oo-redux-utils
Advanced tools
Comparing version
@@ -1,17 +0,60 @@ | ||
// noinspection JSUnusedGlobalSymbols | ||
export default class OOReduxUtils { | ||
// noinspection JSUnusedGlobalSymbols | ||
static mergeOwnAndForeignState(ownState, foreignState) { | ||
const overlappingOwnAndForeignStateKeys = Object.keys(ownState).filter(ownStateKey => Object.keys(foreignState).includes(ownStateKey)); | ||
"use strict"; | ||
if (overlappingOwnAndForeignStateKeys.length > 0) { | ||
throw new Error('One or more overlapping properties in own and foreign state'); | ||
} | ||
Object.defineProperty(exports, "__esModule", { | ||
value: true | ||
}); | ||
exports.OOReduxUtils = void 0; | ||
return { ...ownState, | ||
...foreignState | ||
}; | ||
var _AbstractAction = require("./AbstractAction"); | ||
function ownKeys(object, enumerableOnly) { var keys = Object.keys(object); if (Object.getOwnPropertySymbols) { var symbols = Object.getOwnPropertySymbols(object); if (enumerableOnly) symbols = symbols.filter(function (sym) { return Object.getOwnPropertyDescriptor(object, sym).enumerable; }); keys.push.apply(keys, symbols); } return keys; } | ||
function _objectSpread(target) { for (var i = 1; i < arguments.length; i++) { var source = arguments[i] != null ? arguments[i] : {}; if (i % 2) { ownKeys(source, true).forEach(function (key) { _defineProperty(target, key, source[key]); }); } else if (Object.getOwnPropertyDescriptors) { Object.defineProperties(target, Object.getOwnPropertyDescriptors(source)); } else { ownKeys(source).forEach(function (key) { Object.defineProperty(target, key, Object.getOwnPropertyDescriptor(source, key)); }); } } return target; } | ||
function _defineProperty(obj, key, value) { if (key in obj) { Object.defineProperty(obj, key, { value: value, enumerable: true, configurable: true, writable: true }); } else { obj[key] = value; } return obj; } | ||
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } } | ||
function _defineProperties(target, props) { for (var i = 0; i < props.length; i++) { var descriptor = props[i]; descriptor.enumerable = descriptor.enumerable || false; descriptor.configurable = true; if ("value" in descriptor) descriptor.writable = true; Object.defineProperty(target, descriptor.key, descriptor); } } | ||
function _createClass(Constructor, protoProps, staticProps) { if (protoProps) _defineProperties(Constructor.prototype, protoProps); if (staticProps) _defineProperties(Constructor, staticProps); return Constructor; } | ||
var OOReduxUtils = | ||
/*#__PURE__*/ | ||
function () { | ||
function OOReduxUtils() { | ||
_classCallCheck(this, OOReduxUtils); | ||
} | ||
} | ||
_createClass(OOReduxUtils, null, [{ | ||
key: "mergeOwnAndForeignState", | ||
// noinspection JSUnusedGlobalSymbols | ||
value: function mergeOwnAndForeignState(ownState, foreignState) { | ||
var overlappingOwnAndForeignStateKeys = Object.keys(ownState).filter(function (ownStateKey) { | ||
return Object.keys(foreignState).includes(ownStateKey); | ||
}); | ||
if (overlappingOwnAndForeignStateKeys.length > 0) { | ||
throw new Error('One or more overlapping properties in own and foreign state'); | ||
} | ||
return _objectSpread({}, ownState, {}, foreignState); | ||
} // noinspection JSUnusedGlobalSymbols | ||
}, { | ||
key: "createStateReducer", | ||
value: function createStateReducer(initialState, actionBaseClass) { | ||
var stateNamespace = arguments.length > 2 && arguments[2] !== undefined ? arguments[2] : ''; | ||
return function () { | ||
var currentState = arguments.length > 0 && arguments[0] !== undefined ? arguments[0] : initialState; | ||
var action = arguments.length > 1 ? arguments[1] : undefined; | ||
return action.type instanceof actionBaseClass && action.type.getStateNamespace() === stateNamespace ? action.type.performActionAndReturnNewState(currentState) : currentState; | ||
}; | ||
} | ||
}]); | ||
return OOReduxUtils; | ||
}(); | ||
exports.OOReduxUtils = OOReduxUtils; | ||
//# sourceMappingURL=OOReduxUtils.js.map |
{ | ||
"name": "oo-redux-utils", | ||
"version": "1.0.1", | ||
"version": "1.1.0", | ||
"description": "Utility functions for Object-oriented Redux", | ||
@@ -17,3 +17,4 @@ "keywords": [ | ||
"scripts": { | ||
"build": "babel ./src --out-dir ./lib --source-maps", | ||
"clean": "rimraf ./lib", | ||
"build": "npm run clean && babel ./src --out-dir ./lib --source-maps && flow-copy-source ./src ./lib", | ||
"test": "jest ./test --coverage" | ||
@@ -34,2 +35,3 @@ }, | ||
"@babel/core": "^7.5.5", | ||
"@babel/plugin-proposal-class-properties": "^7.5.5", | ||
"@babel/preset-env": "^7.5.5", | ||
@@ -44,5 +46,13 @@ "@babel/preset-flow": "^7.0.0", | ||
"flow-bin": "^0.105.2", | ||
"flow-copy-source": "^2.0.8", | ||
"jest": "^24.9.0", | ||
"prettier": "^1.18.2" | ||
"prettier": "^1.18.2", | ||
"rimraf": "^3.0.0" | ||
}, | ||
"browserslist": [ | ||
"last 2 version", | ||
"> 0.2%", | ||
"Firefox ESR", | ||
"not dead" | ||
], | ||
"prettier": { | ||
@@ -49,0 +59,0 @@ "arrowParens": "always", |
195
README.md
@@ -1,4 +0,5 @@ | ||
# React Single File Components (SFC) Webpack Loader | ||
Webpack loader for React Single File Components (SFC) inspired by [Vue SFCs] | ||
# Object-oriented Redux Utils | ||
Object-oriented Redux Utils | ||
<!-- | ||
[![version][version-badge]][package] | ||
@@ -8,193 +9,11 @@ [![build][build]][circleci] | ||
[![MIT License][license-badge]][license] | ||
--> | ||
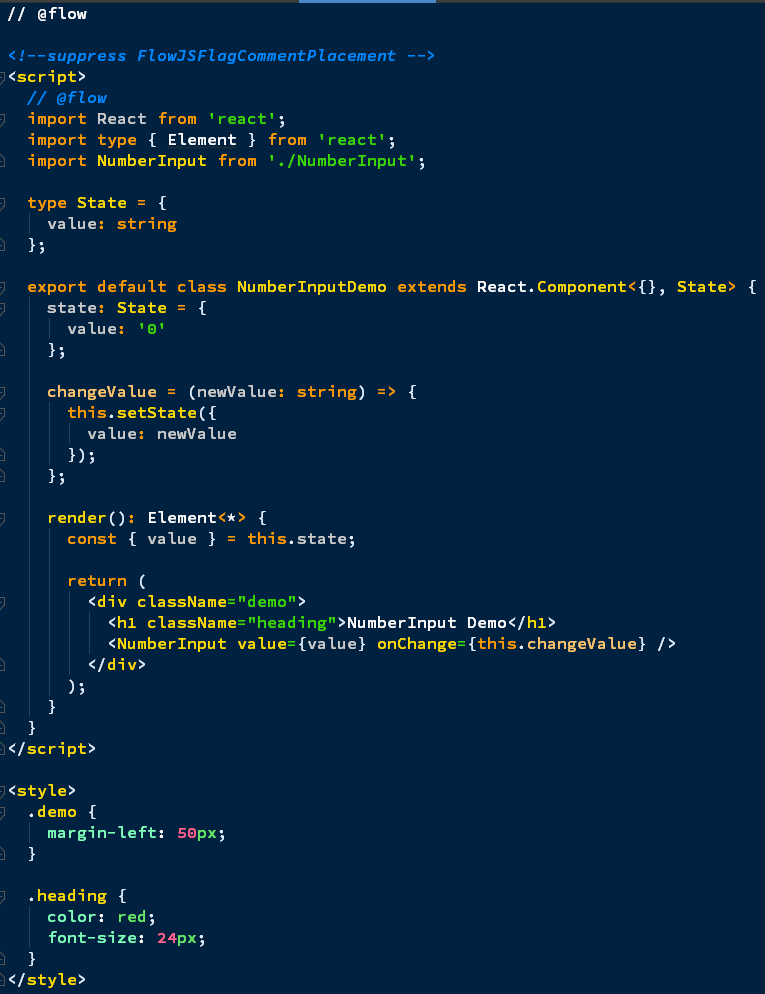 | ||
## Prerequisites | ||
"webpack": "^4.0.0", | ||
"react-redux": "^6.0.0", | ||
"redux": "^4.0.1" | ||
## Installation | ||
npm install --save-dev react-sfc-webpack-loader | ||
## React Single File Component | ||
React Single File Component (SFC) is implemented in a .html file where JavaScript is put inside a single <script>...</script> section | ||
and optional CSS is put inside a single (optional) <style>...</style> section | ||
## Example | ||
See example in [example directory] | ||
### Style types | ||
Define style type as follows: | ||
CSS | ||
npm install --save oo-redux-utils | ||
<style> | ||
... | ||
.. | ||
</style> | ||
or | ||
<style type="text/css"> | ||
... | ||
.. | ||
</style> | ||
SCSS | ||
<style type="text/scss"> | ||
... | ||
.. | ||
</style> | ||
SASS | ||
<style type="text/sass"> | ||
... | ||
.. | ||
</style> | ||
LESS | ||
<style type="text/less"> | ||
... | ||
.. | ||
</style> | ||
Stylus | ||
<style type="text/stylus"> | ||
... | ||
.. | ||
</style> | ||
## CSS Modules | ||
CSS Modules support is enabled with scoped attribute: | ||
<style scoped type="text/scss"> | ||
... | ||
.. | ||
</style> | ||
Your CSS rule for CSS modules in Webpack config must test file extension .module.<style-type>, e.g. .module.css or .module.scss, for example: | ||
{ | ||
test: /\.module.scss$/, | ||
exclude: /node_modules/, | ||
use: [ | ||
'style-loader', | ||
{ | ||
loader: 'css-loader', | ||
options: { | ||
modules: { | ||
mode: 'local', | ||
localIdentName: '[local]--[hash:base64:5]' | ||
} | ||
} | ||
}, | ||
'sass-loader' | ||
] | ||
} | ||
Your CSS is available in .html files through object named "styles", for example: | ||
<div className={styles.demo}> | ||
If you use ESLint and get error of undefined 'styles', add following line to .html file: | ||
/*global styles*/ | ||
## Webpack configuration | ||
### Create React App | ||
If you have created your React app with Create React App, you need to eject it by running: | ||
npm eject | ||
or | ||
yarn eject | ||
Add following rule to rules array in config/webpack.config.js file: | ||
module: { | ||
rules: [ | ||
{ | ||
test: /\.html$/, | ||
exclude: /node_modules/, | ||
use: ['babel-loader', 'react-sfc-webpack-loader'] | ||
} | ||
### Manual configuration | ||
Have your normal Webpack configuration | ||
Have your normal rules for style loading depending on style type (CSS/SCSS/SASS/LESS/Stylus) | ||
Only change needed is to add this following rule to Webpack configuration: | ||
module: { | ||
rules: [ | ||
{ | ||
test: /\.html$/, | ||
exclude: /node_modules/, | ||
use: ['babel-loader', 'react-sfc-webpack-loader'] | ||
} | ||
## Supported tools | ||
* [Prettier] | ||
* [StyleLint] | ||
Use for example following npm script in your package.json: | ||
"stylelint": "stylelint src/**/*.html", | ||
* [ESLint] (below steps must be done in addition to normal ESLint installation and configuration) | ||
* Install eslint-plugin-html | ||
npm install --save-dev eslint-plugin-html | ||
* Add to your ESLint configuration | ||
{ | ||
"plugins": [ | ||
"html" | ||
], | ||
rules: [ | ||
"react/jsx-filename-extension": [1, { "extensions": [".js", ".jsx", ".html"] }] | ||
] | ||
} | ||
* [Flow] (Needs ESLint and below steps must be done in addition to normal Flow installation and configuration) | ||
* Install eslint-plugin-flowtype-errors | ||
npm install --save-dev eslint-plugin-flowtype-errors | ||
* Configure ESLint | ||
{ | ||
"plugins": [ | ||
"flowtype-errors" | ||
], | ||
rules: [ | ||
"flowtype-errors/show-errors": 2 | ||
] | ||
} | ||
* Enable flow usage in .html file | ||
// @flow | ||
<script> | ||
// @flow | ||
. | ||
. | ||
. | ||
</script> | ||
## Tested IDEs/Editors | ||
* WebStorm | ||
## Not supported | ||
* TypeScript | ||
## License | ||
@@ -201,0 +20,0 @@ MIT License |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
14706
40.45%12
100%98
653.85%16
23.08%35
-83.8%