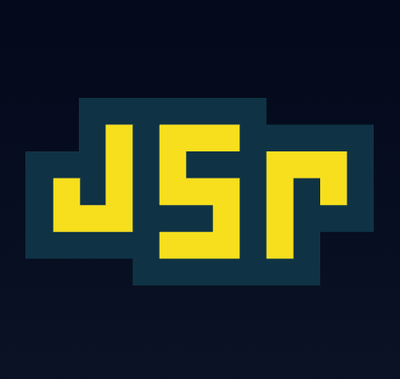
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
npm-packlist
Advanced tools
The npm-packlist package is used to generate a list of files that would be included in an npm package publish. This includes filtering out files that are not meant to be published, such as those specified in `.npmignore` or the `.gitignore` files, and including those specified in the `files` array in `package.json`. It's useful for package authors to understand and control what gets included in their published npm package.
Generate packlist
This feature allows you to generate a list of files that would be included if you were to publish the current package. The code sample demonstrates how to use npm-packlist to get an array of file paths that are included in the package's publish list.
const packlist = require('npm-packlist');
packlist().then(files => {
console.log(files)
});
Customize packlist with package.json
While not a direct feature of npm-packlist, the package respects the `files` field in `package.json`. This allows users to explicitly specify which files or directories should be included in the publish. The code sample shows how to specify a list of files and directories in `package.json` that should be included in the npm package.
{
"files": [
"lib/**/*",
"bin/*",
"README.md"
]
}
The 'glob' package provides functionality to match files using the patterns the shell uses, like stars and stuff. It's similar to npm-packlist in that it can be used to select files to include in a package, but it does not directly integrate with npm's packaging logic or respect `.npmignore` and `files` in `package.json`.
The 'ignore' package is used to filter file paths according to the rules found in `.gitignore` and `.npmignore` files. It's similar to npm-packlist in its purpose of filtering out files, but it's more focused on the ignore rules and doesn't directly generate a list of files for npm packaging.
Get a list of the files to add from a folder into an npm package
These can be handed to tar like so to make an npm package tarball:
const pack = require('npm-packlist')
const tar = require('tar')
const packageDir = '/path/to/package'
const packageTarball = '/path/to/package.tgz'
pack({ path: packageDir })
.then(files => tar.create({
prefix: 'package/',
cwd: packageDir,
file: packageTarball,
gzip: true,
bundled: [
'some',
'deps',
'that-are',
'bundled-dependencies-in-node_modules',
'this-is-optional-of-course'
]
}, files))
.then(_ => {
// tarball has been created, continue with your day
})
This uses the following rules:
If a package.json
file is found, and it has a files
list,
then ignore everything that isn't in files
. Always include the
readme, license, notice, changes, changelog, and history files, if
they exist, and the package.json file itself.
If there's no package.json
file (or it has no files
list), and
there is a .npmignore
file, then ignore all the files in the
.npmignore
file.
If there's no package.json
with a files
list, and there's no
.npmignore
file, but there is a .gitignore
file, then ignore
all the files in the .gitignore
file.
Everything in the root node_modules
is ignored, unless it's a
bundled dependency. If it IS a bundled dependency, and it's a
symbolic link, then the target of the link is included, not the
symlink itself. (The bundled
option determines which packages
are to be considered bundled deps.)
Unless they're explicitly included (by being in a files
list, or
a !negated
rule in a relevant .npmignore
or .gitignore
),
always ignore certain common cruft files:
.*.swp
, ._*
and .*.orig
files/test/
or /tests/
folder at the root.npmrc
files (these may contain private configs)node_modules/.bin
folder/build/config.gypi
and .lock-wscript
.DS_Store
files because wtf are those evennpm-debug.log
files at the root of a projectYou can explicitly re-include any of these with a files
list in
package.json
or a negated ignore file rule.
Same API as ignore-walk, just hard-coded
file list and rule sets, and takes the bundled
list of package names
to include.
FAQs
Get a list of the files to add from a folder into an npm package
We found that npm-packlist demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.