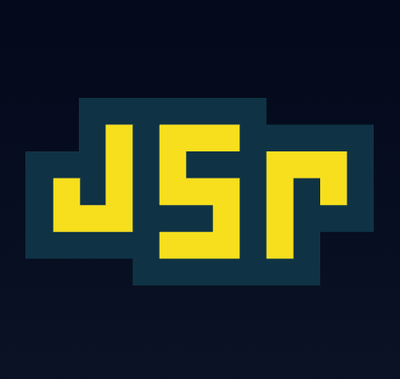
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Install:
# npm
npm i ohmyfetch
# yarn
yarn add ohmyfetch
Import:
// ESM / Typescript
import { $fetch } from 'ohmyfetch'
// CommonJS
const { $fetch } = require('ohmyfetch')
We use conditional exports to detect Node.js
and automatically use node-fetch. If globalThis.fetch
is available, will be used instead.
undici
supportIn order to use experimental fetch implementation from nodejs/undici, You can import from ohmyfetch/undici
.
import { $fetch } from 'ohmyfetch/undici'
On Node.js versions older than 16.5
, node-fetch will be used as the fallback.
keepAlive
supportBy setting FETCH_KEEP_ALIVE
environment variable to true
, A http/https agent will be registred that keeps sockets around even when there are no outstanding requests, so they can be used for future requests without having to reestablish a TCP connection.
Note: This option can potentially introduce memory leaks. Please check node-fetch/node-fetch#1325.
$fetch
will smartly parse JSON and native values using destr, falling back to text if it fails to parse.
const { users } = await $fetch('/api/users')
For binary content types, $fetch
will instead return a Blob
object.
You can optionally provde a different parser than destr, or specify blob
, arrayBuffer
or text
to force parsing the body with the respective FetchResponse
method.
// Use JSON.parse
await $fetch('/movie?lang=en', { parseResponse: JSON.parse })
// Return text as is
await $fetch('/movie?lang=en', { parseResponse: txt => txt })
// Get the blob version of the response
await $fetch('/api/generate-image', { responseType: 'blob' })
$fetch
automatically stringifies request body (if an object is passed) and adds JSON Content-Type
and Accept
headers (for put
, patch
and post
requests).
const { users } = await $fetch('/api/users', { method: 'POST', body: { some: 'json' } })
$fetch
Automatically throw errors when response.ok
is false
with a friendly error message and compact stack (hiding internals).
Parsed error body is available with error.data
. You may also use FetchError
type.
await $fetch('http://google.com/404')
// FetchError: 404 Not Found (http://google.com/404)
// at async main (/project/playground.ts:4:3)
In order to bypass errors as response you can use error.data
:
await $fetch(...).catch((error) => error.data)
$fetch
Automatically retries the request if an error happens. Default is 1
(except for POST
, PUT
and PATCH
methods that is 0
)
await $fetch('http://google.com/404', {
retry: 3
})
Response can be type assisted:
const article = await $fetch<Article>(`/api/article/${id}`)
// Auto complete working with article.id
baseURL
By using baseURL
option, $fetch
prepends it with respecting to trailing/leading slashes and query params for baseURL using ufo:
await $fetch('/config', { baseURL })
By using params
option, $fetch
adds params to URL by preserving params in request itself using ufo:
await $fetch('/movie?lang=en', { params: { id: 123 } })
It is possible to provide async interceptors to hook into lifecycle events of fetch
call.
You might want to use $fetch.create
to set set shared interceptors.
onRequest({ request, options })
onRequest
is called as soon as $fetch
is being called, allowing to modify options or just do simple logging.
await $fetch('/api', {
async onRequest({ request, options }) {
// Log request
console.log('[fetch request]', request, options)
// Add `?t=1640125211170` to query params
options.params = options.params
options.params.t = new Date()
}
})
onRequestError({ request, options, error })
onRequestError
will be called when fetch request fails.
await $fetch('/api', {
async onRequestError({ request, options, error }) {
// Log error
console.log('[fetch request error]', request, error)
}
})
onResponse({ request, options, response })
onResponse
will be called after fetch
call and parsing body.
await $fetch('/api', {
async onResponse({ request, response, options }) {
// Log response
console.log('[fetch response]', request, response.code, reponse.body)
}
})
onResponseError({ request, options, response })
onResponseError
is same as onResponse
but will be called when fetch happens but response.ok
is not true
.
await $fetch('/api', {
async onResponseError({ request, response, options }) {
// Log error
console.log('[fetch response error]', request, reponse.code, response.body)
}
})
This utility is useful if you need to use common options across serveral fetch calls.
Note: Defaults will be cloned at one level and inherrited. Be careful about nested options like headers
.
const apiFetch = $fetch.create({ baseURL: '/api' })
apiFetch('/test') // Same as $fetch('/test', { baseURL: '/api' })
By using headers
option, $fetch
adds extra headers in addition to the request default headers:
await $fetch('/movies', {
headers: {
Accept: 'application/json',
'Cache-Control': 'no-cache'
}
})
If you need to access raw response (for headers, etc), can use $fetch.raw
:
const response = await $fetch.raw('/sushi')
// response.data
// response.headers
// ...
ohmyfetch
, destr
and ufo
packages with babel for ES5 supportfetch
global for supporting legacy browsers like using unfetchWhy export is called $fetch
instead of fetch
?
Using the same name of fetch
can be confusing since API is different but still it is a fetch so using closest possible alternative. You can however, import { fetch }
from ohmyfetch
which is auto polyfilled for Node.js and using native otherwise.
Why not having default export?
Default exports are always risky to be mixed with CommonJS exports.
This also guarantees we can introduce more utils without breaking the package and also encourage using $fetch
name.
Why not transpiled?
By keep transpiling libraries we push web backward with legacy code which is unneeded for most of the users.
If you need to support legacy users, you can optionally transpile the library in your build pipeline.
MIT. Made with 💖
FAQs
oh-my-fetch
The npm package ohmyfetch receives a total of 22,971 weekly downloads. As such, ohmyfetch popularity was classified as popular.
We found that ohmyfetch demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.