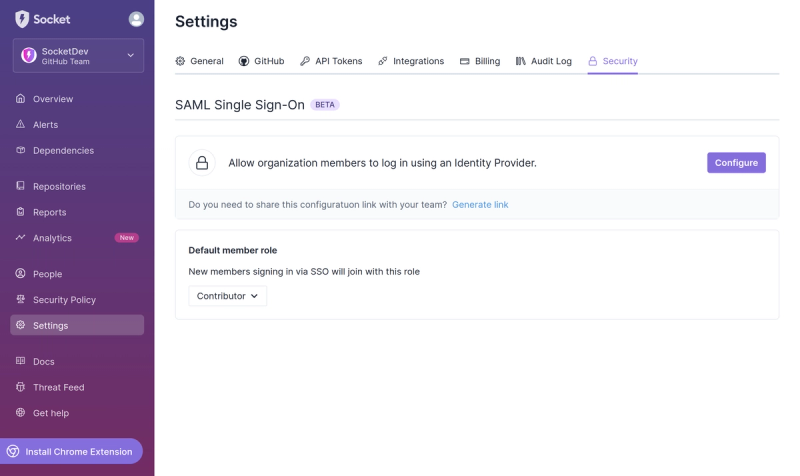
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
numcodecs
Advanced tools
Changelog
Readme
Buffer compression and transformation codecs for use in Zarr.js and beyond...
npm install numcodecs
import { Blosc, GZip, Zlib, LZ4, Zstd } from 'numcodecs';
const codec = new Blosc();
// or Blosc.fromConfig({ clevel: 5, cname: 'lz4', shuffle: Blosc.SHUFFLE, blocksize: 0 });
const size = 100000;
const arr = new Uint32Array(size);
for (let i = 0; i < size; i++) {
arr[i] = i;
}
const bytes = new Uint8Array(arr.buffer);
console.log(bytes);
// Uint8Array(400000) [0, 0, 0, 0, 1, 0, 0, 0, 2, 0, 0, 0, ... ]
const encoded = await codec.encode(bytes);
console.log(encoded);
// Uint8Array(3744) [2, 1, 33, 4, 128, 26, 6, 0, 0, 0, 4, 0, ... ]
const decoded = await codec.decode(encoded);
console.log(new Uint32Array(decoded.buffer));
// Uint32Array(100000) [ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, ... ]
This project is an incomplete TypeScript implementation of the buffer compression library
numcodecs
. The following codecs
are currently supported:
blosc
gzip
lz4
zlib
zstd
Each compressor is bundled as the default export of separate code-split submodules. This makes it easy to import each module independently in your applications or from a ESM-friendly CDN like skypack.
// Main entry point (exports all codecs)
import { Zlib } from 'numcodecs';
// Submodule entry point (exports only `zlib`)
import Zlib from 'numcodecs/zlib';
// Main entry point (exports all codecs)
import { Zlib } from 'https://cdn.skypack.dev/numcodecs';
// Submodule entry point (exports only `zlib`)
import Zlib from 'https://cdn.skypack.dev/numcodecs/zlib';
$ git clone https://github.com/manzt/numcodecs.js.git
$ cd numcodecs.js
$ npm install && npm run test
The <codec_name>.js
+ <codec_name>.wasm
source for each WASM-based codec are
generated with Docker with the following commands:
cd codecs/<codec_name>
npm run build
For changes to be reflected in package changelogs, run npx changeset
and
follow the prompts.
Note not every PR requires a changeset. Since changesets are focused on releases and changelogs, changes to the repository that don't effect these won't need a changeset (e.g., documentation, tests).
The Changesets GitHub action will create and update a PR that applies changesets and publishes new versions.
FAQs
Buffer compression and transformation codecs for use in data storage and communication applications.
The npm package numcodecs receives a total of 832 weekly downloads. As such, numcodecs popularity was classified as not popular.
We found that numcodecs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.