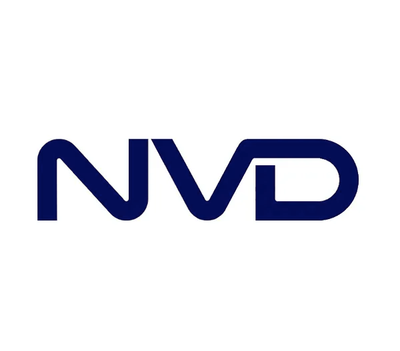
Security News
NIST Misses 2024 Deadline to Clear NVD Backlog
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
parse-path
Advanced tools
The parse-path npm package is a utility for parsing URLs and file paths into their constituent parts. It can handle a variety of path formats and provides a structured way to access different components of a path.
Parse URL
This feature allows you to parse a URL into its components such as protocol, host, port, pathname, query, and hash.
const parsePath = require('parse-path');
const parsedUrl = parsePath('https://example.com:8080/path/name?query=string#hash');
console.log(parsedUrl);
Parse File Path
This feature allows you to parse a file path into its components such as root, dir, base, ext, and name.
const parsePath = require('parse-path');
const parsedFilePath = parsePath('/home/user/docs/file.txt');
console.log(parsedFilePath);
Handle Different Path Formats
This feature allows you to handle different path formats, including Windows-style paths, and parse them into their components.
const parsePath = require('parse-path');
const parsedPath = parsePath('C:\Users\user\docs\file.txt');
console.log(parsedPath);
The url-parse package is a robust URL parser that works in both Node.js and the browser. It provides similar functionality to parse-path but is more focused on URLs rather than file paths. It offers additional features like URL normalization and query string parsing.
The path-parse package is a simple utility for parsing file paths into their components. It is similar to parse-path but is more focused on file paths rather than URLs. It provides a straightforward way to access different parts of a file path.
The whatwg-url package is a full implementation of the WHATWG URL Standard. It provides comprehensive URL parsing and manipulation capabilities, making it more feature-rich compared to parse-path. However, it is more complex and may be overkill for simple use cases.
FAQs
Parse paths (local paths, urls: ssh/git/etc)
The npm package parse-path receives a total of 2,982,513 weekly downloads. As such, parse-path popularity was classified as popular.
We found that parse-path demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.