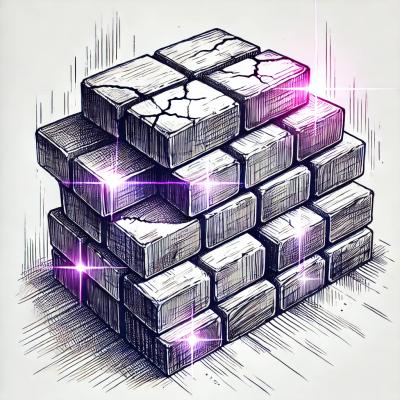
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Orion is a configurable server application, which allows you to build a fully functional REST API in just a few steps! This library is built on top of Express. It sets up all the necessary CRUD data endpoints, file uploads, authentication endpoints, and error handling.
The latest version of the library supports the following components:
In this documentation:
$ npm install --save orion-api
module.exports =
{
database:
{
engine: "mssql",
connectionString: _DATABASE_CONNECTION_STRING_
},
entities:
{
"blogpost":
{
"fields":
{
"title": { type: "string", isEditable: true, createReq: 2, foreignKey: null },
"content": { type: "richtext", isEditable: true, createReq: 2, foreignKey: null }
},
"allowedRoles":
{
"read": ["guest"],
"create": ["guest"],
"update": ["guest"],
"delete": ["guest"]
}
}
}
}
Please see the configuration section for more configuration options.$ node node_modules/orion-api/setup.js ./config.js ./setup.sql
The above command will create an SQL query file named setup.sql that you can run on the database server to set up the tables.var orion = require('orion-api');
var config = require('./config');
var app = orion.create(config);
// You can add more endpoints to the app object or do other things here
app.start();
$ node server.js
For the blog post example above, you can test it by running a POST to add a blog post entry and GET to retrieve it.
$ # insert a new blog post entry
$ curl -d '{"title":"I like trains", "content":"Trains are cool!"}' -H "Content-Type: application/json" -X POST http://localhost:1337/api/data/blogpost
$
$ # retrieve all blog post entries
$ curl http://localhost:1337/api/data/blogpost/public/findall/id/0/100
Go to API Endpoints section to see all of the endpoints that we provide.A configuration module is required to give the application the necessary information about your project. This module should specify the connection strings, authentication and authorization settings, user roles and access levels, and most importantly, the data models.
Below is the list of settings to be included in a configuration module:
Here are the properties that must/may be included in an entity configuration object:
Here are the properties that must/may be included in a field configuration object:
{
id: 123
title: "Roses are red"
authorId: 456
author:
{
id: 456,
name: "John Doe"
}
}
There are some fields and entities that we add to the config at runtime, both when an SQL query is being constructed using setup.js and when an actual API app is being initialized.
Here are the default fields:
name | value | type | isEditable | createReq | foreignEntity | resolvedKeyName |
---|---|---|---|---|---|---|
id | id of the record | id | false | 0 | null | null |
ownerid | id of the record owner | int | false | 0 | user | owner |
createdtime | timestamp of the record creation | timestamp | false | 0 | null | null |
The data types "id" and "timestamp" are special types reserved only for fields "id" and "createdtime". We add the default fields above to every entity specified in the config, except those that are part of the default entities (see below). Default fields cannot be overridden, so if a field with the same name as one of the default fields exists in the config, that field will be ignored.
Here are the default entities:
fields
name | value | type | isEditable | createReq | foreignEntity | resolvedKeyName |
---|---|---|---|---|---|---|
id | user id | id | false | 0 | null | null |
domain | domain where user info is hosted | string | false | 0 | null | null |
domainid | user id on its domain | string | false | 0 | null | null |
roles | user roles (comma separated) | string | false | 0 | null | null |
username | username | string | true | 2 | null | null |
password | password | secret | true | 2 | null | null |
string | true | 2 | null | null | ||
firstname | first name | string | true | 1 | null | null |
lastname | last name | string | true | 1 | null | null |
createdtime | timestamp of the record creation | timestamp | false | 0 | null | null |
allowedRoles
action | roles |
---|---|
read | member, owner, admin |
create | guest |
update | owner, admin |
delete | admin |
fields
name | value | type | isEditable | createReq | foreignEntity | resolvedKeyName |
---|---|---|---|---|---|---|
id | id of the record | id | false | 0 | null | null |
ownerid | id of the record owner | int | false | 0 | user | owner |
filename | file name of the asset | string | false | 2 | null | null |
allowedRoles
action | roles |
---|---|
read | owner, admin |
create | member |
update | |
delete | owner, admin |
The existing fields in the default entities above cannot be overriden, but the list itself can be extended. For instance, in the config you can specify additional fields "firstname" and "lastname" for the user entity. The allowedRoles can be overriden, so in the config you can specify your own permission rules for any of the default entities. You can also specify a getReadCondition and an isWriteAllowed functions for a default entity.
Here is a sample configuration that utilize the provided features (authentication, storage, granular permission checks). You can find it here.
GET /api/data/:entity/:accessType/findbyid/:id
Retrieve a record by its ID.
Parameters:
Success response:
GET /api/data/:entity/:accessType/findbycondition/:orderByField/:skip/:take/:condition
Retrieve records that match a certain set of conditions.
Parameters:
Success response:
GET /api/data/:entity/:accessType/findall/:orderByField/:skip/:take
Retrieve all records in a certain entity.
Parameters:
Success response:
POST /api/data/asset
Upload a file into the file storage (specified in the config) and add a database entry for it.
If the endpoint is set to be open to authenticated users only, an Authorization header containing an access token is required. See Authentication section for more details on how to get the access token.
Request body:
Success response: The inserted asset ID
POST /api/data/:entity
Add a new record to an entity.
If the endpoint is set to be open to authenticated users only, an Authorization header containing an access token is required. See Authentication section for more details on how to get the access token.
Parameters:
Request body: JSON object representation of the new record
Success response: The inserted ID
PUT /api/data/:entity/:id
Update a record in an entity.
If the endpoint is set to be open to authenticated users only, an Authorization header containing an access token is required. See Authentication section for more details on how to get the access token.
Parameters:
Request body: JSON object representation of the new record
Success response: 200 status code
DELETE /api/data/asset/:id
Delete an uploaded file from the file storage and from database.
If the endpoint is set to be open to authenticated users only, an Authorization header containing an access token is required. See Authentication section for more details on how to get the access token.
Parameters:
Success response: 200 status code
DELETE /api/data/:entity/:id
Delete a record from an entity.
If the endpoint is set to be open to authenticated users only, an Authorization header containing an access token is required. See Authentication section for more details on how to get the access token.
Parameters:
Success response: 200 status code
POST /api/auth/token
Get an access token using a set of login credentials. This can be used if all first party authentication settings are specified in the config. See Authentication section for more details on how to get the access token.
Request body:
Success response:
POST /api/auth/token/fb
Get an access token using a temporary Facebook token. See Authentication section for more details on how to get the access token using Facebook token.
Request body:
Success response:
POST /api/error
Log an error message. The logs will be stored in a table called "errortable" in the database. There is currently no built-in endpoint for retrieving these logs, so it would have to be manually retrieved from the database.
Request body:
Success response: 200 status code
The library is using OAuth mechanism to authorize users for accessing certain API resources. This mechanism allows API requests to be executed on behalf of a user using an access token. This token is issued by a certain token provider when the user is logged in / authenticated. The Orion application acts as the token provider in this scenario, and users can request for a token in two ways, by submitting login credentials, or by submitting a Facebook token.
FAQs
Config based REST API framework
The npm package orion-api receives a total of 0 weekly downloads. As such, orion-api popularity was classified as not popular.
We found that orion-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.