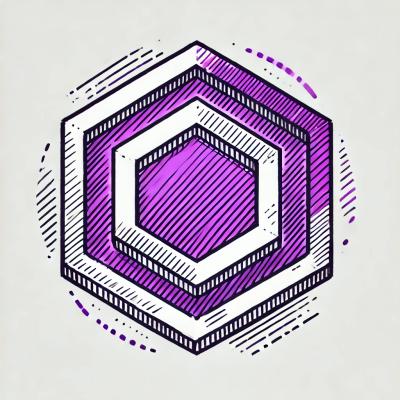
Security News
ESLint is Now Language-Agnostic: Linting JSON, Markdown, and Beyond
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
The p-timeout package is a utility that allows you to wrap a Promise with a timeout, after which a specified exception is thrown if the promise does not resolve or reject before the timeout period elapses. This is useful for ensuring that your application can handle situations where a Promise might take too long to complete, or when you want to enforce a strict timing policy for certain operations.
Timeout a Promise
This feature allows you to set a timeout for a Promise. If the Promise does not resolve or reject within the specified time, it will be rejected with a TimeoutError.
const pTimeout = require('p-timeout');
const doSomething = () => new Promise(resolve => setTimeout(resolve, 2000));
pTimeout(doSomething(), 1000).then(() => console.log('Resolved')).catch(error => console.log('Timed out:', error.message));
Custom Timeout Error
This feature allows you to specify a custom error message for the timeout. If the Promise times out, it will be rejected with a TimeoutError that contains your custom message.
const pTimeout = require('p-timeout');
const TimeoutError = pTimeout.TimeoutError;
const doSomething = () => new Promise(resolve => setTimeout(resolve, 2000));
pTimeout(doSomething(), 1000, 'Operation timed out').then(() => console.log('Resolved')).catch(error => {
if (error instanceof TimeoutError) {
console.log('Custom timeout message:', error.message);
}
});
Clearing the Timeout
This feature allows you to clear the timeout before it occurs. This is useful if the conditions of your operation change and you no longer require the timeout.
const pTimeout = require('p-timeout');
const doSomething = () => new Promise(resolve => setTimeout(resolve, 2000));
const promiseWithTimeout = pTimeout(doSomething(), 1000);
promiseWithTimeout.then(() => console.log('Resolved')).catch(error => console.log('Timed out:', error.message));
// Later, if you decide you no longer want the timeout:
pTimeout.clear(promiseWithTimeout);
The promise-timeout package offers similar functionality to p-timeout, allowing you to add a timeout to a Promise. It differs in API design and error handling specifics.
Bluebird is a full-featured Promise library that includes a .timeout() method for timing out promises. It is more comprehensive than p-timeout but also larger in size and scope.
The async-timeout package is similar to p-timeout but is designed specifically for use with async functions and the async/await syntax.
Timeout a promise after a specified amount of time
$ npm install p-timeout
const delay = require('delay');
const pTimeout = require('p-timeout');
const delayedPromise = delay(200);
pTimeout(delayedPromise, 50).then(() => 'foo');
//=> [TimeoutError: Promise timed out after 50 milliseconds]
Returns a decorated input
that times out after milliseconds
time. It has a .clear()
method that clears the timeout.
If you pass in a cancelable promise, specifically a promise with a .cancel()
method, that method will be called when the pTimeout
promise times out.
Type: Promise
Promise to decorate.
Type: number
Milliseconds before timing out.
Passing Infinity
will cause it to never time out.
Type: string | Error
Default: 'Promise timed out after 50 milliseconds'
Specify a custom error message or error.
If you do a custom error, it's recommended to sub-class pTimeout.TimeoutError
.
Type: Function
Do something other than rejecting with an error on timeout.
You could for example retry:
const delay = require('delay');
const pTimeout = require('p-timeout');
const delayedPromise = () => delay(200);
pTimeout(delayedPromise(), 50, () => {
return pTimeout(delayedPromise(), 300);
});
Type: object
Type: object
with function properties setTimeout
and clearTimeout
Custom implementations for the setTimeout
and clearTimeout
functions.
Useful for testing purposes, in particular to work around sinon.useFakeTimers()
.
Example:
const pTimeout = require('p-timeout');
const sinon = require('sinon');
(async () => {
const originalSetTimeout = setTimeout;
const originalClearTimeout = clearTimeout;
sinon.useFakeTimers();
// Use `pTimeout` without being affected by `sinon.useFakeTimers()`:
await pTimeout(doSomething(), 2000, undefined, {
customTimers: {
setTimeout: originalSetTimeout,
clearTimeout: originalClearTimeout
}
});
})();
Exposed for instance checking and sub-classing.
FAQs
Timeout a promise after a specified amount of time
The npm package p-timeout receives a total of 10,291,526 weekly downloads. As such, p-timeout popularity was classified as popular.
We found that p-timeout demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
Security News
Members Hub is conducting large-scale campaigns to artificially boost Discord server metrics, undermining community trust and platform integrity.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.