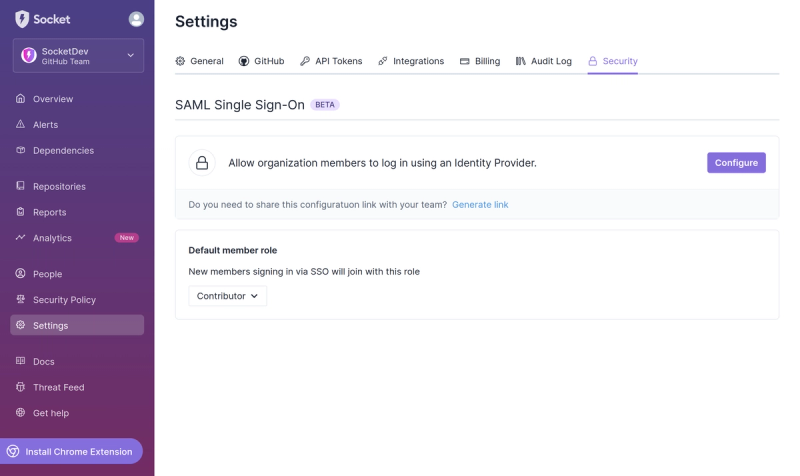
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
parse-asn1
Advanced tools
Package description
The parse-asn1 npm package is used for parsing ASN.1 (Abstract Syntax Notation One) data. ASN.1 is a standard interface description language for defining data structures that can be serialized and deserialized in a cross-platform way. This package is particularly useful for dealing with cryptographic formats, as many cryptographic protocols use ASN.1 to define the structure of keys and certificates.
Parsing ASN.1 DER
This feature allows you to parse ASN.1 DER (Distinguished Encoding Rules) encoded data. The code sample reads a DER-encoded file (e.g., a certificate) from the filesystem and uses parse-asn1 to parse it into a JavaScript object.
const parseAsn1 = require('parse-asn1');
const fs = require('fs');
const der = fs.readFileSync('certificate.der');
const certificate = parseAsn1(der);
console.log(certificate);
Extracting Public Key
This feature is used to extract a public key from a DER-encoded file. The code sample demonstrates how to read the DER file and parse it to obtain the public key information.
const parseAsn1 = require('parse-asn1');
const fs = require('fs');
const der = fs.readFileSync('publicKey.der');
const publicKey = parseAsn1(der);
console.log(publicKey);
Parsing Private Key
This feature enables the parsing of a private key from a DER-encoded file. The code sample shows how to read the DER file and use parse-asn1 to parse the private key.
const parseAsn1 = require('parse-asn1');
const fs = require('fs');
const der = fs.readFileSync('privateKey.der');
const privateKey = parseAsn1(der);
console.log(privateKey);
asn1.js is a library that provides a similar functionality to parse-asn1. It allows for encoding and decoding ASN.1 data structures using JavaScript. Compared to parse-asn1, asn1.js offers a more comprehensive API for building and deconstructing ASN.1 elements programmatically.
node-forge is a comprehensive toolkit for cryptography in JavaScript. It includes functionality for parsing ASN.1 data, but also provides a wide range of cryptographic operations such as encryption, decryption, signing, and verification. It is more extensive than parse-asn1, which focuses primarily on ASN.1 parsing.
pkijs is a set of JavaScript libraries that work with Public Key Infrastructure (PKI) using ASN.1. It is built on top of the Web Cryptography API and provides a higher-level API for working with certificates, certificate requests, and other PKI-related functionalities. It is more specialized for PKI operations compared to the general ASN.1 parsing provided by parse-asn1.
Readme
FAQs
utility library for parsing asn1 files for use with browserify-sign.
The npm package parse-asn1 receives a total of 7,023,615 weekly downloads. As such, parse-asn1 popularity was classified as popular.
We found that parse-asn1 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.