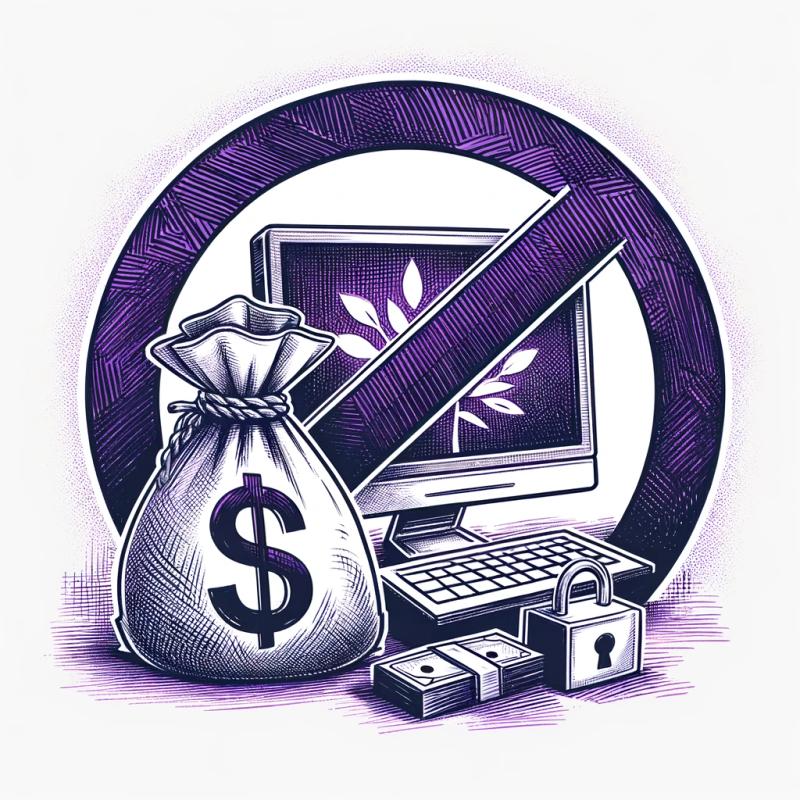
Security News
The Push to Ban Ransom Payments Is Gaining Momentum
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
odesza
Advanced tools
Readme
Odesza is a templating engine that allows you to write clean, expressive templates with inline JavaScript. Think of it as JS template strings, but for anything.
Take inheritance, partials, and inline JS ideas from Jade and strip away the "magic" and shorthand HTML syntax.
Variables are passed in when Odesza templates are rendered. Scope is maintained through includes and extends. You can also treat ${}
as a function statement.
hello.ode
<html>
<head>
<title>${title}</title>
</head>
<body>
<p>
Welcome, ${names.join(', ')}!
</p>
</body>
</html>
code
var vars = {
title: 'hello world',
names: ['foo', 'bar']
};
odesza.renderFile('hello.ode', vars);
output
<html>
<head>
<title>hello world</title>
</head>
<p>
Welcome, foo, bar
</p>
</body>
</html>
Odesza makes it easy to nest templates within each other. You can include templates as many levels deep as you like. Variables maintain scope in included files.
greeting.ode
hello!
welcome.ode
include greeting
welcome, ${name}!
question.ode
include welcome
would you like to play a game, ${name}?
code
var vars = {
name: 'foo'
};
odesza.renderFile('question,ode', vars);
output
hello!
welcome, foo!
would you like to play a game, foo?
Odesza gives you access to multiple inheritance through extending templates and block scopes.
layout.ode
<!doctype html>
<html>
<head>
<title>${title}</title>
block js
</head>
<body>
block content
</body>
</html>
page.ode (extends layout.ode)
extends layout
block js
<script src="${base_path}/page.js"></script>
endblock
block content
<p>
Some content.
</p>
endblock
extended_page.ode (extends page.ode, overwrites 'content' block)
extends page
block content
<p>
Overwritten content.
</p>
endblock
code
var vars = {
title: 'hello world',
base_path: 'public/js'
};
odesza.renderFile('extended_page.ode', vars);
output
<!doctype html>
<html>
<head>
<title>hello world</title>
<script src="public/js/page.js"></script>
</head>
<body>
<p>
Overwritten content.
</p>
</body>
</html>
Odesza makes it easy to write inline JavaScript in your templates. Under the hood, templates are evaluated as ES6 template strings, which means you have access to ${}
expressions. If you need more flexibility with inline js, you can create a self-executing function expression with code inside it like this: ${(() => { ... })()}
.
greetings.ode
<h2>welcome ${names.join(', ')}!</h2>
${(() => {
// this is a self-executing function expression inside an ES6 template string.
// essentially that means you can write any inline js you want here. the
// following code is to demonstrate how you can programatically generate HTML.
var items = [];
names.forEach((name, index) => {
items.push(`<div>${index + 1}: ${name}</div>`);
});
return items.join('<br/>');
})()}
code
var vars = {
names: ['wells', 'joe', 'dom']
};
odesza.renderFile('greetings.ode', vars);
output
<h2>welcome wells, joe, dom!</h2>
<div>1: wells</div><br/>
<div>2: joe</div><br/>
<div>3: dom</div>
index.js
app.set('view engine', 'ode');
app.engine('.ode', require('odesza').__express);
controller
res.render('template', {
foo: 'bar'
});
You can compile odesza templates from the command line to stdout
or an output file.
odesza <file> [-o <output>]
npm install odesza --save
MIT
FAQs
Write clean, expressive templates with just HTML and inline JavaScript
We found that odesza demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
Application Security
New SEC disclosure rules aim to enforce timely cyber incident reporting, but fear of job loss and inadequate resources lead to significant underreporting.
Security News
The Python Software Foundation has secured a 5-year sponsorship from Fastly that supports PSF's activities and events, most notably the security and reliability of the Python Package Index (PyPI).