Comparing version 0.6.0 to 0.7.0
@@ -9,3 +9,21 @@ # Changelog | ||
## [0.7.0] - 2023-11-27 | ||
### Changed | ||
- Set `req.authInfo` by default when using the `assignProperty` option to | ||
`authenticate()` middleware. This makes the behavior the same as when not using | ||
the option, and can be disabled by setting `authInfo` option to `false`. | ||
## [0.6.0] - 2022-05-20 | ||
### Added | ||
- `authenticate()`, `req#login`, and `req#logout` accept a | ||
`keepSessionInfo: true` option to keep session information after regenerating | ||
the session. | ||
### Changed | ||
- `req#login()` and `req#logout()` regenerate the the session and clear session | ||
information by default. | ||
- `req#logout()` is now an asynchronous function and requires a callback | ||
function as the last argument. | ||
### Security | ||
@@ -12,0 +30,0 @@ |
@@ -1,4 +0,2 @@ | ||
/** | ||
* Module dependencies. | ||
*/ | ||
// Module dependencies. | ||
var SessionStrategy = require('./strategies/session') | ||
@@ -9,5 +7,6 @@ , SessionManager = require('./sessionmanager'); | ||
/** | ||
* `Authenticator` constructor. | ||
* Create a new `Authenticator` object. | ||
* | ||
* @api public | ||
* @public | ||
* @class | ||
*/ | ||
@@ -28,3 +27,8 @@ function Authenticator() { | ||
* | ||
* @api protected | ||
* Initializes the `Authenticator` instance by creating the default `{@link SessionManager}`, | ||
* {@link Authenticator#use `use()`}'ing the default `{@link SessionStrategy}`, and | ||
* adapting it to work as {@link https://github.com/senchalabs/connect#readme Connect}-style | ||
* middleware, which is also compatible with {@link https://expressjs.com/ Express}. | ||
* | ||
* @private | ||
*/ | ||
@@ -38,15 +42,18 @@ Authenticator.prototype.init = function() { | ||
/** | ||
* Utilize the given `strategy` with optional `name`, overridding the strategy's | ||
* default name. | ||
* Register a strategy for later use when authenticating requests. The name | ||
* with which the strategy is registered is passed to {@link Authenticator#authenticate `authenticate()`}. | ||
* | ||
* Examples: | ||
* @public | ||
* @param {string} [name=strategy.name] - Name of the strategy. When specified, | ||
* this value overrides the strategy's name. | ||
* @param {Strategy} strategy - Authentication strategy. | ||
* @returns {this} | ||
* | ||
* passport.use(new TwitterStrategy(...)); | ||
* @example <caption>Register strategy.</caption> | ||
* passport.use(new GoogleStrategy(...)); | ||
* | ||
* passport.use('api', new http.BasicStrategy(...)); | ||
* | ||
* @param {String|Strategy} name | ||
* @param {Strategy} strategy | ||
* @return {Authenticator} for chaining | ||
* @api public | ||
* @example <caption>Register strategy and override name.</caption> | ||
* passport.use('password', new LocalStrategy(function(username, password, cb) { | ||
* // ... | ||
* })); | ||
*/ | ||
@@ -65,19 +72,14 @@ Authenticator.prototype.use = function(name, strategy) { | ||
/** | ||
* Un-utilize the `strategy` with given `name`. | ||
* Deregister a strategy that was previously registered with the given name. | ||
* | ||
* In typical applications, the necessary authentication strategies are static, | ||
* configured once and always available. As such, there is often no need to | ||
* invoke this function. | ||
* In a typical application, the necessary authentication strategies are | ||
* registered when initializing the app and, once registered, are always | ||
* available. As such, it is typically not necessary to call this function. | ||
* | ||
* However, in certain situations, applications may need dynamically configure | ||
* and de-configure authentication strategies. The `use()`/`unuse()` | ||
* combination satisfies these scenarios. | ||
* @public | ||
* @param {string} name - Name of the strategy. | ||
* @returns {this} | ||
* | ||
* Examples: | ||
* | ||
* passport.unuse('legacy-api'); | ||
* | ||
* @param {String} name | ||
* @return {Authenticator} for chaining | ||
* @api public | ||
* @example | ||
* passport.unuse('acme'); | ||
*/ | ||
@@ -90,19 +92,11 @@ Authenticator.prototype.unuse = function(name) { | ||
/** | ||
* Setup Passport to be used under framework. | ||
* Adapt this `Authenticator` to work with a specific framework. | ||
* | ||
* By default, Passport exposes middleware that operate using Connect-style | ||
* middleware using a `fn(req, res, next)` signature. Other popular frameworks | ||
* have different expectations, and this function allows Passport to be adapted | ||
* to operate within such environments. | ||
* By default, Passport works as {@link https://github.com/senchalabs/connect#readme Connect}-style | ||
* middleware, which makes it compatible with {@link https://expressjs.com/ Express}. | ||
* For any app built using Express, there is no need to call this function. | ||
* | ||
* If you are using a Connect-compatible framework, including Express, there is | ||
* no need to invoke this function. | ||
* | ||
* Examples: | ||
* | ||
* passport.framework(require('hapi-passport')()); | ||
* | ||
* @param {Object} name | ||
* @return {Authenticator} for chaining | ||
* @api public | ||
* @public | ||
* @param {Object} fw | ||
* @returns {this} | ||
*/ | ||
@@ -115,19 +109,26 @@ Authenticator.prototype.framework = function(fw) { | ||
/** | ||
* Passport's primary initialization middleware. | ||
* Create initialization middleware. | ||
* | ||
* This middleware must be in use by the Connect/Express application for | ||
* Passport to operate. | ||
* Returns middleware that initializes Passport to authenticate requests. | ||
* | ||
* Options: | ||
* - `userProperty` Property to set on `req` upon login, defaults to _user_ | ||
* As of v0.6.x, it is typically no longer necessary to use this middleware. It | ||
* exists for compatiblity with apps built using previous versions of Passport, | ||
* in which this middleware was necessary. | ||
* | ||
* Examples: | ||
* The primary exception to the above guidance is when using strategies that | ||
* depend directly on `passport@0.4.x` or earlier. These earlier versions of | ||
* Passport monkeypatch Node.js `http.IncomingMessage` in a way that expects | ||
* certain Passport-specific properties to be available. This middleware | ||
* provides a compatibility layer for this situation. | ||
* | ||
* app.use(passport.initialize()); | ||
* @public | ||
* @param {Object} [options] | ||
* @param {string} [options.userProperty='user'] - Determines what property on | ||
* `req` will be set to the authenticated user object. | ||
* @param {boolean} [options.compat=true] - When `true`, enables a compatibility | ||
* layer for packages that depend on `passport@0.4.x` or earlier. | ||
* @returns {function} | ||
* | ||
* app.use(passport.initialize({ userProperty: 'currentUser' })); | ||
* | ||
* @param {Object} options | ||
* @return {Function} middleware | ||
* @api public | ||
* @example | ||
* app.use(passport.initialize()); | ||
*/ | ||
@@ -140,9 +141,9 @@ Authenticator.prototype.initialize = function(options) { | ||
/** | ||
* Middleware that will authenticate a request using the given `strategy` name, | ||
* with optional `options` and `callback`. | ||
* Create authentication middleware. | ||
* | ||
* Returns middleware that authenticates the request by applying the given | ||
* strategy (or strategies). | ||
* | ||
* Examples: | ||
* | ||
* passport.authenticate('local', { successRedirect: '/', failureRedirect: '/login' })(req, res); | ||
* | ||
* passport.authenticate('local', function(err, user) { | ||
@@ -153,16 +154,25 @@ * if (!user) { return res.redirect('/login'); } | ||
* | ||
* passport.authenticate('basic', { session: false })(req, res); | ||
* @public | ||
* @param {string|string[]|Strategy} strategy | ||
* @param {Object} [options] | ||
* @param {boolean} [options.session=true] | ||
* @param {boolean} [options.keepSessionInfo=false] | ||
* @param {string} [options.failureRedirect] | ||
* @param {boolean|string|Object} [options.failureFlash=false] | ||
* @param {boolean|string} [options.failureMessage=false] | ||
* @param {boolean|string|Object} [options.successFlash=false] | ||
* @param {string} [options.successReturnToOrRedirect] | ||
* @param {string} [options.successRedirect] | ||
* @param {boolean|string} [options.successMessage=false] | ||
* @param {boolean} [options.failWithError=false] | ||
* @param {string} [options.assignProperty] | ||
* @param {boolean} [options.authInfo=true] | ||
* @param {function} [callback] | ||
* @returns {function} | ||
* | ||
* app.get('/auth/twitter', passport.authenticate('twitter'), function(req, res) { | ||
* // request will be redirected to Twitter | ||
* }); | ||
* app.get('/auth/twitter/callback', passport.authenticate('twitter'), function(req, res) { | ||
* res.json(req.user); | ||
* }); | ||
* @example <caption>Authenticate username and password submitted via HTML form.</caption> | ||
* app.get('/login/password', passport.authenticate('local', { successRedirect: '/', failureRedirect: '/login' })); | ||
* | ||
* @param {String} strategy | ||
* @param {Object} options | ||
* @param {Function} callback | ||
* @return {Function} middleware | ||
* @api public | ||
* @example <caption>Authenticate bearer token used to access an API resource.</caption> | ||
* app.get('/api/resource', passport.authenticate('bearer', { session: false })); | ||
*/ | ||
@@ -174,20 +184,28 @@ Authenticator.prototype.authenticate = function(strategy, options, callback) { | ||
/** | ||
* Middleware that will authorize a third-party account using the given | ||
* `strategy` name, with optional `options`. | ||
* Create third-party service authorization middleware. | ||
* | ||
* If authorization is successful, the result provided by the strategy's verify | ||
* callback will be assigned to `req.account`. The existing login session and | ||
* `req.user` will be unaffected. | ||
* Returns middleware that will authorize a connection to a third-party service. | ||
* | ||
* This function is particularly useful when connecting third-party accounts | ||
* to the local account of a user that is currently authenticated. | ||
* This middleware is identical to using {@link Authenticator#authenticate `authenticate()`} | ||
* middleware with the `assignProperty` option set to `'account'`. This is | ||
* useful when a user is already authenticated (for example, using a username | ||
* and password) and they want to connect their account with a third-party | ||
* service. | ||
* | ||
* Examples: | ||
* In this scenario, the user's third-party account will be set at | ||
* `req.account`, and the existing `req.user` and login session data will be | ||
* be left unmodified. A route handler can then link the third-party account to | ||
* the existing local account. | ||
* | ||
* passport.authorize('twitter-authz', { failureRedirect: '/account' }); | ||
* All arguments to this function behave identically to those accepted by | ||
* `{@link Authenticator#authenticate}`. | ||
* | ||
* @param {String} strategy | ||
* @param {Object} options | ||
* @return {Function} middleware | ||
* @api public | ||
* @public | ||
* @param {string|string[]|Strategy} strategy | ||
* @param {Object} [options] | ||
* @param {function} [callback] | ||
* @returns {function} | ||
* | ||
* @example | ||
* app.get('/oauth/callback/twitter', passport.authorize('twitter')); | ||
*/ | ||
@@ -194,0 +212,0 @@ Authenticator.prototype.authorize = function(strategy, options, callback) { |
@@ -1,4 +0,2 @@ | ||
/** | ||
* Module dependencies. | ||
*/ | ||
// Module dependencies. | ||
var Passport = require('./authenticator') | ||
@@ -22,3 +20,3 @@ , SessionStrategy = require('./strategies/session'); | ||
/** | ||
/* | ||
* Expose strategies. | ||
@@ -25,0 +23,0 @@ */ |
@@ -253,3 +253,12 @@ /** | ||
req[options.assignProperty] = user; | ||
return next(); | ||
if (options.authInfo !== false) { | ||
passport.transformAuthInfo(info, req, function(err, tinfo) { | ||
if (err) { return next(err); } | ||
req.authInfo = tinfo; | ||
next(); | ||
}); | ||
} else { | ||
next(); | ||
} | ||
return; | ||
} | ||
@@ -256,0 +265,0 @@ |
@@ -1,4 +0,2 @@ | ||
/** | ||
* Module dependencies. | ||
*/ | ||
// Module dependencies. | ||
var pause = require('pause') | ||
@@ -10,5 +8,35 @@ , util = require('util') | ||
/** | ||
* `SessionStrategy` constructor. | ||
* Create a new `SessionStrategy` object. | ||
* | ||
* @api public | ||
* An instance of this strategy is automatically used when creating an | ||
* `{@link Authenticator}`. As such, it is typically unnecessary to create an | ||
* instance using this constructor. | ||
* | ||
* @classdesc This `Strategy` authenticates HTTP requests based on the contents | ||
* of session data. | ||
* | ||
* The login session must have been previously initiated, typically upon the | ||
* user interactively logging in using a HTML form. During session initiation, | ||
* the logged-in user's information is persisted to the session so that it can | ||
* be restored on subsequent requests. | ||
* | ||
* Note that this strategy merely restores the authentication state from the | ||
* session, it does not authenticate the session itself. Authenticating the | ||
* underlying session is assumed to have been done by the middleware | ||
* implementing session support. This is typically accomplished by setting a | ||
* signed cookie, and verifying the signature of that cookie on incoming | ||
* requests. | ||
* | ||
* In {@link https://expressjs.com/ Express}-based apps, session support is | ||
* commonly provided by {@link https://github.com/expressjs/session `express-session`} | ||
* or {@link https://github.com/expressjs/cookie-session `cookie-session`}. | ||
* | ||
* @public | ||
* @class | ||
* @augments base.Strategy | ||
* @param {Object} [options] | ||
* @param {string} [options.key='passport'] - Determines what property ("key") on | ||
* the session data where login session data is located. The login | ||
* session is stored and read from `req.session[key]`. | ||
* @param {function} deserializeUser - Function which deserializes user. | ||
*/ | ||
@@ -23,2 +51,8 @@ function SessionStrategy(options, deserializeUser) { | ||
Strategy.call(this); | ||
/** The name of the strategy, set to `'session'`. | ||
* | ||
* @type {string} | ||
* @readonly | ||
*/ | ||
this.name = 'session'; | ||
@@ -29,19 +63,36 @@ this._key = options.key || 'passport'; | ||
/** | ||
* Inherit from `Strategy`. | ||
*/ | ||
// Inherit from `passport.Strategy`. | ||
util.inherits(SessionStrategy, Strategy); | ||
/** | ||
* Authenticate request based on the current session state. | ||
* Authenticate request based on current session data. | ||
* | ||
* The session authentication strategy uses the session to restore any login | ||
* state across requests. If a login session has been established, `req.user` | ||
* will be populated with the current user. | ||
* When login session data is present in the session, that data will be used to | ||
* restore login state across across requests by calling the deserialize user | ||
* function. | ||
* | ||
* This strategy is registered automatically by Passport. | ||
* If login session data is not present, the request will be passed to the next | ||
* middleware, rather than failing authentication - which is the behavior of | ||
* most other strategies. This deviation allows session authentication to be | ||
* performed at the application-level, rather than the individual route level, | ||
* while allowing both authenticated and unauthenticated requests and rendering | ||
* responses accordingly. Routes that require authentication will need to guard | ||
* that condition. | ||
* | ||
* @param {Object} req | ||
* @param {Object} options | ||
* @api protected | ||
* This function is protected, and should not be called directly. Instead, | ||
* use `passport.authenticate()` middleware and specify the {@link SessionStrategy#name `name`} | ||
* of this strategy and any options. | ||
* | ||
* @protected | ||
* @param {http.IncomingMessage} req - The Node.js {@link https://nodejs.org/api/http.html#class-httpincomingmessage `IncomingMessage`} | ||
* object. | ||
* @param {Object} [options] | ||
* @param {boolean} [options.pauseStream=false] - When `true`, data events on | ||
* the request will be paused, and then resumed after the asynchronous | ||
* `deserializeUser` function has completed. This is only necessary in | ||
* cases where later middleware in the stack are listening for events, | ||
* and ensures that those events are not missed. | ||
* | ||
* @example | ||
* passport.authenticate('session'); | ||
*/ | ||
@@ -82,6 +133,3 @@ SessionStrategy.prototype.authenticate = function(req, options) { | ||
/** | ||
* Expose `SessionStrategy`. | ||
*/ | ||
// Export `SessionStrategy`. | ||
module.exports = SessionStrategy; |
{ | ||
"name": "passport", | ||
"version": "0.6.0", | ||
"version": "0.7.0", | ||
"description": "Simple, unobtrusive authentication for Node.js.", | ||
@@ -5,0 +5,0 @@ "keywords": [ |
@@ -23,3 +23,15 @@ [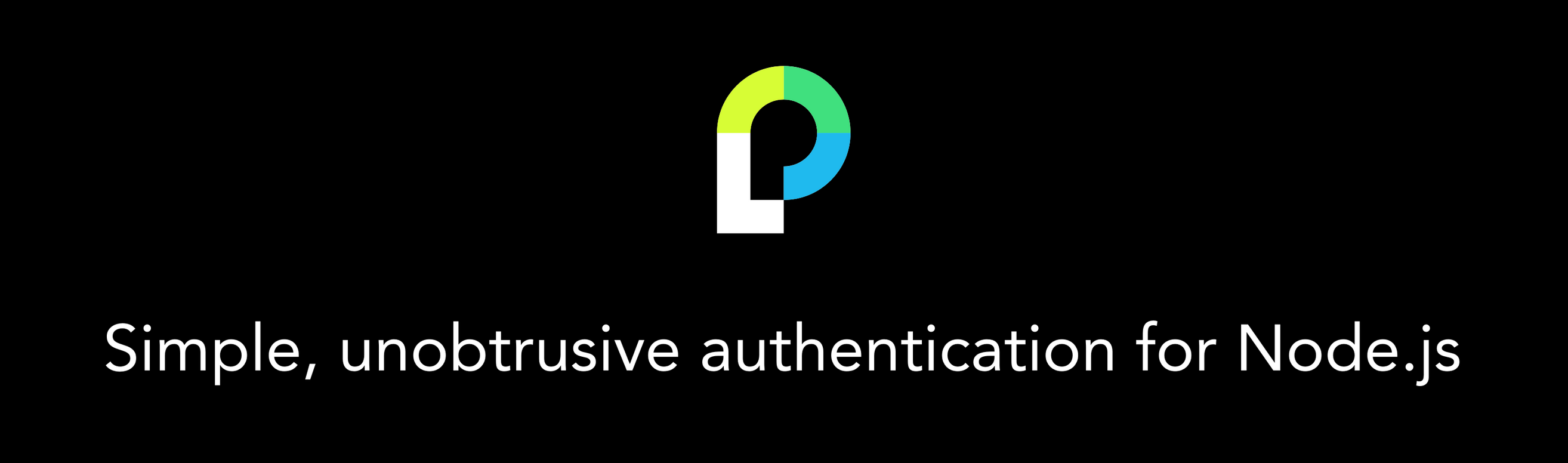](http://passportjs.org) | ||
<br> | ||
<a href="https://snyk.co/passport"><img src="https://raw.githubusercontent.com/jaredhanson/passport/master/sponsors/snyk.png" width="301" height="171"></a><br/> | ||
<br> | ||
<a href="https://www.descope.com/?utm_source=PassportJS&utm_medium=referral&utm_campaign=oss-sponsorship"> | ||
<picture> | ||
<source media="(prefers-color-scheme: dark)" srcset="https://raw.githubusercontent.com/jaredhanson/passport/master/sponsors/descope-dark.svg"> | ||
<source media="(prefers-color-scheme: light)" srcset="https://raw.githubusercontent.com/jaredhanson/passport/master/sponsors/descope.svg"> | ||
<img src="https://raw.githubusercontent.com/jaredhanson/passport/master/sponsors/descope.svg" width="275"> | ||
</picture> | ||
</a><br/> | ||
<a href="https://www.descope.com/?utm_source=PassportJS&utm_medium=referral&utm_campaign=oss-sponsorship"><b>Drag and drop your auth</b><br/>Add authentication and user management to your consumer and business apps with a few lines of code.</a> | ||
<br> | ||
<br> | ||
<a href="https://fusionauth.io/?utm_source=passportjs&utm_medium=referral&utm_campaign=sponsorship"><img src="https://raw.githubusercontent.com/jaredhanson/passport/master/sponsors/fusionauth.png" width="275"></a><br/> | ||
<a href="https://fusionauth.io/?utm_source=passportjs&utm_medium=referral&utm_campaign=sponsorship"><b>Auth. Built for Devs, by Devs</b><br/>Add login, registration, SSO, MFA, and a bazillion other features to your app in minutes. Integrates with any codebase and installs on any server, anywhere in the world.</a> | ||
</p> | ||
@@ -26,0 +38,0 @@ |
156687
21
1226
186