Comparing version 0.4.0 to 0.4.2
@@ -11,3 +11,3 @@ { | ||
"author": "Fatih Kadir Akın <fka@fatihak.in>", | ||
"version": "0.4.0", | ||
"version": "0.4.2", | ||
"licenses": [ | ||
@@ -14,0 +14,0 @@ { |
132
README.md
@@ -1,5 +0,8 @@ | ||
# Omelette.js v0.4.0 | ||
<img src="https://rawgit.com/f/omelette/master/resources/logo.svg?v1" height="80"> | ||
[](https://travis-ci.org/f/omelette) | ||
> Omelette is a simple, template based autocompletion tool for Node projects with super easy API. | ||
[](https://badge.fury.io/js/omelette) | ||
[](https://travis-ci.org/f/omelette) | ||
```bash | ||
@@ -11,20 +14,12 @@ yarn add omelette | ||
Omelette is a simple, template based autocompletion tool for Node projects. | ||
You just have to decide your program name and CLI fragments. | ||
```javascript | ||
omelette('githubber <module> <command> <suboption>') | ||
omelette`github ${['pull', 'push']} ${['origin', 'upstream']} ${['master', 'develop']}`.init() | ||
``` | ||
And you are almost done! | ||
And you are almost done! The output will be like this: | ||
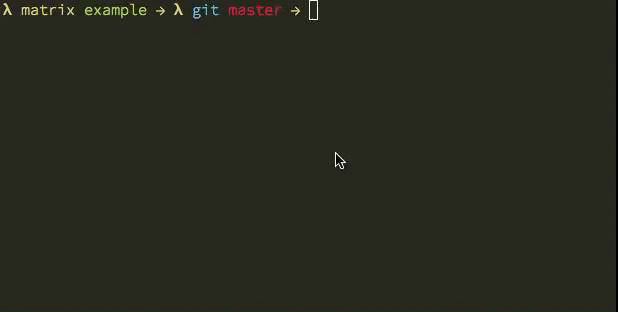 | ||
<img src="https://raw.github.com/f/omelette/master/resources/omelette-new.gif?v1" width="640"> | ||
A more detailed template spec: | ||
```javascript | ||
omelette('<programname>[|<shortname>|<short>|<...>] <module> [<command> <suboption> <...>]') | ||
``` | ||
## Quick Start | ||
@@ -37,14 +32,16 @@ | ||
const completion = omelette('programname|prgmnm|prgnm <firstargument>'); | ||
const firstArgument = ({ reply }) => { | ||
reply([ 'beautiful', 'cruel', 'far' ]) | ||
} | ||
completion.on('firstargument', ({ reply }) => { | ||
reply(["hello", "cruel", "world"]); | ||
}); | ||
const planet = ({ reply }) => { | ||
reply([ 'world', 'mars', 'pluto' ]) | ||
} | ||
comp.init(); | ||
omelette`hello|hi ${firstArgument} ${planet}`.init() | ||
``` | ||
**You can add multiple names to programs** | ||
<img src="https://raw.github.com/f/omelette/master/resources/omelette-new-hello.gif?v1" width="640"> | ||
### Code | ||
### Simple Event Based API ☕️ | ||
@@ -64,24 +61,28 @@ It's based on a simple CLI template. | ||
// Bind events for every template part. | ||
completion.on("action", ({ reply }) => reply(["clone", "update", "push"])); | ||
completion.on('action', ({ reply }) => { | ||
reply([ 'clone', 'update', 'push' ]) | ||
}) | ||
completion.on("user", ({ reply }) => reply(fs.readdirSync("/Users/"))); | ||
completion.on('user', ({ reply }) => { | ||
reply(fs.readdirSync('/Users/')) | ||
}) | ||
completion.on("repo", ({ before, reply }) => { | ||
completion.on('repo', ({ before, reply }) => { | ||
reply([ | ||
`http://github.com/${before}/helloworld`, | ||
`http://github.com/${before}/blabla` | ||
]); | ||
); | ||
]) | ||
}) | ||
// Initialize the omelette. | ||
completion.init(); | ||
completion.init() | ||
// If you want to have a setup feature, you can use `omeletteInstance.setupShellInitFile()` function. | ||
if (~process.argv.indexOf('--setup') { | ||
complete.setupShellInitFile(); | ||
complete.setupShellInitFile() | ||
} | ||
// Rest is yours | ||
console.log("Your program's default workflow."); | ||
console.log(process.argv); | ||
console.log("Your program's default workflow.") | ||
console.log(process.argv) | ||
``` | ||
@@ -91,3 +92,3 @@ | ||
### ES6 Template Literals 🚀 | ||
### ES6 Template Literal API 🚀 | ||
@@ -100,5 +101,23 @@ You can use **Template Literals** to define your completion with a simpler (super easy) API. | ||
// Just pass a template literal to use super easy API. | ||
omelette`hello ${['cruel', 'nice']} ${['world', 'mars']}`.init(); | ||
omelette`hello ${[ 'cruel', 'nice' ]} ${[ 'world', 'mars' ]}`.init() | ||
``` | ||
Let's make the example above with ES6 TL: | ||
```javascript | ||
import * as omelette from 'omelette' | ||
// Write your CLI template. | ||
omelette` | ||
githubber|gh | ||
${[ 'clone', 'update', 'push' ]} | ||
${() => fs.readdirSync('/Users/')} | ||
${({ before }) => [ | ||
`http://github.com/${before}/helloworld`, | ||
`http://github.com/${before}/blabla`, | ||
]} | ||
`.init() | ||
``` | ||
Also you can still use lambda functions to make more complex template literals: | ||
@@ -122,8 +141,8 @@ | ||
]} | ||
`.init(); | ||
`.init() | ||
// No extra configuration required. | ||
console.log("Your program's default workflow."); | ||
console.log(process.argv); | ||
console.log("Your program's default workflow.") | ||
console.log(process.argv) | ||
``` | ||
@@ -141,3 +160,3 @@ | ||
// If you want to write file, | ||
complete.setupShellInitFile('~/.my_bash_profile'); | ||
complete.setupShellInitFile('~/.my_bash_profile') | ||
``` | ||
@@ -148,5 +167,5 @@ | ||
If you use Zsh, it just append a loader code to `~/.zshrc` file. | ||
If you use Zsh, it appends a loader code to `~/.zshrc` file. | ||
If you use Fish, it just append a loader code to `~/.config/fish/config.fish` file. | ||
If you use Fish, it appends a loader code to `~/.config/fish/config.fish` file. | ||
@@ -186,4 +205,5 @@ *TL;DR: It does the Manual Install part, basically.* | ||
### Parameters | ||
### Parameters | ||
Callbacks have two parameters: | ||
@@ -194,3 +214,3 @@ | ||
- `fragment`: The number of fragment. | ||
- `prev`: The previous word. | ||
- `before`: The previous word. | ||
- `line`: The whole command line buffer allow you to parse and reply as you wish. | ||
@@ -215,2 +235,36 @@ - `reply`: This is the reply function to use *this-less* API. | ||
### Autocompletion Tree | ||
You can create **completion tree** to more complex autocompletions. | ||
```js | ||
omelette('hello').tree({ | ||
how: { | ||
much: { | ||
is: { | ||
this: ['car'], | ||
that: ['house'], | ||
} | ||
} | ||
are: ['you'], | ||
many: ['cars', 'houses'], | ||
}, | ||
where: { | ||
are: { | ||
you: ['from'], | ||
the: ['houses', 'cars'], | ||
}, | ||
is: { | ||
your: ['house', 'car'], | ||
} | ||
}, | ||
}).init() | ||
``` | ||
Now you will be able to use your completion as tree. | ||
<img src="https://raw.github.com/f/omelette/master/resources/omelette-tree-new.gif?v1" width="640"> | ||
> Thanks [@jblandry](https://github.com/jblandry) for the idea. | ||
### Short Names | ||
@@ -217,0 +271,0 @@ |
@@ -8,5 +8,5 @@ // Generated by CoffeeScript 1.12.5 | ||
(function() { | ||
var EventEmitter, Omelette, fs, os, path, | ||
var EventEmitter, Omelette, depthOf, fs, os, path, | ||
hasProp = {}.hasOwnProperty, | ||
extend = function(child, parent) { for (var key in parent) { if (hasProp.call(parent, key)) child[key] = parent[key]; } function ctor() { this.constructor = child; } ctor.prototype = parent.prototype; child.prototype = new ctor(); child.__super__ = parent.prototype; return child; }, | ||
hasProp = {}.hasOwnProperty, | ||
slice = [].slice; | ||
@@ -22,2 +22,15 @@ | ||
depthOf = function(object) { | ||
var depth, key, level; | ||
level = 1; | ||
for (key in object) { | ||
if (!hasProp.call(object, key)) continue; | ||
if (typeof object[key] === 'object') { | ||
depth = depthOf(object[key]) + 1; | ||
level = Math.max(depth, level); | ||
} | ||
} | ||
return level; | ||
}; | ||
Omelette = (function(superClass) { | ||
@@ -31,3 +44,3 @@ var log; | ||
function Omelette() { | ||
var isFish, isZsh, ref; | ||
var isFish, isZsh, ref, ref1; | ||
this.compgen = process.argv.indexOf("--compgen"); | ||
@@ -41,4 +54,4 @@ this.install = process.argv.indexOf("--completion") > -1; | ||
this.line = process.argv[this.compgen + 3]; | ||
this.word = this.line.trim().split(/\s+/).pop(); | ||
ref = process.env, this.HOME = ref.HOME, this.SHELL = ref.SHELL; | ||
this.word = (ref = this.line) != null ? ref.trim().split(/\s+/).pop() : void 0; | ||
ref1 = process.env, this.HOME = ref1.HOME, this.SHELL = ref1.SHELL; | ||
} | ||
@@ -82,2 +95,30 @@ | ||
Omelette.prototype.tree = function(objectTree) { | ||
var depth, i, level, ref; | ||
if (objectTree == null) { | ||
objectTree = {}; | ||
} | ||
depth = depthOf(objectTree); | ||
for (level = i = 1, ref = depth; 1 <= ref ? i <= ref : i >= ref; level = 1 <= ref ? ++i : --i) { | ||
this.on("$" + level, function(arg) { | ||
var accessor, fragment, line, replies, reply; | ||
fragment = arg.fragment, reply = arg.reply, line = arg.line; | ||
accessor = new Function('_', "return _['" + (line.split(/\s+/).slice(1).filter(Boolean).join("']['")) + "']"); | ||
replies = fragment === 1 ? Object.keys(objectTree) : accessor(objectTree); | ||
return reply((function(replies) { | ||
if (replies instanceof Function) { | ||
return replies(); | ||
} | ||
if (replies instanceof Array) { | ||
return replies; | ||
} | ||
if (replies instanceof Object) { | ||
return Object.keys(replies); | ||
} | ||
})(replies)); | ||
}); | ||
} | ||
return this; | ||
}; | ||
Omelette.prototype.generateCompletionCode = function() { | ||
@@ -84,0 +125,0 @@ var completions; |
Sorry, the diff of this file is not supported yet
Uses eval
Supply chain riskPackage uses dynamic code execution (e.g., eval()), which is a dangerous practice. This can prevent the code from running in certain environments and increases the risk that the code may contain exploits or malicious behavior.
Found 1 instance in 1 package
1479251
14
250
338
1