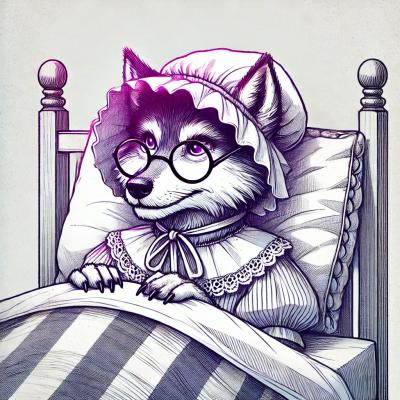
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The parse-url npm package is used to parse URLs and extract components such as protocol, auth, host, pathname, query parameters, and hash. It provides a simple API to break down a URL string into its constituent parts for easy access and manipulation.
Parsing the URL
This feature allows you to parse a full URL and returns an object with properties like protocol, resource, user, pathname, hash, search, and query. Each property represents a specific part of the URL.
const parseUrl = require('parse-url');
const parsed = parseUrl('https://www.example.com:8080/path/name?query=value#hash');
console.log(parsed);
Parsing the Query String
This feature specifically focuses on parsing the query string part of the URL and converting it into an object for easy access to each query parameter.
const parseUrl = require('parse-url');
const parsed = parseUrl('https://www.example.com/path/name?query=value&another=thing');
console.log(parsed.query);
Reconstructing the URL
After parsing a URL and potentially modifying its components, this feature allows you to reconstruct the URL back into a string.
const parseUrl = require('parse-url');
const parsed = parseUrl('https://www.example.com/path/name');
parsed.resource = 'www.another-example.com';
const reconstructedUrl = parseUrl.stringify(parsed);
console.log(reconstructedUrl);
The url-parse package offers similar functionality to parse-url, allowing for the parsing of URLs into components. It also provides the ability to resolve relative URLs against an absolute base URL, which is a feature not present in parse-url.
While qs is not a direct alternative to parse-url, it provides robust query string parsing and stringifying capabilities. It can be used in conjunction with other URL parsing libraries to handle the query string part of URLs more extensively than parse-url.
URI.js is a URL manipulation library that provides a fluent API for building, parsing, and manipulating URLs. It offers more features than parse-url, such as URL normalization, resolution of relative paths, and support for punycode and IPv6.
An advanced url parser supporting git urls too.
For low-level path parsing, check out parse-path
. This very module is designed to parse urls. By default the urls are normalized.
# Using npm
npm install --save parse-url
# Using yarn
yarn add parse-url
// Dependencies
const parseUrl = require("parse-url")
console.log(parseUrl("http://ionicabizau.net/blog"))
// {
// protocols: [ 'http' ],
// protocol: 'http',
// port: '',
// resource: 'ionicabizau.net',
// user: '',
// password: '',
// pathname: '/blog',
// hash: '',
// search: '',
// href: 'http://ionicabizau.net/blog',
// query: {}
// }
console.log(parseUrl("http://domain.com/path/name?foo=bar&bar=42#some-hash"))
// {
// protocols: [ 'http' ],
// protocol: 'http',
// port: '',
// resource: 'domain.com',
// user: '',
// password: '',
// pathname: '/path/name',
// hash: 'some-hash',
// search: 'foo=bar&bar=42',
// href: 'http://domain.com/path/name?foo=bar&bar=42#some-hash',
// query: { foo: 'bar', bar: '42' }
// }
// If you want to parse fancy Git urls, turn off the automatic url normalization
console.log(parseUrl("git+ssh://git@host.xz/path/name.git", false))
// {
// protocols: [ 'git', 'ssh' ],
// protocol: 'git',
// port: '',
// resource: 'host.xz',
// user: 'git',
// password: '',
// pathname: '/path/name.git',
// hash: '',
// search: '',
// href: 'git+ssh://git@host.xz/path/name.git',
// query: {}
// }
console.log(parseUrl("git@github.com:IonicaBizau/git-stats.git", false))
// {
// protocols: [ 'ssh' ],
// protocol: 'ssh',
// port: '',
// resource: 'github.com',
// user: 'git',
// password: '',
// pathname: '/IonicaBizau/git-stats.git',
// hash: '',
// search: '',
// href: 'git@github.com:IonicaBizau/git-stats.git',
// query: {}
// }
There are few ways to get help:
parseUrl(url, normalize)
Parses the input url.
Note: This throws if invalid urls are provided.
String url
: The input url.
Boolean|Object normalize
: Whether to normalize the url or not. Default is false
. If true
, the url will
be normalized. If an object, it will be the
options object sent to normalize-url
.
For SSH urls, normalize won't work.
protocols
(Array): An array with the url protocols (usually it has one element).protocol
(String): The first protocol, "ssh"
(if the url is a ssh url) or "file"
.port
(null|Number): The domain port.resource
(String): The url domain (including subdomains).user
(String): The authentication user (usually for ssh urls).pathname
(String): The url pathname.hash
(String): The url hash.search
(String): The url querystring value.href
(String): The input url.query
(Object): The url querystring, parsed as object.Have an idea? Found a bug? See how to contribute.
I open-source almost everything I can, and I try to reply to everyone needing help using these projects. Obviously, this takes time. You can integrate and use these projects in your applications for free! You can even change the source code and redistribute (even resell it).
However, if you get some profit from this or just want to encourage me to continue creating stuff, there are few ways you can do it:
Starring and sharing the projects you like :rocket:
—I love books! I will remember you after years if you buy me one. :grin: :book:
—You can make one-time donations via PayPal. I'll probably buy a
coffee tea. :tea:
—Set up a recurring monthly donation and you will get interesting news about what I'm doing (things that I don't share with everyone).
Bitcoin—You can send me bitcoins at this address (or scanning the code below): 1P9BRsmazNQcuyTxEqveUsnf5CERdq35V6
Thanks! :heart:
If you are using this library in one of your projects, add it in this list. :sparkles:
git-up
lien
stun
@open-wa/wa-automate
kakapo
parse-db-uri
url-local
fuge-runner
build-plugin-ssr
rucksack
egg-muc-custom-loader
hologit
@enkeledi/react-native-week-month-date-picker
normalize-ssh
robots-agent
warp-api
normalize-id
xl-git-up
warp-server
@hemith/react-native-tnk
@kriblet/wa-automate
@notnuzzel/crawl
gitlab-backup-util-harduino
bilibili2local
miguelcostero-ng2-toasty
native-kakao-login
react-native-my-first-try-arun-ramya
react-native-kakao-maps
react-native-is7
react-native-ytximkit
react-native-payu-payment-testing
npm_one_1_2_3
react-native-biometric-authenticate
react-native-arunmeena1987
react-native-contact-list
rn-adyen-dropin
@positionex/position-sdk
begg
@corelmax/react-native-my2c2p-sdk
@dataparty/api
@felipesimmi/react-native-datalogic-module
@jprustv/sulla-hotfix
@hawkingnetwork/react-native-tab-view
@mergulhao/wa-automate
cli-live-tutorial
drowl-base-theme-iconset
native-apple-login
react-native-cplus
npm_qwerty
ssh-host-manager
soajs.repositories
react-native-arunjeyam1987
react-native-bubble-chart
verify-aws-sns-signature
vrt-cli
vue-cli-plugin-ice-builder
react-native-flyy
graphmilker
native-zip
@apardellass/react-native-audio-stream
@geeky-apo/react-native-advanced-clipboard
@hsui/plugin-wss
blitzzz
candlelabssdk
@roshub/api
@saad27/react-native-bottom-tab-tour
generator-bootstrap-boilerplate-template
react-feedback-sdk
loast
npm_one_12_34_1_
npm_one_2_2
payutesting
react-native-sayhello-module
react-native-dsphoto-module
vue-cli-plugin-ut-builder
xbuilder-forms
tumblr-text
deploy-versioning
eval-spider
homebridge-pushcutter
@con-test/react-native-concent-common
@hstech/utils
@angga30prabu/wa-modified
birken-react-native-community-image-editor
get-tarball-cli
luojia-cli-dev
reac-native-arun-ramya-test
react-native-transtracker-library
react-native-pulsator-native
react-native-arun-ramya-test
react-native-arunramya151
react-native-plugpag-wrapper
workpad
ndla-source-map-resolver
@screeb/react-native
@lakutata-module/service
delta-screen
microbe.js
@buganto/client
@jimengio/mocked-proxy
@mockswitch/cli
@ndla/source-map-resolver
angularvezba
api-reach-react-native-fix
astra-ufo-sdk
react-native-syan-photo-picker
@wecraftapps/react-native-use-keyboard
hui-plugin-wss
l2forlerna
native-google-login
raact-native-arunramya151
react-native-modal-progress-bar
react-native-test-module-hhh
react-native-jsi-device-info
react-native-badge-control
rn-tm-notify
wander-cli
ts-scraper
heroku-wp-environment-sync
hubot-will-it-connect
normalize-ssh-url
ba-js-cookie-banner
FAQs
An advanced url parser supporting git urls too.
The npm package parse-url receives a total of 1,637,638 weekly downloads. As such, parse-url popularity was classified as popular.
We found that parse-url demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.