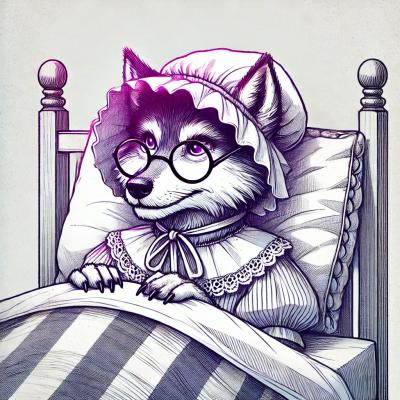
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The p-limit npm package is a utility that allows you to limit the number of promises that are running at the same time. It is useful for controlling concurrency when you have operations that can be run in parallel but you want to limit the number of these operations due to resource constraints.
Concurrency Limiting
This feature allows you to create a limit for how many promises are allowed to run at once. In the code sample, the limit is set to 1, meaning that `doSomething` and `doAnotherThing` will not run at the same time.
const pLimit = require('p-limit');
const limit = pLimit(1);
async function doSomething() {}
async function doAnotherThing() {}
// Only one promise will run at once
const result1 = limit(() => doSomething());
const result2 = limit(() => doAnotherThing());
Queueing
This feature demonstrates how additional promises are queued when the limit is reached. In this example, only two promises will run concurrently, and the rest will wait in the queue.
const pLimit = require('p-limit');
const limit = pLimit(2);
const input = [
limit(() => fetchSomething('foo')),
limit(() => fetchSomething('bar')),
limit(() => doSomethingElse()),
];
// Only two promises will run at once, the rest will be queued
Promise.all(input).then(results => {
console.log(results);
});
Bottleneck is a powerful rate limiter that allows you to limit the number of calls to a function. It can be used to throttle requests to an API or any other operation that needs to be rate-limited. It is more feature-rich than p-limit, providing priorities, clustering support, and more configuration options.
Async provides a collection of utilities for working with asynchronous JavaScript. While it offers functions like `async.parallelLimit` which can limit the number of asynchronous operations running in parallel, it is a more comprehensive toolkit for asynchronous control flow, including series, waterfall, and eachOf methods.
P-Queue is a promise queue with adjustable concurrency that supports priorities, timeouts, and pausing. It is similar to p-limit but offers a higher level of abstraction with a queue system, making it suitable for more complex scenarios where you need to manage the order and priority of tasks.
Run multiple promise-returning & async functions with limited concurrency
$ npm install p-limit
const pLimit = require('p-limit');
const limit = pLimit(1);
const input = [
limit(() => fetchSomething('foo')),
limit(() => fetchSomething('bar')),
limit(() => doSomething())
];
(async () => {
// Only one promise is run at once
const result = await Promise.all(input);
console.log(result);
})();
Returns a limit
function.
Type: number
Minimum: 1
Concurrency limit.
Returns the promise returned by calling fn(...args)
.
Type: Function
Promise-returning/async function.
Any arguments to pass through to fn
.
Support for passing arguments on to the fn
is provided in order to be able to avoid creating unnecessary closures. You probably don't need this optimization unless you're pushing a lot of functions.
MIT © Sindre Sorhus
FAQs
Run multiple promise-returning & async functions with limited concurrency
The npm package p-limit receives a total of 0 weekly downloads. As such, p-limit popularity was classified as not popular.
We found that p-limit demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.