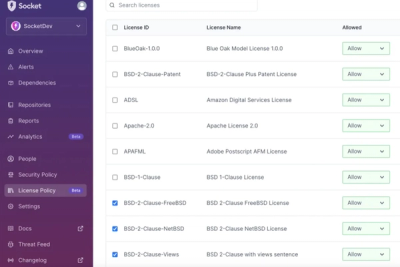
Product
Introducing License Enforcement in Socket
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
ocr_14_library
Advanced tools
This repository contains the code for a library of react components. The components are published in TypeScript
the front-end developer: Lucie
Install this package:
npm i ocr_14_library
Import the Counter component:
import { Modal } from "ocr_14_library";
You can then render the Modal
component like any other React component in JSX.
It's an easy way to add a modal box to your project To use this component you need to create a boolean state
import { Modal } from "ocr_14_library"
import { useState } from "react";
export default function Component() {
const [openModal, setOpenModal] = useState(false);
return(
<button onClick={() => setOpenModal(true)}/>
<Modal
open={openModal}
onClose={() => setOpenModal(false)}
HtmlElement={document.getElementById("portal")}
> My modal text </Modal>
)
}
This components needs four params :
Fork this repository to GitHub
Add your TypeScript components in src/ folder
Export your component in src/index.ts file :
export { MyNewComponent } from "./MyNewComponent";
Modify the information in package.json
Run build scripts :
npm run build
And publish dist folder on npm :
npm publish ./dist
FAQs
A component library
The npm package ocr_14_library receives a total of 0 weekly downloads. As such, ocr_14_library popularity was classified as not popular.
We found that ocr_14_library demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.