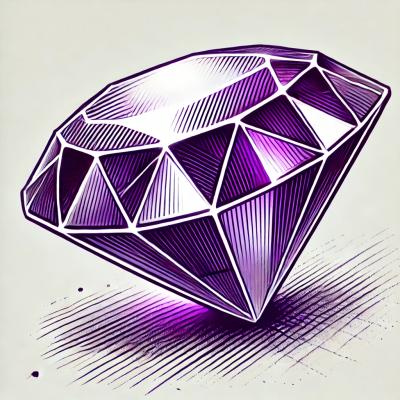
Security News
Highlights from the 2024 Rails Community Survey
A record 2,709 developers participated in the 2024 Ruby on Rails Community Survey, revealing key tools, practices, and trends shaping the Rails ecosystem.
parse-domain
Advanced tools
The parse-domain npm package is used to parse domain names into their constituent parts, such as the top-level domain (TLD), second-level domain (SLD), and subdomain. This can be useful for various applications, including URL validation, domain name extraction, and more.
Basic Domain Parsing
This feature allows you to parse a domain name into its constituent parts. The code sample demonstrates how to parse 'www.example.com' into its subdomain, domain, and TLD.
const parseDomain = require('parse-domain');
const parsed = parseDomain('www.example.com');
console.log(parsed);
Handling Different TLDs
This feature allows you to handle domains with different TLDs, including country-code TLDs. The code sample demonstrates parsing 'example.co.uk' into its parts.
const parseDomain = require('parse-domain');
const parsed = parseDomain('example.co.uk');
console.log(parsed);
Parsing URLs with Protocols
This feature allows you to parse full URLs, including the protocol and path, to extract the domain parts. The code sample demonstrates parsing 'https://www.example.com/path'.
const parseDomain = require('parse-domain');
const parsed = parseDomain('https://www.example.com/path');
console.log(parsed);
The tldjs package provides similar functionality to parse-domain, allowing you to parse domain names and extract TLDs, SLDs, and subdomains. It also offers additional features like checking if a domain is valid and extracting the public suffix.
The psl package is another alternative that focuses on parsing domain names based on the Public Suffix List. It provides methods to get the domain, subdomain, and TLD, and is often used for more advanced domain parsing needs.
The url-parse package is a more general URL parsing library that can also extract domain parts. While it offers broader URL parsing capabilities, it may not be as specialized in domain parsing as parse-domain.
Splits a URL into sub-domain, domain and the effective top-level domain.
Since domains are handled differently across different countries and organizations, splitting a URL into sub-domain, domain and top-level-domain parts is not a simple regexp. parse-domain uses a large list of effective tld names from publicsuffix.org to recognize different parts of the domain.
Please also read the note on effective top-level domains.
npm install --save parse-domain
// long subdomains can be handled
expect(parseDomain("some.subdomain.example.co.uk")).to.eql({
subdomain: "some.subdomain",
domain: "example",
tld: "co.uk"
});
// usernames, passwords and ports are disregarded
expect(parseDomain("https://user:password@example.co.uk:8080/some/path?and&query#hash")).to.eql({
subdomain: "",
domain: "example",
tld: "co.uk"
});
// non-canonical top-level domains are ignored
expect(parseDomain("unknown.tld.kk")).to.equal(null);
// invalid urls are also ignored
expect(parseDomain("invalid url")).to.equal(null);
expect(parseDomain({})).to.equal(null);
// custom top-level domains can optionally be specified
expect(parseDomain("mymachine.local",{ customTlds: ["local"] })).to.eql({
subdomain: "",
domain: "mymachine",
tld: "local"
});
// custom regexps can optionally be specified (instead of customTlds)
expect(parseDomain("localhost",{ customTlds:/localhost|\.local/ })).to.eql({
subdomain: "",
domain: "",
tld: "localhost"
});
It can sometimes be helpful to apply the customTlds argument using a helper function
function parseLocalDomains(url) {
var options = {
customTlds: /localhost|\.local/
};
return parseDomain(url, options);
}
expect(parseLocalDomains("localhost")).to.eql({
subdomain: "",
domain: "",
tld: "localhost"
});
expect(parseLocalDomains("mymachine.local")).to.eql({
subdomain: "",
domain: "mymachine",
tld: "local"
});
parseDomain(url: String, options: ParseOptions): ParsedDomain|null
Returns null
if url
has an unknown tld or if it's not a valid url.
ParseOptions
{
customTlds: RegExp|String[]
}
ParsedDomain
{
tld: String,
domain: String,
subdomain: String
}
Technically, the top-level domain is always the part after the last dot. That's why publicsuffix.org is a list of effective top-level domains: It lists all top-level domains where users are allowed to host any content. That's why foo.blogspot.com
will be split into
{
tld: "blogspot.com",
domain: "foo",
subdomain: ""
}
See also #4
Unlicense
FAQs
Splits a hostname into subdomains, domain and (effective) top-level domains
The npm package parse-domain receives a total of 153,389 weekly downloads. As such, parse-domain popularity was classified as popular.
We found that parse-domain demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A record 2,709 developers participated in the 2024 Ruby on Rails Community Survey, revealing key tools, practices, and trends shaping the Rails ecosystem.
Security News
In 2023, data breaches surged 78% from zero-day and supply chain attacks, but developers are still buried under alerts that are unable to prevent these threats.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.