company/brand/img/jetbrains_logo.png" height="50" />](https://www.jetbrains.com) | ||
[<img src="https://raw.githubusercontent.com/pola-rs/polars-static/master/sponsors/xomnia.png" height="40" />](https://www.xomnia.com/)   [<img src="https://www.jetbrains.com/company/brand/img/jetbrains_logo.png" height="50" />](https://www.jetbrains.com) |
285802
12
7645
164