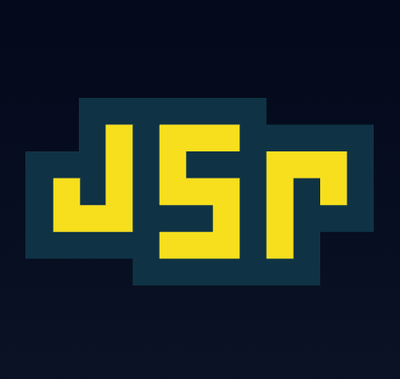
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Routing engine for OpenStreetMap data implementing high-performance algorithms for shortest paths in road networks.
Provides bindings to the Open Source Routing Machine - OSRM.
By default, binaries are provided for:
On those platforms no external dependencies are needed.
Just do:
npm install osrm
However other platforms will fall back to a source compile: see Source Build for details.
See the example/server.js
and test/osrm.test.js
for examples of using OSRM through this Node.js API.
The node-osrm
module consumes data processed by OSRM core.
This repository contains a Makefile that does this automatically:
osrm-extract
and osrm-prepare
Just run:
make berlin-latest.osrm.hsgr
Once that is done then you can calculate routes in Javascript like:
// Note: to require osrm locally do:
// require('./lib/osrm.js')
var OSRM = require('osrm')
var osrm = new OSRM("berlin-latest.osrm");
osrm.locate([52.4224,13.333086], function (err, result) {
console.log(result);
// Output: {"status":0,"mapped_coordinate":[52.422442,13.332101]}
});
osrm.nearest([52.4224, 13.333086], function (err, result) {
console.log(result);
// Output: {"status":0,"mapped_coordinate":[52.422590,13.333838],"name":"Mariannenstraße"}
});
var query = {coordinates: [[52.519930,13.438640], [52.513191,13.415852]]};
osrm.route(query, function (err, result) {
console.log(result);
/* Output:
{ status: 0,
status_message: 'Found route between points',
route_geometry: '{~pdcBmjfsXsBrD{KhS}DvHyApCcf@l}@kg@z|@_MbX|GjHdXh^fm@dr@~\\l_@pFhF|GjCfeAbTdh@fFqRp}DoEn\\cHzR{FjLgCnFuBlG{AlHaAjJa@hLXtGnCnKtCnFxCfCvEl@lHBzA}@vIoFzCs@|CcAnEQ~NhHnf@zUpm@rc@d]zVrTnTr^~]xbAnaAhSnPgJd^kExPgOzk@maAx_Ek@~BuKvd@cJz`@oAzFiAtHvKzAlBXzNvB|b@hGl@Dha@zFbGf@fBAjQ_AxEbA`HxBtPpFpa@rO_Cv_B_ZlD}LlBGB',
route_instructions:
[ ... ],
route_summary:
{ total_distance: 2814,
total_time: 211,
start_point: 'Friedenstraße',
end_point: 'Am Köllnischen Park' },
alternative_geometries: [],
alternative_instructions: [],
alternative_summaries: [],
route_name:
[ 'Lichtenberger Straße',
'Holzmarktstraße' ],
alternative_names: [ [ '', '' ] ],
via_points:
[ [ 52.519934, 13.438647 ],
[ 52.513162, 13.415509 ] ],
via_indices: [ 0, 69 ],
alternative_indices: [],
hint_data:
{ checksum: 222545162,
locations:
[ '9XkCAJgBAAAtAAAA____f7idcBkPGuw__mMhA7cOzQA',
'TgcEAFwFAAAAAAAAVAAAANIeb5DqBHs_ikkhA1W0zAA' ] } }
*/
});
To build from source you will need:
develop
branch, cloned from github.-DWITH_TOOLS=1
so that libOSRM
is createdTo build the bindings you need to first build and install the develop
branch of Project-OSRM
:
# grab develop branch
git clone -b develop https://github.com/DennisOSRM/Project-OSRM.git
cd Project-OSRM
mkdir build;
cd build;
cmake ../ -DWITH_TOOLS=1
make
sudo make install
NOTE: If you hit problems building Project-OSRM see the wiki for details.
Then build node-osrm
against Project-OSRM
installed in /usr/local
:
git clone https://github.com/DennisOSRM/node-osrm.git
cd node-osrm
npm install --build-from-source
Developers of node-osrm
should set up a Source Build and after changes to the code run:
make
Under the hood this uses node-gyp to compile the source code.
Run the tests like:
make test
Releasing a new version of node-osrm
is mostly automated using travis.ci.
If you create and push a new git tag Travis.ci will automatically publish both binaries and the package to the npm registry.
Before tagging you can test publishing of just the binaries by including the keyword [publish binary]
in your commit message. Make sure to run node-pre-gyp unpublish
before trying publish binaries for a version that has already been published as trying to publish over an existing binary will fail.
1) Confirm the desired OSRM branch and commit.
This is configurable via the OSRM_BRANCH
and OSRM_COMMIT
variables in travis.ci.
See Issue 36 for further ideas on streamlining this.
2) Bump node-osrm version
Update the CHANGELOG.md
and the package.json
version.
3) Check Travis.ci
Ensure Travis.ci builds are passing after your last commit. This is important because upstream OSRM is being pulled in and may have changed.
4) Tag
Tag a new release:
git add CHANGELOG.md package.json
git commit -m "Tagging v0.2.8"
git tag v0.2.8
5) Push the tag to github:
git push origin master v0.2.8
This will trigger travis.ci to build Ubuntu binaries and publish the entire package to the npm registry upon success. The publishing will use the s3 and npm auth credentials of @springmeyer currently - this needs to be made more configurable in the future.
6) Merge into the osx
branch
git checkout osx
git merge v0.2.8 -m "[publish binary]"
git push origin osx
This will build and publish OS X binaries on travis.ci. Be prepared to watch the travis run and re-start builds that fail due to timeouts (the OS X machines are underpowered).
7) Check published binaries
If travis builds passed for both the master
branch and the osx
branch then binaries should be published for both platforms.
Confirm the remote binaries are available with node-pre-gyp:
$ npm install node-pre-gyp # or use the copy in ./node_modules/.bin
$ node-pre-gyp info --loglevel silent | grep `node -e "console.log(require('./package.json').version)"`
osrm-v0.2.8-node-v11-darwin-x64.tar.gz
osrm-v0.2.8-node-v11-linux-x64.tar.gz
osrm-v0.2.8-v8-3.11-darwin-x64.tar.gz
osrm-v0.2.8-v8-3.11-linux-x64.tar.gz
9) Publish node-osrm
Publish node-osrm
npm publish
Dependent apps can now pull from the npm registry like:
"dependencies": {
"osrm": "~0.2.8"
}
Or can still pull from the github tag like:
"dependencies": {
"osrm": "https://github.com/DennisOSRM/node-osrm/tarball/v0.2.8"
}
FAQs
The Open Source Routing Machine is a high performance routing engine written in C++14 designed to run on OpenStreetMap data.
We found that osrm demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 21 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.