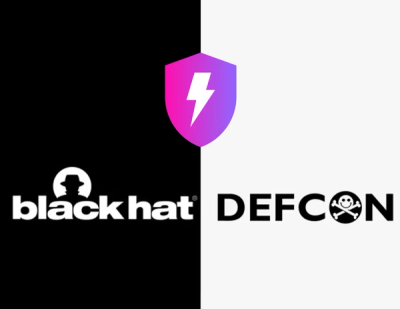
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
packageurl-js
Advanced tools
JavaScript library to parse and build "purl" aka. package URLs. This is a microlibrary implementing the purl spec at https://github.com/package-url
The packageurl-js npm package is a JavaScript implementation of the Package URL (purl) specification. It allows you to parse, construct, and manipulate package URLs, which are used to identify and locate software packages in a standardized way.
Parsing a Package URL
This feature allows you to parse a package URL string into its components. The code sample demonstrates how to parse a package URL for the Angular core package.
const { PackageURL } = require('packageurl-js');
const purl = PackageURL.fromString('pkg:npm/%40angular/core@12.0.0');
console.log(purl);
Constructing a Package URL
This feature allows you to construct a package URL from its components. The code sample demonstrates how to create a package URL for the Angular core package.
const { PackageURL } = require('packageurl-js');
const purl = new PackageURL('npm', '@angular', 'core', '12.0.0', null, null);
console.log(purl.toString());
Manipulating a Package URL
This feature allows you to manipulate the components of a package URL. The code sample demonstrates how to change the version of an existing package URL.
const { PackageURL } = require('packageurl-js');
let purl = new PackageURL('npm', '@angular', 'core', '12.0.0', null, null);
purl.version = '12.1.0';
console.log(purl.toString());
The url-parse npm package is a more general-purpose URL parsing library. While it can handle package URLs, it is not specifically designed for them and lacks some of the specialized features of packageurl-js.
The parse-package-name npm package focuses on parsing package names and versions from strings. It provides some similar functionality but is more limited in scope compared to packageurl-js.
To install packageurl-js
in your project, simply run:
npm install packageurl-js
This command will download the packageurl-js
npm package for use in your application.
Clone the packageurl-js
repo and cd
into the directory.
Then run:
npm install
To run the test suite:
npm test
As an ES6 module
import { PackageURL } from 'packageurl-js'
As a CommonJS module
const { PackageURL } = require('packageurl-js')
const purlStr = 'pkg:maven/org.springframework.integration/spring-integration-jms@5.5.5'
console.log(PackageURL.fromString(purlStr))
console.log(new PackageURL(...PackageURL.parseString(purlStr)))
will both log
PackageURL {
type: 'maven',
name: 'spring-integration-jms',
namespace: 'org.springframework.integration',
version: '5.5.5',
qualifiers: undefined,
subpath: undefined
}
const pkg = new PackageURL(
'maven',
'org.springframework.integration',
'spring-integration-jms',
'5.5.5'
)
console.log(pkg.toString())
=>
pkg:maven/org.springframework.integration/spring-integration-jms@5.5.5
try {
PackageURL.fromString('not-a-purl')
} catch (e) {
console.error(e.message)
}
=>
Invalid purl: missing required "pkg" scheme component
Helpers for encoding, normalizing, and validating purl components and types can be imported directly from the module or found on the PackageURL class as static properties.
import {
PackageURL,
PurlComponent,
PurlType
} from 'packageurl-js'
PurlComponent === PackageURL.Component // => true
PurlType === PackageURL.Type // => true
Contains the following properties each with their own encode
, normalize
,
and validate
methods, e.g. PurlComponent.name.validate(nameStr)
:
Contains the following properties each with their own normalize
, and validate
methods, e.g. PurlType.npm.validate(purlObj)
:
FAQs
JavaScript library to parse and build "purl" aka. package URLs. This is a microlibrary implementing the purl spec at https://github.com/package-url
The npm package packageurl-js receives a total of 1,005,973 weekly downloads. As such, packageurl-js popularity was classified as popular.
We found that packageurl-js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.