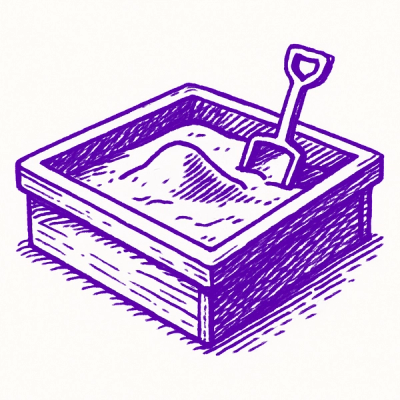
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
p-cancelable
Advanced tools
The p-cancelable package provides a way to create cancelable promises in JavaScript. This is particularly useful for managing asynchronous operations that may need to be canceled before they complete, such as HTTP requests, long-running computations, or any other task that might not need to continue executing if certain conditions are met.
Creating a cancelable promise
This feature allows the creation of a new cancelable promise. The promise can be canceled at any time before it resolves or rejects, which triggers the onCancel function where cleanup operations like clearing timeouts or aborting requests can be performed.
const PCancelable = require('p-cancelable');
const myCancelablePromise = new PCancelable((resolve, reject, onCancel) => {
const timer = setTimeout(() => {
resolve('Done!');
}, 2000);
onCancel(() => {
clearTimeout(timer);
reject(new Error('Operation canceled.'));
});
});
myCancelablePromise.cancel();
Bluebird is a comprehensive promise library that includes support for cancelable promises. Compared to p-cancelable, Bluebird offers a broader set of promise-related utilities, such as map, reduce, and filter for promises, making it suitable for more complex scenarios.
The abort-controller package provides a way to abort one or more Web API tasks, such as Fetch API requests, by using an AbortSignal. While it serves a similar purpose for canceling tasks, it is specifically designed to work with the Fetch API and other APIs that support aborting, unlike p-cancelable which is more generic.
Create a promise that can be canceled
Useful for animation, loading resources, long-running async computations, async iteration, etc.
If you target Node.js 16 or later, this package is less useful and you should probably use AbortController
instead.
npm install p-cancelable
import PCancelable from 'p-cancelable';
const cancelablePromise = new PCancelable((resolve, reject, onCancel) => {
const worker = new SomeLongRunningOperation();
onCancel(() => {
worker.close();
});
worker.on('finish', resolve);
worker.on('error', reject);
});
// Cancel the operation after 10 seconds
setTimeout(() => {
cancelablePromise.cancel('Unicorn has changed its color');
}, 10000);
try {
console.log('Operation finished successfully:', await cancelablePromise);
} catch (error) {
if (cancelablePromise.isCanceled) {
// Handle the cancelation here
console.log('Operation was canceled');
return;
}
throw error;
}
Same as the Promise
constructor, but with an appended onCancel
parameter in executor
.
Cancelling will reject the promise with CancelError
. To avoid that, set onCancel.shouldReject
to false
.
import PCancelable from 'p-cancelable';
const cancelablePromise = new PCancelable((resolve, reject, onCancel) => {
const job = new Job();
onCancel.shouldReject = false;
onCancel(() => {
job.stop();
});
job.on('finish', resolve);
});
cancelablePromise.cancel(); // Doesn't throw an error
PCancelable
is a subclass of Promise
.
Type: Function
Accepts a function that is called when the promise is canceled.
You're not required to call this function. You can call this function multiple times to add multiple cancel handlers.
Type: Function
Cancel the promise and optionally provide a reason.
The cancellation is synchronous. Calling it after the promise has settled or multiple times does nothing.
Type: boolean
Whether the promise is canceled.
Convenience method to make your promise-returning or async function cancelable.
The function you specify will have onCancel
appended to its parameters.
import PCancelable from 'p-cancelable';
const fn = PCancelable.fn((input, onCancel) => {
const job = new Job();
onCancel(() => {
job.cleanup();
});
return job.start(); //=> Promise
});
const cancelablePromise = fn('input'); //=> PCancelable
// …
cancelablePromise.cancel();
Type: Error
Rejection reason when .cancel()
is called.
It includes a .isCanceled
property for convenience.
In American English, the verb cancel is usually inflected canceled and canceling—with one l. Both a browser API and the Cancelable Promises proposal use this spelling.
It's still an early draft and I don't really like its current direction. It complicates everything and will require deep changes in the ecosystem to adapt to it. And the way you have to use cancel tokens is verbose and convoluted. I much prefer the more pragmatic and less invasive approach in this module. The proposal was withdrawn.
Available as part of the Tidelift Subscription.
The maintainers of p-cancelable and thousands of other packages are working with Tidelift to deliver commercial support and maintenance for the open source dependencies you use to build your applications. Save time, reduce risk, and improve code health, while paying the maintainers of the exact dependencies you use. Learn more.
.then()
or .catch()
is calledFAQs
Create a promise that can be canceled
The npm package p-cancelable receives a total of 21,839,129 weekly downloads. As such, p-cancelable popularity was classified as popular.
We found that p-cancelable demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.