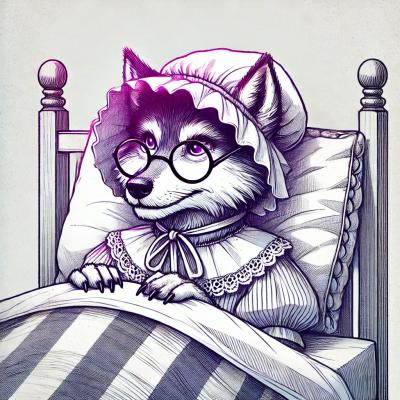
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The ohash npm package is a utility library for handling objects and hashes in JavaScript. It provides a variety of functions to manipulate and work with objects, including creating hashes, deep cloning, and merging objects.
Creating hashes from objects
This feature allows you to create a unique hash string from a JavaScript object. It is useful for identifying objects or storing them in a hash table.
const ohash = require('ohash');
const myObject = { name: 'John', age: 30 };
const hash = ohash.hash(myObject);
console.log(hash);
Deep cloning objects
This feature provides a method to create a deep clone of an object, ensuring that nested objects are also cloned rather than just copying references.
const ohash = require('ohash');
const original = { name: 'John', details: { age: 30, city: 'New York' } };
const cloned = ohash.clone(original);
console.log(cloned);
Merging objects
This feature allows you to merge two or more objects into a single object, combining their properties. If properties overlap, the last object's properties will take precedence.
const ohash = require('ohash');
const object1 = { name: 'John' };
const object2 = { age: 30 };
const mergedObject = ohash.merge(object1, object2);
console.log(mergedObject);
Lodash is a comprehensive utility library that offers similar functionalities to ohash, such as deep cloning and merging objects. Lodash is more extensive and widely used in the industry, providing a broader range of functions and better performance optimizations.
Deepmerge is a package specifically designed for merging objects deeply. While ohash provides this functionality, deepmerge offers more advanced options for controlling the merge process, such as array concatenation and custom merge functions.
Super fast hashing library written in Vanilla JS
Install package:
# npm
npm install ohash
# yarn
yarn add ohash
# pnpm
pnpm install ohash
Import:
// ESM
import { hash, objectHash, murmurHash, sha256 } from 'ohash'
// CommonJS
const { hash, objectHash, murmurHash, sha256 } = require('ohash')
hash(object, options?)
Converts object value into a string hash using objectHash
and then applies sha256
(trimmed by length of 10).
Usage:
import { hash } from 'ohash'
// "7596ed03b7"
console.log(hash({ foo: 'bar'}))
objectHash(object, options?)
Converts a nest object value into a stable and safe string for hashing.
Usage:
import { objectHash } from 'ohash'
// "object:1:string:3:foo:string:3:bar,"
console.log(objectHash({ foo: 'bar'}))
murmurHash(str)
Converts input string (of any length) into a 32-bit positive integer using MurmurHash3.
Usage:
import { murmurHash } from 'ohash'
// "2708020327"
console.log(murmurHash('Hello World'))
sha256
Create a secure SHA 256 digest from input string.
import { sha256 } from 'ohash'
// "a591a6d40bf420404a011733cfb7b190d62c65bf0bcda32b57b277d9ad9f146e"
console.log(sha256('Hello World'))
corepack enable
(use npm i -g corepack
for Node.js < 16.10)pnpm install
pnpm dev
Made with 💛
Published under MIT License.
Based on puleos/object-hash by Scott Puleo, and implementations from perezd/node-murmurhash and garycourt/murmurhash-js by Gary Court and Austin Appleby and brix/crypto-js.
FAQs
Super fast hashing library based on murmurhash3 written in Vanilla JS
The npm package ohash receives a total of 1,487,684 weekly downloads. As such, ohash popularity was classified as popular.
We found that ohash demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.