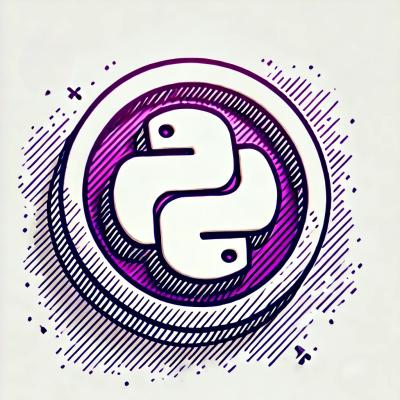
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
openapi-fetch
Advanced tools
Fast, type-safe fetch client for your OpenAPI schema. Only 5 kb (min). Works with React, Vue, Svelte, or vanilla JS.
The openapi-fetch npm package is a tool designed to simplify the process of making HTTP requests to APIs that are defined using the OpenAPI specification. It provides a type-safe way to interact with APIs, ensuring that the requests and responses conform to the defined API schema.
Type-safe API requests
This feature allows you to make type-safe API requests based on the OpenAPI definition. The `createClient` function initializes the client with the API definition, and you can then use methods like `get` to make requests. The types are inferred from the OpenAPI schema, ensuring that your requests and responses are type-checked.
const { createClient } = require('openapi-fetch');
const api = createClient({ definition: 'https://api.example.com/openapi.json' });
async function fetchUser() {
const response = await api.get('/users/{id}', { params: { id: 1 } });
console.log(response.data);
}
fetchUser();
Automatic request validation
This feature ensures that the requests you make are validated against the OpenAPI schema. If the request does not conform to the schema, an error will be thrown. This helps in catching errors early in the development process.
const { createClient } = require('openapi-fetch');
const api = createClient({ definition: 'https://api.example.com/openapi.json' });
async function createUser() {
const response = await api.post('/users', { body: { name: 'John Doe', email: 'john.doe@example.com' } });
console.log(response.data);
}
createUser();
Response validation
This feature validates the responses from the API against the OpenAPI schema. It ensures that the data you receive is in the expected format, reducing the chances of runtime errors due to unexpected data structures.
const { createClient } = require('openapi-fetch');
const api = createClient({ definition: 'https://api.example.com/openapi.json' });
async function fetchUser() {
const response = await api.get('/users/{id}', { params: { id: 1 } });
if (response.status === 200) {
console.log('User data:', response.data);
} else {
console.error('Failed to fetch user:', response.error);
}
}
fetchUser();
Axios is a popular HTTP client for making requests to APIs. While it does not provide built-in support for OpenAPI specifications, it is highly configurable and can be used with additional libraries to achieve similar functionality. Compared to openapi-fetch, axios requires more manual setup for type safety and validation.
Swagger Client is a JavaScript client for connecting to APIs that use the OpenAPI (Swagger) specification. It provides similar functionality to openapi-fetch, including type-safe requests and response validation. However, Swagger Client is more tightly integrated with the Swagger ecosystem and may offer more features for users already using Swagger tools.
openapi-client-axios is a library that combines the features of OpenAPI and axios. It generates an axios client based on an OpenAPI definition, providing type-safe requests and response validation. It is similar to openapi-fetch in terms of functionality but leverages axios for the underlying HTTP requests.
openapi-fetch is a type-safe fetch client that pulls in your OpenAPI schema. Weighs 5 kb and has virtually zero runtime. Works with React, Vue, Svelte, or vanilla JS.
Library | Size (min) | “GET” request* |
---|---|---|
openapi-fetch | 5 kB | 300k ops/s (fastest) |
openapi-typescript-fetch | 4 kB | 150k ops/s (2× slower) |
axios | 32 kB | 225k ops/s (1.3× slower) |
superagent | 55 kB | 50k ops/s (6× slower) |
openapi-typescript-codegen | 367 kB | 100k ops/s (3× slower) |
* Benchmarks are approximate to just show rough baseline and will differ among machines and browsers. The relative performance between libraries is more reliable.
The syntax is inspired by popular libraries like react-query or Apollo client, but without all the bells and whistles and in a 5 kb package.
import createClient from "openapi-fetch";
import type { paths } from "./my-openapi-3-schema"; // generated by openapi-typescript
const client = createClient<paths>({ baseUrl: "https://myapi.dev/v1/" });
const {
data, // only present if 2XX response
error, // only present if 4XX or 5XX response
} = await client.GET("/blogposts/{post_id}", {
params: {
path: { post_id: "123" },
},
});
await client.PUT("/blogposts", {
body: {
title: "My New Post",
},
});
data
and error
are typechecked and expose their shapes to Intellisense in VS Code (and any other IDE with TypeScript support). Likewise, the request body
will also typecheck its fields, erring if any required params are missing, or if there’s a type mismatch.
GET()
, PUT()
, POST()
, etc. are thin wrappers around the native fetch API (which you can swap for any call).
Notice there are no generics, and no manual typing. Your endpoint’s request and response were inferred automatically. This is a huge improvement in the type safety of your endpoints because every manual assertion could lead to a bug! This eliminates all of the following:
any
types that hide bugsas
type overrides that can also hide bugsInstall this library along with openapi-typescript:
npm i openapi-fetch
npm i -D openapi-typescript typescript
Highly recommended
Enable noUncheckedIndexedAccess in your
tsconfig.json
(docs)
Next, generate TypeScript types from your OpenAPI schema using openapi-typescript:
npx openapi-typescript ./path/to/api/v1.yaml -o ./src/lib/api/v1.d.ts
Lastly, be sure to run typechecking in your project. This can be done by adding tsc --noEmit
to your npm scripts like so:
{
"scripts": {
"test:ts": "tsc --noEmit"
}
}
And run npm run test:ts
in your CI to catch type errors.
TIP:
Use
tsc --noEmit
to check for type errors rather than relying on your linter or your build command. Nothing will typecheck as accurately as the TypeScript compiler itself.
The best part about using openapi-fetch over oldschool codegen is no documentation needed. openapi-fetch encourages using your existing OpenAPI documentation rather than trying to find what function to import, or what parameters that function wants:
import createClient from "openapi-fetch";
import type { paths } from "./my-openapi-3-schema"; // generated by openapi-typescript
const client = createClient<paths>({ baseUrl: "https://myapi.dev/v1/" });
const { data, error } = await client.GET("/blogposts/{post_id}", {
params: {
path: { post_id: "my-post" },
query: { version: 2 },
},
});
const { data, error } = await client.PUT("/blogposts", {
body: {
title: "New Post",
body: "<p>New post body</p>",
publish_date: new Date("2023-03-01T12:00:00Z").getTime(),
},
});
createClient()
path
to GET()
, PUT()
, etc.FAQs
Fast, type-safe fetch client for your OpenAPI schema. Only 6 kb (min). Works with React, Vue, Svelte, or vanilla JS.
The npm package openapi-fetch receives a total of 273,203 weekly downloads. As such, openapi-fetch popularity was classified as popular.
We found that openapi-fetch demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.