nuxt-cookie-consent
Advanced tools
Comparing version 0.0.1 to 0.1.1
@@ -12,3 +12,3 @@ const path = require('path') | ||
src: path.resolve(__dirname, 'plugin.js'), | ||
fileName: 'nuxt-cookie-control.js', | ||
fileName: 'nuxt-cookie-consent.js', | ||
options | ||
@@ -15,0 +15,0 @@ }) |
@@ -6,114 +6,112 @@ import Vue from 'vue' | ||
// todo handle expirationDate | ||
// noinspection JSAnnotator | ||
const options = <%= serialize(options) %> | ||
const cookieNames = { | ||
consentStatusByGroupName: 'cookie_consent_status', | ||
} | ||
const CookieUniversal = makeCookieUniversal(req, res) | ||
let cookieConsentObject = { | ||
_showConsentOptions: false, | ||
get showConsentOptions() { | ||
return this._showConsentOptions | ||
// properties derived from cookie values | ||
hasUserMadeChoice: null, | ||
consentStatus: null, | ||
updateCookieProperties() { | ||
this.hasUserMadeChoice = !!CookieUniversal.get(cookieNames.consentStatusByGroupName) | ||
this.consentStatus = CookieUniversal.get(cookieNames.consentStatusByGroupName) || {} | ||
}, | ||
set showConsentOptions(show) { | ||
this._showConsentOptions = show | ||
// data properties | ||
showConsentOptions: false, | ||
// getters | ||
get hasUserAcceptedAllGroups() { | ||
return Object.keys(this.config.groups).every(groupId => !!this.consentStatus[groupId]) | ||
}, | ||
hasUserMadeChoice: false, | ||
get hasUserAcceptedAllCookies() { | ||
// todo: implement | ||
return false | ||
} | ||
scripts: [], | ||
enabled: [], | ||
enabledList: [], | ||
optional: [], | ||
openConsentOptions() { | ||
this.showConfigurationOptions = true | ||
get isConsentComplete() { | ||
return Object.keys(this.config.groups).every(groupId => groupId in this.consentStatus) | ||
}, | ||
closeConsentOptions() { | ||
this.showConfigurationOptions = false | ||
get config() { | ||
return options | ||
}, | ||
get(name, options) { | ||
return CookieUniversal.get(name, options) | ||
// functions | ||
init() { | ||
// read data from cookies | ||
this.updateCookieProperties() | ||
// call all onAccept handlers | ||
Object.keys(this.consentStatus) | ||
.filter(groupId => this.config.groups[groupId] && this.config.groups[groupId].onInit) | ||
.forEach(groupId => this.config.groups[groupId].onInit(this.consentStatus[groupId])) | ||
}, | ||
set(name, value, options) { | ||
return CookieUniversal.get(name, value, options) | ||
acceptGroup(groupId) { | ||
this._assertGroupExists(groupId) | ||
if (this.consentStatus[groupId] === true) { | ||
return | ||
} | ||
CookieUniversal.set( | ||
cookieNames.consentStatusByGroupName, | ||
{ | ||
...this.consentStatus, | ||
[groupId]: true, | ||
} | ||
) | ||
this.updateCookieProperties() | ||
this.config.groups[groupId].onAccept && this.config.groups[groupId].onAccept(true) | ||
}, | ||
} | ||
declineGroup(groupId) { | ||
this._assertGroupExists(groupId) | ||
if (this.consentStatus[groupId] === false) { | ||
return | ||
} | ||
CookieUniversal.set( | ||
cookieNames.consentStatusByGroupName, | ||
{ | ||
...this.consentStatus, | ||
[groupId]: false, | ||
} | ||
) | ||
this.updateCookieProperties() | ||
this.config.groups[groupId].onDecline && this.config.groups[groupId].onDecline(true) | ||
}, | ||
acceptAll() { | ||
Object.keys(this.config.groups).forEach(groupId => this.acceptGroup(groupId)) | ||
}, | ||
declineAll() { | ||
Object.keys(this.config.groups).forEach(groupId => this.declineGroup(groupId)) | ||
}, | ||
clearAllData() { | ||
CookieUniversal.remove(cookieNames.consentStatusByGroupName) | ||
this.updateCookieProperties() | ||
}, | ||
getGroupIdByCookieName(cookieName) { | ||
return Object.keys(this.config.groups).find(groupId => this.config.groups[groupId].cookies.includes(cookieName)) | ||
}, | ||
// isEnabled: (identifier) => { | ||
// return cookieConsentObject.enabledList.includes(identifier) | ||
// }, | ||
// | ||
// remove: (name) =>{ | ||
// if(process.browser){ | ||
// let domain = window.location.hostname | ||
// CookieUniversal.set({name, expires: 'Thu, 01 Jan 1970 00:00:00 GMT', domain }) | ||
// for (let j = domain.split('.'); j.length;) { | ||
// let o = j.join('.') | ||
// CookieUniversal.set({name, expires: 'Thu, 01 Jan 1970 00:00:00 GMT', domain: `.${o}` }) | ||
// j.shift() | ||
// } | ||
// } | ||
// } | ||
// | ||
// | ||
// const clearCookies = () =>{ | ||
// let disabled = cookieConsentObject.optional.filter(cookie => { | ||
// let cookieName = typeof(cookie.name) === 'string' ? cookie.name : cookie.name[Object.keys(cookie.name)[0]] | ||
// return !cookieConsentObject.enabledList.includes(cookie.identifier) | ||
// }) | ||
// if(disabled.length > 0){ | ||
// disabled.forEach(cookie => { | ||
// if(cookie.declined) cookie.declined() | ||
// if(cookie.cookieConsentObject && cookie.cookieConsentObject.length > 0){ | ||
// cookie.cookieConsentObject.forEach(i => { | ||
// cookieConsentObject.remove(i) | ||
// }) | ||
// } | ||
// }) | ||
// } | ||
// } | ||
// | ||
// const setHead = () =>{ | ||
// if(cookieConsentObject.enabled.length > 0){ | ||
// let head = document.getElementsByTagName('head')[0] | ||
// cookieConsentObject.enabled.forEach(cookie =>{ | ||
// if(cookie.src){ | ||
// let script = document.createElement('script') | ||
// script.src = cookie.src | ||
// head.appendChild(script) | ||
// script.addEventListener('load', () =>{ | ||
// if(cookie.accepted) cookie.accepted() | ||
// }) | ||
// } | ||
// }) | ||
// } | ||
// } | ||
// | ||
// const callAcceptedFunctions = () =>{ | ||
// if(cookieConsentObject.enabled.length > 0){ | ||
// cookieConsentObject.enabled.forEach(cookie =>{ | ||
// if(cookie.accepted && !cookie.src) cookie.accepted() | ||
// }) | ||
// } | ||
// } | ||
// decorator for cookie-universal | ||
get(cookieName, options) { | ||
return CookieUniversal.get(cookieName, options) | ||
}, | ||
set(cookieName, value, options) { | ||
if (!this.consentStatus[this.getGroupIdByCookieName(cookieName)]) { | ||
throw new Error(`Cookie ${cookieName} has not been given consent`) | ||
} | ||
return CookieUniversal.get(cookieName, value, options) | ||
}, | ||
_assertGroupExists(groupId) { | ||
if (!(groupId in this.config.groups)) { | ||
throw new Error(`group ${groupId} is not configured`) | ||
} | ||
} | ||
// cookieConsentObject.hasUserMadeChoice = CookieUniversal.get('cookie_consent_given') === 'true' ? true : false | ||
// | ||
// if(cookieConsentObject.hasUserMadeChoice === true){ | ||
// let enabledFromCookie = CookieUniversal.get('cookie_consent_enabled_groups') | ||
// cookieConsentObject.enabled.push(...cookieConsentObject.optional.filter(cookie => { | ||
// let cookieName = typeof(cookie.name) === 'string' ? cookie.name : cookie.name[Object.keys(cookie.name)[ | ||
// return enabledFromCookie.includes(cookie.identifier) | ||
// })) | ||
// cookieConsentObject.enabledList = cookieConsentObject.enabled.length > 0 ? cookieConsentObject.enabled.map(cookie => { | ||
// let cookieName = typeof(cookie.name) === 'string' ? cookie.name : cookie.name[Object.keys(cookie.name)[0]] | ||
// return cookie.identifier | ||
// }) : [] | ||
// } | ||
// | ||
// if(cookieConsentObject.necessary) cookieConsentObject.enabled.push(...cookieConsentObject.necessary.filter(cookie => {return cookie.src || cookie.accepted})) | ||
// | ||
} | ||
const options = <%= serialize(options) %> | ||
// read cookies | ||
cookieConsentObject.init() | ||
Object.assign(cookieConsentObject, options) | ||
// make observable | ||
@@ -120,0 +118,0 @@ cookieConsentObject = Vue.observable(cookieConsentObject) |
{ | ||
"name": "nuxt-cookie-consent", | ||
"version": "0.0.1", | ||
"description": "Renderless GDPR compliant Nuxt cookie consent library", | ||
"version": "0.1.1", | ||
"description": "Renderless Nuxt cookie consent library to stay GDPR compliant", | ||
"license": "MIT", | ||
"author": "Aimo Künkel", | ||
"contributors": [ | ||
"Dario Ferderber", | ||
"Davor Teskera" | ||
], | ||
"contributors": [], | ||
"keywords": [ | ||
@@ -43,5 +40,4 @@ "nuxt cookie consent", | ||
"devDependencies": {}, | ||
"scripts": { | ||
"test": "echo \"Error: no test specified\" && exit 1" | ||
} | ||
"scripts": {}, | ||
"typings": "types/index.d.ts" | ||
} |
@@ -8,3 +8,3 @@ 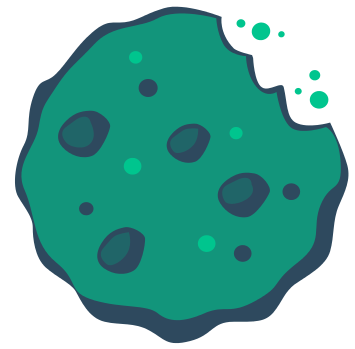 | ||
```bash | ||
npm i nuxt-cookie-control | ||
npm i nuxt-cookie-consent | ||
``` | ||
@@ -18,7 +18,7 @@ [![npm version][npm-version-src]][npm-version-href] | ||
modules: [ | ||
'nuxt-cookie-control' | ||
'nuxt-cookie-consent' | ||
] | ||
//or | ||
modules: [ | ||
['nuxt-cookie-control', { | ||
['nuxt-cookie-consent', { | ||
//your options | ||
@@ -90,6 +90,6 @@ }] | ||
modules: [ | ||
['nuxt-cookie-control', { | ||
['nuxt-cookie-consent', { | ||
//default css (true) | ||
//if css is set to false, you will still be able to access | ||
//your color variables (example. background-color: var(--cookie-control-barBackground)) | ||
//your color variables (example. background-color: var(--cookie-consent-barBackground)) | ||
css: true, | ||
@@ -170,3 +170,3 @@ | ||
modules: [ | ||
'nuxt-cookie-control' | ||
'nuxt-cookie-consent' | ||
] | ||
@@ -177,3 +177,3 @@ ``` | ||
modules: [ | ||
'nuxt-cookie-control' | ||
'nuxt-cookie-consent' | ||
] | ||
@@ -270,4 +270,4 @@ ... | ||
<!-- Badges --> | ||
[npm-version-src]: https://badgen.net/npm/v/nuxt-cookie-control/latest | ||
[npm-version-href]: https://npmjs.com/package/nuxt-cookie-control | ||
[npm-version-src]: https://badgen.net/npm/v/nuxt-cookie-consent/latest | ||
[npm-version-href]: https://npmjs.com/package/nuxt-cookie-consent | ||
@@ -277,3 +277,3 @@ [kofi-src]: https://badgen.net/badge/icon/kofi?icon=kofi&label=support | ||
[npm-downloads-src]: https://badgen.net/npm/dm/nuxt-cookie-control | ||
[npm-downloads-href]: https://npmjs.com/package/nuxt-cookie-control | ||
[npm-downloads-src]: https://badgen.net/npm/dm/nuxt-cookie-consent | ||
[npm-downloads-href]: https://npmjs.com/package/nuxt-cookie-consent |
@@ -5,4 +5,22 @@ // augment typings of Vue.js | ||
export interface ConsentStatus { | ||
[key: string]: true|false|undefined | ||
} | ||
export interface CookieConsent { | ||
hasUserMadeChoice: boolean | ||
hasUserMadeChoice: boolean, | ||
readonly consentStatus: ConsentStatus, | ||
updateCookieProperties: () => null | ||
showConsentOptions: boolean, | ||
readonly hasUserAcceptedAllGroups: boolean | ||
readonly isConsentComplete: boolean | ||
readonly config: any | ||
acceptGroup: (groupId: string) => null | ||
declineGroup: (groupId: string) => null | ||
acceptAll: () => null | ||
declineAll: () => null | ||
clearAllData: () => null | ||
getGroupIdByCookieName: (cookieName: string) => string | ||
// decorators | ||
get: NuxtCookies['get'] | ||
@@ -9,0 +27,0 @@ set: NuxtCookies['set'] |
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
No tests
QualityPackage does not have any tests. This is a strong signal of a poorly maintained or low quality package.
Found 1 instance in 1 package
165
1
273
12366
1