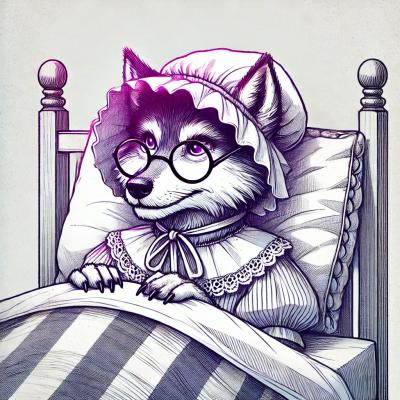
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
passport-oauth2
Advanced tools
The `passport-oauth2` npm package is a strategy for the Passport authentication middleware that allows you to authenticate using OAuth 2.0. It is designed to be flexible and can be used with any OAuth 2.0 provider, making it a versatile tool for integrating third-party authentication into your Node.js applications.
OAuth 2.0 Authentication
This feature allows you to set up OAuth 2.0 authentication with a specified provider. The code sample demonstrates how to configure the OAuth2Strategy with the necessary endpoints and client credentials, and how to handle the authentication callback.
const passport = require('passport');
const OAuth2Strategy = require('passport-oauth2').Strategy;
passport.use(new OAuth2Strategy({
authorizationURL: 'https://example.com/oauth2/authorize',
tokenURL: 'https://example.com/oauth2/token',
clientID: 'YOUR_CLIENT_ID',
clientSecret: 'YOUR_CLIENT_SECRET',
callbackURL: 'https://www.example.net/auth/example/callback'
},
function(accessToken, refreshToken, profile, cb) {
User.findOrCreate({ exampleId: profile.id }, function (err, user) {
return cb(err, user);
});
}
));
Custom Authorization Parameters
This feature allows you to add custom headers or parameters to the authorization request. The code sample shows how to include custom headers in the OAuth2Strategy configuration.
passport.use(new OAuth2Strategy({
authorizationURL: 'https://example.com/oauth2/authorize',
tokenURL: 'https://example.com/oauth2/token',
clientID: 'YOUR_CLIENT_ID',
clientSecret: 'YOUR_CLIENT_SECRET',
callbackURL: 'https://www.example.net/auth/example/callback',
customHeaders: { 'Authorization': 'Bearer YOUR_ACCESS_TOKEN' }
},
function(accessToken, refreshToken, profile, cb) {
User.findOrCreate({ exampleId: profile.id }, function (err, user) {
return cb(err, user);
});
}
));
Token Revocation
This feature allows you to revoke an OAuth 2.0 token. The code sample demonstrates how to send a POST request to the token revocation endpoint with the token to be revoked.
const request = require('request');
function revokeToken(token, done) {
const options = {
url: 'https://example.com/oauth2/revoke',
form: { token: token }
};
request.post(options, function(err, response, body) {
if (err) { return done(err); }
done(null, body);
});
}
The `passport-google-oauth20` package is a Passport strategy for authenticating with Google using the OAuth 2.0 API. It is specifically tailored for Google authentication, making it easier to integrate Google login into your application. Unlike `passport-oauth2`, which is a general-purpose OAuth 2.0 strategy, `passport-google-oauth20` is specialized for Google.
The `passport-facebook` package is a Passport strategy for authenticating with Facebook using the OAuth 2.0 API. It simplifies the process of integrating Facebook login into your application. Similar to `passport-google-oauth20`, it is specialized for Facebook authentication, whereas `passport-oauth2` is more generic.
The `passport-github` package is a Passport strategy for authenticating with GitHub using the OAuth 2.0 API. It is designed specifically for GitHub authentication, making it easier to integrate GitHub login into your application. This package is specialized for GitHub, unlike `passport-oauth2`, which can be used with any OAuth 2.0 provider.
General-purpose OAuth 2.0 authentication strategy for Passport.
This module lets you authenticate using OAuth 2.0 in your Node.js applications. By plugging into Passport, OAuth 2.0 authentication can be easily and unobtrusively integrated into any application or framework that supports Connect-style middleware, including Express.
Note that this strategy provides generic OAuth 2.0 support. In many cases, a provider-specific strategy can be used instead, which cuts down on unnecessary configuration, and accommodates any provider-specific quirks. See the list for supported providers.
Developers who need to implement authentication against an OAuth 2.0 provider that is not already supported are encouraged to sub-class this strategy. If you choose to open source the new provider-specific strategy, please add it to the list so other people can find it.
$ npm install passport-oauth2
The OAuth 2.0 authentication strategy authenticates users using a third-party
account and OAuth 2.0 tokens. The provider's OAuth 2.0 endpoints, as well as
the client identifer and secret, are specified as options. The strategy
requires a verify
callback, which receives an access token and profile,
and calls done
providing a user.
passport.use(new OAuth2Strategy({
authorizationURL: 'https://www.example.com/oauth2/authorize',
tokenURL: 'https://www.example.com/oauth2/token',
clientID: EXAMPLE_CLIENT_ID,
clientSecret: EXAMPLE_CLIENT_SECRET,
callbackURL: "http://localhost:3000/auth/example/callback"
},
function(accessToken, refreshToken, profile, done) {
User.findOrCreate({ exampleId: profile.id }, function (err, user) {
return done(err, user);
});
}
));
Use passport.authenticate()
, specifying the 'oauth2'
strategy, to
authenticate requests.
For example, as route middleware in an Express application:
app.get('/auth/example',
passport.authenticate('oauth'));
app.get('/auth/example/callback',
passport.authenticate('oauth', { failureRedirect: '/login' }),
function(req, res) {
// Successful authentication, redirect home.
res.redirect('/');
});
$ npm install
$ npm test
Copyright (c) 2011-2013 Jared Hanson <http://jaredhanson.net/>
FAQs
OAuth 2.0 authentication strategy for Passport.
The npm package passport-oauth2 receives a total of 310,229 weekly downloads. As such, passport-oauth2 popularity was classified as popular.
We found that passport-oauth2 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.