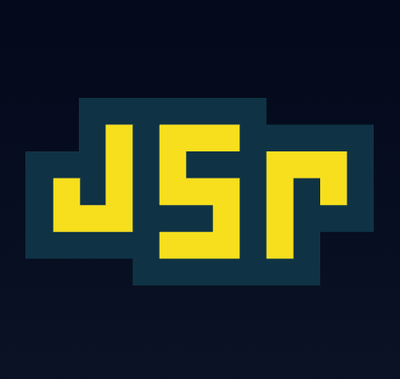
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
object-path
Advanced tools
The object-path npm package provides utilities for accessing and manipulating deep properties of objects using a string path notation. It simplifies the process of getting, setting, and deleting nested properties in JavaScript objects.
Get a nested property
This feature allows you to retrieve the value of a deeply nested property within an object using a string path.
const objectPath = require('object-path');
const obj = { a: { b: { c: 42 } } };
const value = objectPath.get(obj, 'a.b.c');
console.log(value); // 42
Set a nested property
This feature allows you to set the value of a deeply nested property within an object using a string path.
const objectPath = require('object-path');
const obj = { a: { b: { } } };
objectPath.set(obj, 'a.b.c', 42);
console.log(obj); // { a: { b: { c: 42 } } }
Delete a nested property
This feature allows you to delete a deeply nested property within an object using a string path.
const objectPath = require('object-path');
const obj = { a: { b: { c: 42 } } };
objectPath.del(obj, 'a.b.c');
console.log(obj); // { a: { b: { } } }
Check if a nested property exists
This feature allows you to check if a deeply nested property exists within an object using a string path.
const objectPath = require('object-path');
const obj = { a: { b: { c: 42 } } };
const exists = objectPath.has(obj, 'a.b.c');
console.log(exists); // true
Lodash is a popular utility library that provides a wide range of functions for manipulating arrays, objects, and other data types. It includes methods like _.get, _.set, and _.has for working with nested properties, similar to object-path. However, Lodash offers a broader set of utilities beyond just object manipulation.
Dot-prop is a lightweight package that provides similar functionality to object-path for getting, setting, and deleting nested properties using dot notation. It is simpler and more focused on object property manipulation compared to the more feature-rich Lodash.
Deepdash is an extension of Lodash that adds deep traversal and manipulation capabilities. It provides methods for working with deeply nested properties, similar to object-path, but with additional features for deep operations on arrays and objects.
Access deep properties using a path
op.withInheritedProps.set({}, [['__proto__'], 'polluted'], true)
)set()
function when using the "inherited props" mode (e.g. when a new object-path
instance is created with the includeInheritedProps
option set to true
or when using the withInheritedProps
default instance. The vulnerability does not exist in the default instance exposed by object path (e.g objectPath.set()
) if using version >= 0.11.0
.object-path
object-path
deals with inherited properties (includeInheritedProps
)object-path
instance already configured to handle not-own object properties (withInheritedProps
)get
, set
, and push
by 2x-3xdel
, empty
, set
will not affect not-own object's properties (made them consistent with the other methods)npm install object-path --save
bower install object-path --save
typings install --save dt~object-path
var obj = {
a: {
b: "d",
c: ["e", "f"],
'\u1200': 'unicode key',
'dot.dot': 'key'
}
};
var objectPath = require("object-path");
//get deep property
objectPath.get(obj, "a.b"); //returns "d"
objectPath.get(obj, ["a", "dot.dot"]); //returns "key"
objectPath.get(obj, 'a.\u1200'); //returns "unicode key"
//get the first non-undefined value
objectPath.coalesce(obj, ['a.z', 'a.d', ['a','b']], 'default');
//empty a given path (but do not delete it) depending on their type,so it retains reference to objects and arrays.
//functions that are not inherited from prototype are set to null.
//object instances are considered objects and just own property names are deleted
objectPath.empty(obj, 'a.b'); // obj.a.b is now ''
objectPath.empty(obj, 'a.c'); // obj.a.c is now []
objectPath.empty(obj, 'a'); // obj.a is now {}
//works also with arrays
objectPath.get(obj, "a.c.1"); //returns "f"
objectPath.get(obj, ["a","c","1"]); //returns "f"
//can return a default value with get
objectPath.get(obj, ["a.c.b"], "DEFAULT"); //returns "DEFAULT", since a.c.b path doesn't exists, if omitted, returns undefined
//set
objectPath.set(obj, "a.h", "m"); // or objectPath.set(obj, ["a","h"], "m");
objectPath.get(obj, "a.h"); //returns "m"
//set will create intermediate object/arrays
objectPath.set(obj, "a.j.0.f", "m");
//will insert values in array
objectPath.insert(obj, "a.c", "m", 1); // obj.a.c = ["e", "m", "f"]
//push into arrays (and create intermediate objects/arrays)
objectPath.push(obj, "a.k", "o");
//ensure a path exists (if it doesn't, set the default value you provide)
objectPath.ensureExists(obj, "a.k.1", "DEFAULT");
var oldVal = objectPath.ensureExists(obj, "a.b", "DEFAULT"); // oldval === "d"
//deletes a path
objectPath.del(obj, "a.b"); // obj.a.b is now undefined
objectPath.del(obj, ["a","c",0]); // obj.a.c is now ['f']
//tests path existence
objectPath.has(obj, "a.b"); // true
objectPath.has(obj, ["a","d"]); // false
//bind object
var model = objectPath({
a: {
b: "d",
c: ["e", "f"]
}
});
//now any method from above is supported directly w/o passing an object
model.get("a.b"); //returns "d"
model.get(["a.c.b"], "DEFAULT"); //returns "DEFAULT"
model.del("a.b"); // obj.a.b is now undefined
model.has("a.b"); // false
object-path
deals with inherited propertiesBy default object-path
will only access an object's own properties. Look at the following example:
var proto = {
notOwn: {prop: 'a'}
}
var obj = Object.create(proto);
//This will return undefined (or the default value you specified), because notOwn is
//an inherited property
objectPath.get(obj, 'notOwn.prop');
//This will set the property on the obj instance and not the prototype.
//In other words proto.notOwn.prop === 'a' and obj.notOwn.prop === 'b'
objectPath.set(obj, 'notOwn.prop', 'b');
To configure object-path
to also deal with inherited properties, you need to create a new instance and specify
the includeInheritedProps = true
in the options object:
var objectPath = require("object-path");
var objectPathWithInheritedProps = objectPath.create({includeInheritedProps: true})
Alternatively, object-path
exposes an instance already configured to handle inherited properties (objectPath.withInheritedProps
):
var objectPath = require("object-path");
var objectPathWithInheritedProps = objectPath.withInheritedProps
Once you have the new instance, you can access inherited properties as you access other properties:
var proto = {
notOwn: {prop: 'a'}
}
var obj = Object.create(proto);
//This will return 'a'
objectPath.withInheritedProps.get(obj, 'notOwn.prop');
//This will set proto.notOwn.prop to 'b'
objectPath.set(obj, 'notOwn.prop', 'b');
If you are looking for an immutable alternative of this library, you can take a look at: object-path-immutable
FAQs
Access deep object properties using a path
The npm package object-path receives a total of 1,727,355 weekly downloads. As such, object-path popularity was classified as popular.
We found that object-path demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.