overload2
Elegant solution for function overload in JavaScript.
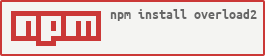
Tied with writing tasteless code to do with arguments? Use overload2 to make things easy.
On programming with strongly-typed language, such as C++ and Java, function overloading is frequently employed to make API more convenient to be used. As a weakly-typed language, JavaScript does not support function overloading. At the same time, fortunately, functions in JavaScript may be passed with any arguments that is why overload2 is feasible.
Table of contents
See CHANGE LOG for notable changes. Or access project's homepage for latest updates.
Get Started
Install overload2 firstly.
npm install -g overload2
Open node and run next code:
const overload2 = require('overload2');
var getDay = overload2()
.overload(
Date,
function foo(d) { return d.getDay(); }
)
.overload(
'string',
function bar(s) { return new Date(s).getDay(); }
)
.overload(
'number', 'number', 'number',
function quz(year, month, date) { return new Date(year, month - 1, date).getDay(); }
)
.overload(
4,
function four() { console.log('too many arguments'); }
)
;
getDay(new Date);
getDay('2000-1-1');
getDay(2000, 1, 1);
getDay(1, 2, 3, 4);
Datatypes
According to overload2 , there are different ways to define a datatype.
Constructor Function
overload2 can match any instance with its constructor function, e.g
var getDay = overlaod2()
.overload(Date, function foo(d) { return d.getDay(); })
.overload(String, function bar(s) { return new Date(s).getDay(); })
;
getDay(new Date);
getDay(new String('2000-1-1'));
for example [0,1]
is matched with Array
.
Customized Datatype
You may create customized datatypes by new overload2.Datatype(fn)
, e.g.m
var DAYNAME = new overload2.Type(function(value) {
var names = ['sun', 'mon', 'tue', 'wed', 'thu', 'fri', 'sat'];
return names.indexOf(value) >= 0;
});
Predefined Datatype
Predefined Data Type | Remark |
---|
overload2.ANY | Anything. |
overload2.BOOLEAN | It must be true or false , anything else including instance of Boolean is unmatched. |
overload2.CHAR | A string whose length equals 1, e.g. "a" |
overload2.NUMBER | A number, but NOT instance of Number . |
overload2.SCALAR | A number, string or boolean, but NOT instance of Number`, Stringor Boolean``. |
overload2.STRING | A string, but NOT instance of String . |
Datatype Alias
ATTENTION: Datatype aliases are CaseSensitive.
Alias | Corresponding Datetype |
---|
* | overload2.ANY |
any | overload2.ANY |
boolean | overload2.BOOLEAN |
char | overload2.CHAR |
number | overload2.NUMBER |
scalar | overload2.SCALAR |
string | overload2.STRING |
Create Datatype With Factory Method
overload2 offers some factory methods to create frequently used datatypes, e.g. enum.
- overload2.enum(item[, ...])
Return Datatype instance
Move Forward
Beyond the basic use, overload2 is also suitable with more complex and large-scale programs. See the class hierarchy shown below:

The usage of classes in overload2 is explained in the next table:
Class | Remark |
---|
overload2.OverloadedFunction | wrapper of overloaded function, not a function instance itself |
overload2.Overload | to define overloading implementation |
overload2.ParamList | to define a parameter list |
overload2.Param | to define a parameter |
overload2.Type | wrapper of class (consturctor function), or to customise some datatype |
Instances of Type
, Param
, ParamList
and Overload
are able to be created independently and be re-used in creating instances of superior class(es).
Here is an example for advanced mode.
APIs
Overloaded Function Instance
overload2
itself is a function, when invoked, it will return an overloded function instance.
-
<fn> overload2( [ <datatype>, ... ] function <implementation> )
Create a new overloaded function.
-
<fn> <fn>.overload( [ <datatype>, ... ] function <implementation> )
Append an overloading implementation to existing overloaded function.
-
<fn> <fn>.default( function <implementation> )
Set default implementation function for existing overloaded function.
overload2.Type
To define a datatype in context of overload2, there are different ways including overload2.Type
. And all other datatypes will be converted to instances of overload2.Type
before being used.
-
new overload2.Type( function | RegExp <matcher> )
Here matcher
may be a function or RegExp object.
-
private boolean <type>.match( <value> )
Return true
if value matches the datatype, otherwise return false
.
overload2.Param
A Param is made up of a Type and some decorators. Available decorators are:
Decorator | Remark |
---|
null | If argument equals null, it matches the parameter. |
undefined | If argument equals undefined (the place should be occupied), it matches the parameter. |
-
new overload2.Param( string "<alias> <decorator> ..." )
The alias
should be one of alias listed in table Datatype Alias.
-
new overload2.Param( Type | function | string <datatype>, string <decorator(s)> [ , string <decorator(s)> ] )
Here datatype
may be instance of Type
, or construtor function, or datatype alias.
-
private boolean <param>.satisfy( <value> )
To judge if the argument value satisfy the parameter.
-
<Param> overload2.Param.parse( ? )
Arguments suitable for new Param()
are also suitable for the Param.parse()
.
overload2.ParamList
-
new overload2.ParamList( [ Param | Array | String <param> [ , ... ] ] )
Here param
may be an instance of Param
, or a string or an array which may used as argument(s) for new Param()
.
-
private boolean <paramList>.satisfy( Array | Arguments <args> )
To check arguments with parameters, return true
if matched or false
if not.
-
<ParamList> overload2.ParamList.parse( ? )
Arguments suitable for new ParamList()
are also suitable for the ParamList.parse()
.
overload2.Overload
-
new overload2.Overload( number , function <implementation> )
-
new overload2.Overload( ParamList, function <implementation> )
-
new overload2.Overload( [ <param>, [ ..., ] ] function <implementation> )
-
<Overload> overload2.Overload.parse( ? )
Arguments suitable for new Overload()
are also suitable for the Overload.parse()
.
overload2.OverloadedFunction
-
new overload2.OverloadedFunction()
The instance of OverloadedFunction
is a wrapper, not a function itself.
-
<wrapper>.exec( ... )
Run the overloaded function.
-
<wrapper>.apply( <scope>, Array | Arguments <args> )
Run the overloaded function under specified scope, passing arguments as an array or Arguments instance.
-
<wrapper>.call( <scope> [ , <arg> [ , ... ] ] )
Run the overloaded function under specified scope, passing arguments one by one.
-
<wrapper>.overload( Overload <overloadInstance> )
Append an overloading implementation.
-
<wrapper>.overload( ? )
Append an overloading implementation, arguments suitable for new Overload()
are also suitable for the <wrapper>.overload()
.
Examples
Why overload2
To be continued.