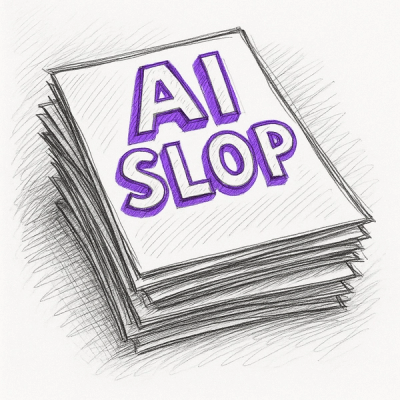
Security News
Django Joins curl in Pushing Back on AI Slop Security Reports
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
NorkHash is a simple HashMap implementation for node.js.
It was built for Norker but broken out - because, well it's self contained. And why not? You probably won't need it since there are a lot of other HashMap implementations (a lot of them better than this) but - it's still usable.
NorkHash is unit tested, not application tested and should be considered experimental. Use at your own risk.
npm install nork-hash
var Hash = require(NorkHash);
var hash = new Hash();
hash.put('one', {val:"one"});
console.log(hash.get('one'));
console.log('forEach')
hash.puts(['two', 'three'],[{val:'two'},{val:'three'}])
hash.forEach(function(key, val){
console.log(key);
console.log(val);
});
Returns int
.
Size of hash.
Returns self
.
Inserts new key/value pair.
Returns Whatever you put in it
key
Get value from key
.
Returns Whatever you put in it
at key
.
Get and delete value at key
.
Returns self
Delete value at key
Returns self
.
Removes all key/value pairs of hash.
Returns Boolean
Return true if hash contains key
Returns Array
.
Of all values stored in hash.
Returns Array
Of all keys stored in hash
Returns self
.
For each key/value pair in the hash, calls fun(key, value)
.
This call can have side effects. this
will be bound to fun()
.
Returns a new Hash
.
The return value of fun(key, value)
is the new value of key
in the new Hash.
Returns a new Hash
.
The return value of fun(key, value)
determines if the key/value pair should be contained in the new Hash.
Returns Boolean
True if fun(key, value)
evaluates to truthy for one or more key/value pairs.
Returns Boolean
True if fun(key, value)
evalutes to truthy for all key/value pairs
Functions that takes a key param now throws a TypeError if the key is not a string or ''
All array methods are removed. Map doesn't loop two times any longer. put doesn't react to put(undefined).
Added pop(key)
, pops([keys])
, clear()
and unit test for theese.
First commit, working code.
FAQs
Simple hash map implementation with map, filter, each, every and some.
The npm package nork-hash receives a total of 3 weekly downloads. As such, nork-hash popularity was classified as not popular.
We found that nork-hash demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.