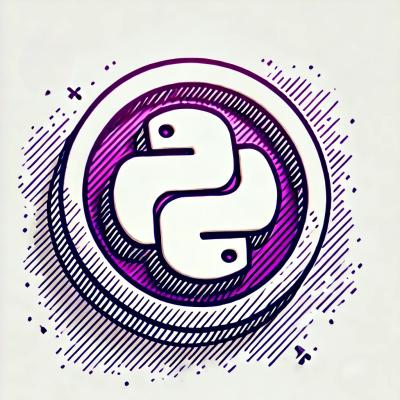
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
notifications-node-client
Advanced tools
The notifications-node-client is an npm package designed to interact with the GOV.UK Notify service, which allows you to send emails, text messages, and letters. This package provides a simple interface to integrate these notification services into your Node.js applications.
Send Email
This feature allows you to send an email using a predefined template. You need to provide the template ID, recipient's email address, and any personalisation fields required by the template.
const NotifyClient = require('notifications-node-client').NotifyClient;
const notifyClient = new NotifyClient('your-api-key');
notifyClient.sendEmail('template-id', 'recipient@example.com', {
personalisation: {
name: 'John Doe',
reference: '12345'
},
reference: 'your-reference'
}).then(response => console.log(response)).catch(err => console.error(err));
Send SMS
This feature allows you to send an SMS using a predefined template. You need to provide the template ID, recipient's phone number, and any personalisation fields required by the template.
const NotifyClient = require('notifications-node-client').NotifyClient;
const notifyClient = new NotifyClient('your-api-key');
notifyClient.sendSms('template-id', '07123456789', {
personalisation: {
name: 'John Doe',
reference: '12345'
},
reference: 'your-reference'
}).then(response => console.log(response)).catch(err => console.error(err));
Send Letter
This feature allows you to send a letter using a predefined template. You need to provide the template ID and the recipient's address details.
const NotifyClient = require('notifications-node-client').NotifyClient;
const notifyClient = new NotifyClient('your-api-key');
notifyClient.sendLetter('template-id', {
personalisation: {
address_line_1: 'John Doe',
address_line_2: '123 Main Street',
postcode: 'AB1 2CD'
},
reference: 'your-reference'
}).then(response => console.log(response)).catch(err => console.error(err));
Nodemailer is a module for Node.js applications to allow easy email sending. Unlike notifications-node-client, which is specific to the GOV.UK Notify service, Nodemailer is a more general-purpose email sending library that supports various transport methods including SMTP, AWS SES, and more.
Twilio is a comprehensive communication API that allows you to send SMS, make voice calls, and perform other communication tasks. It is more versatile compared to notifications-node-client, which is focused on the GOV.UK Notify service. Twilio supports a wide range of communication channels and is used globally.
SendGrid is a cloud-based service that provides email delivery and marketing campaigns. It offers a robust API for sending emails, managing lists, and tracking email performance. Unlike notifications-node-client, which is limited to the GOV.UK Notify service, SendGrid provides a broader range of email-related functionalities.
Use this client to send emails, text messages and letters using the GOV.UK Notify API.
Useful links:
8.2.1 - 2024-07-03
FAQs
GOV.UK Notify Node.js client
The npm package notifications-node-client receives a total of 4,961,743 weekly downloads. As such, notifications-node-client popularity was classified as popular.
We found that notifications-node-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.