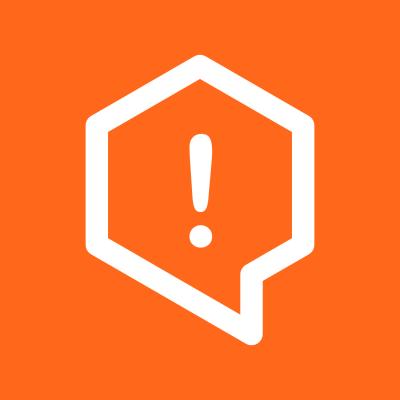
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
paypal-invoices
Advanced tools
An api wrapper for paypal 2.0 invoices.
npm i paypal-invoices
const { Invoices } = require('paypal-invoices')
const invoice = {...}
const main = async () => {
// Create a new API instance
const api = new Invoices(CLIENT_ID, CLIENT_SECRET)
// Or a sandbox api
// const api = new Invoices(CLIENT_ID, CLIENT_SECRET, true)
// Initialize the API
try {
await api.initialize();
} catch (e) {
console.log("Could not initialize");
return;
}
// Get the next Invoice number
const invoiceNum = await api.generateInvoiceNumber();
// Create a new Invoice draft
const link = await api.createDraftInvoice(/* Invoice Object*/);
// Get the created invoice
const invoiceDraft = await api.getInvoiceByLink(link);
// Send the new Invoice to the recipient
await api.sendInvoice(invoiceDraft.id);
};
main();
To enabled request logging, add the following environment variable.
PP_INVOICER:LOG=true
FAQs
Library for Paypal Invoice API
The npm package paypal-invoices receives a total of 17 weekly downloads. As such, paypal-invoices popularity was classified as not popular.
We found that paypal-invoices demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.