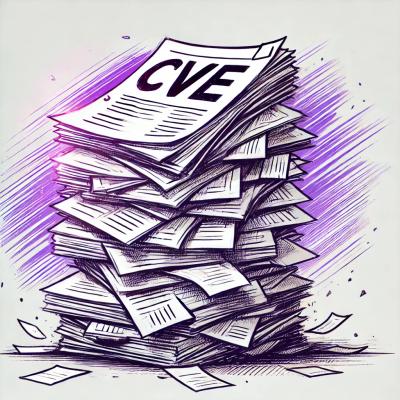
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
payment-backoffice-api
Advanced tools
This project acts like an authentication proxy. Each route will call the desired API.
For payload and params validation, please refer to specific API documentation.
v0.5.0
Developers will probably need to run docker
The project needs some environment variables to run properly.
In development mode, you can use a .env
file to define them.
ACCOUNTING_API_URL
: accounting api urlACCOUNTING_API_SHARED_KEY
: accounting api shared key without ending slashAUTH_HEADER_KEY
=oyst-authorizationAUTH_HEADER_PREFIX
=OystBO_PAY_FRONT_URL
: url of payment-backoffice-frontDATABASE_URL
: Databse connection stringMAIL_FROM_ADDR
="no-reply@oyst.com"MAIL_FROM_ALIAS
="OYST Validator"MAIL_SUPPORT_TO
: email address to which the support message will be sentMAIL_VALIDATION_TO
: email address to which the validation email will be sentMERCHANT_API_SHARED_KEY
: Shared key for merchant-apiMERCHANT_API_URL
: Merchant's API endpointPAYMENT_API_URL
: Payment's API endpointPAYMENT_API_SHARED_KEY
: Shared key for payment-apiSENDGRID_API_KEY
: API key for sendgridSHARED_KEY
: Shared encryption key used to sign and verify JsonWebTokenSWAGGER_HOST
(optional): define the URL used by Swagger to test APIs. eg: localhost:8080
USER_API_URL
: User API endpoint$ npm install
In development mode, be sure docker is running postgres
:
$ docker-compose up -d
Then, in development mode:
$ $(npm bin)/gulp serve
Or, in production mode:
$ $(npm bin)/npm run start
If a route needs authentication, you have to provide an auth header
AUTH_HEADER_KEY: AUTH_HEADER_PREFIX jwt_token
POST /users
needs auth: FALSE
payload
Joi.object({
email: Joi.string().email().required(),
password: Joi.string().min(8).max(20).required(),
password_confirmation: Joi.any()
.valid(Joi.ref('password'))
.required().options({language: {any: {allowOnly: 'must match password'}}})
.strip(),
phone: phoneValidator.phone().mobile().required()
})
{
"token": "JWT token used for authentication",
"user": {
"created_at": "",
"email": "",
"id": "",
"merchants": ["merchantID"],
"phone": "",
"scopes": ["USER"],
"updated_at": ""
}
}
POST /sessions
needs auth: FALSE
payload
Joi.object({
email: Joi.string().email().required(),
password: Joi.string().required()
})
{
"token": "JWT token used for authentication",
"merchant": {},
"user": {
"created_at": "",
"email": "",
"id": "",
"merchants": ["merchantID"],
"phone": "",
"scopes": ["USER"],
"updated_at": ""
}
}
GET /sessions/{token}
needs auth: TRUE
return
404
Bad token401
Not authenticated200
authenticated
{
"token": "JWT token used for authentication",
"user": {
"created_at": "",
"email": "",
"id": "",
"merchants": ["merchantID"],
"phone": "",
"scopes": ["USER"],
"updated_at": ""
}
}
PUT /users/{id}
TRUE
Joi.object().keys({
email: Joi.string().email(),
password: Joi.string().min(8),
password_confirmation: Joi.any()
.valid(Joi.ref('password'))
.required().options({language: {any: {allowOnly: 'must match password'}}})
.strip().optional(),
phone: phoneValidator.phone().mobile()
}).or(
'email',
'password',
'password_confirmation',
'phone'
)
{
"statusCode": 200,
"success": true,
"user": {
"created_at": "",
"email": "",
"id": "",
"merchants": ["merchantID"],
"phone": "",
"scopes": ["USER"],
"updated_at": ""
}
}
POST /support/mail
FALSE
Joi.object({
email: Joi.string().email().required(),
message: Joi.string().required(),
subject: Joi.string().required()
})
{
"statusCode": 200,
"success": true
}
PATCH /users/password
TRUE
Joi.object({
current: Joi.string().min(8).max(20).required(),
password: Joi.string().min(8).max(20).required(),
password_confirmation: Joi.any()
.valid(Joi.ref('password'))
.required().options({language: {any: {allowOnly: 'must match password'}}})
.strip()
})
{
"statusCode": 200,
"success": true
}
POST /users/password/forgot
FALSE
Joi.object({
email: Joi.string().email().required()
})
{
"statusCode": 200,
"success": true
}
GET /users/password/checkToken
FALSE
Joi.object({
id: Joi.string().guid().required(),
token: jwt.required()
})
{
"statusCode": 200,
"success": true
}
PATCH /users/password/new
FALSE
Joi.object({
password: Joi.string().min(8).max(20).required(),
password_confirmation: Joi.any()
.valid(Joi.ref('password'))
.required().options({language: {any: {allowOnly: 'must match password'}}})
.strip()
})
Joi.object({
id: Joi.string().guid().required(),
token: jwt.required()
})
{
"token": "JWT token used for authentication",
"user": {
"created_at": "",
"email": "",
"id": "",
"merchants": ["merchantID"],
"phone": "",
"scopes": ["USER"],
"updated_at": ""
}
}
GET /merchants/{id}/activate/{token}
Activate a merchant using link provided by email (OYST side)
needs auth: FALSE
params
{
id: Joi.string().guid().required(),
token: jwt.required()
}
PATCH /merchants/{id}/activate
GET /merchants/{id}/deactivate/{token}
Deactivate a merchant using link provided by email (OYST side)
needs auth: FALSE
params
{
id: Joi.string().guid().required(),
token: jwt.required()
}
PATCH /merchants/{id}/deactivate
POST /merchants
Create a merchant
TRUE
GET /merchants
Get merchant's informations based on logged in user's merchantID
needs auth: TRUE
remote endpoint: GET /merchants/{id}
PUT /merchants
Update merchant's informations based on logged in user's merchantID
needs auth: TRUE
remote endpoint: PUT /merchants/{id}
PUT /merchants/upload/{type}
Upload merchant's CGV/logo based on logged in user's merchantID
needs auth: TRUE
params
{
type: Joi.string().valid([
'cgv',
'logo'
])
}
PUT /merchants/{id}/upload/{type}
GET /payments
Get all transactions with pagination based on logged in user's merchantID
needs auth: TRUE
query params
{
page: Joi.number().integer().min(1).default(1),
per_page: Joi.number().integer().max(100).default(10)
}
GET /merchants/{merchant_id}/payments
POST /payments/{id}/cancel
Cancel desired transaction based on logged in user's merchantID
needs auth: TRUE
remote endpoint: POST /merchants/{merchant_id}/payments/{id}/cancel
POST /payments/{id}/refund
Refund desired transaction based on logged in user's merchantID
needs auth: TRUE
remote endpoint: POST /merchants/{merchant_id}/payments/{id}/refund
GET /payments/{id}
Get desired transaction based on logged in user's merchantID
needs auth: TRUE
remote endpoint: */!\ Not yet implemented /!*
GET /payments/overview
Get overview for transactions based on logged in user's merchantID
needs auth: TRUE
remote endpoint: /merchants/{merchant_id}/payments/overview
GET /accounting/overview
Get merchant's account's overview based on logged in user's merchantID
needs auth: TRUE
remote endpoint: /merchants/${merchant_id}/payments/overview
POST /payouts
Create new payout
needs auth: TRUE
remote endpoint: POST /merchants/{merchant_id}/payouts/submit
GET /payouts
Get all payouts from current logged in merchant
needs auth: TRUE
remote endpoint: GET /merchants/{merchant_id}/payouts
v0.8.0
v0.7.0
Validated
or Refused
v0.6.0
v0.5.0
v0.4.1
v0.4.0
FAQs
BO Oyst PAY
The npm package payment-backoffice-api receives a total of 2 weekly downloads. As such, payment-backoffice-api popularity was classified as not popular.
We found that payment-backoffice-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.