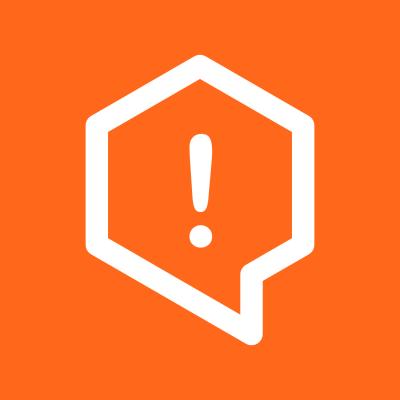
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
The pbf npm package is a low-level library for reading and writing Protocol Buffers (protobuf), a language-neutral, platform-neutral, extensible mechanism for serializing structured data. It is designed to be both efficient in performance and small in size, making it ideal for applications like map data processing in browsers or on servers.
Reading Protocol Buffers
This code sample demonstrates how to read data from a Protocol Buffers file. It uses the Pbf instance to parse the buffer based on predefined tags and assigns the parsed data to a JavaScript object.
const Pbf = require('pbf');
const { readFileSync } = require('fs');
const buffer = readFileSync('data.pbf');
const pbf = new Pbf(buffer);
const data = pbf.readFields(readData, {});
function readData(tag, data, pbf) {
if (tag === 1) data.name = pbf.readString();
else if (tag === 2) data.version = pbf.readVarint();
}
Writing Protocol Buffers
This code sample shows how to write data to a Protocol Buffers file. It creates a new Pbf instance, writes data into it by specifying tags, and then writes the serialized data to a file.
const Pbf = require('pbf');
const { writeFileSync } = require('fs');
const data = { name: 'Example', version: 1 };
const pbf = new Pbf();
writeData(data, pbf);
pbf.finish();
writeFileSync('output.pbf', Buffer.from(pbf.buffer));
function writeData(obj, pbf) {
pbf.writeStringField(1, obj.name);
pbf.writeVarintField(2, obj.version);
}
protobufjs is a more feature-rich library for working with Protocol Buffers in JavaScript. Unlike pbf, which focuses on minimalism and speed, protobufjs offers a comprehensive suite of tools including a CLI for generating static code and a more extensive API for manipulating protobuf schemas dynamically. This makes protobufjs better suited for applications requiring complex protobuf handling.
google-protobuf is the official Protocol Buffers library for JavaScript, developed by Google. It provides robust support for the full range of protobuf features, including reflection and extensions, which are not supported by pbf. This package is ideal for users looking for official support and the most complete implementation of the Protocol Buffers specification.
A low-level, fast, ultra-lightweight (3KB gzipped) JavaScript library for decoding and encoding protocol buffers, a compact binary format for structured data serialization. Works both in Node and the browser. Supports lazy decoding and detailed customization of the reading/writing code.
This library is extremely fast — much faster than native JSON.parse
/JSON.stringify
and the protocol-buffers module.
Here's a result from running a real-world benchmark on Node v6.5
(decoding and encoding a sample of 439 vector tiles, 22.6 MB total):
Install pbf
and compile a JavaScript module from a .proto
file:
$ npm install -g pbf
$ pbf example.proto > example.js
Then read and write objects using the module like this:
import Pbf from 'pbf';
import {readExample, writeExample} from './example.js';
// read
var obj = readExample(new Pbf(buffer));
// write
const pbf = new Pbf();
writeExample(obj, pbf);
const buffer = pbf.finish();
Alternatively, you can compile a protobuf schema file directly in the code:
import {compile} from 'pbf/compile';
import schema from 'protocol-buffers-schema';
const proto = schema.parse(fs.readFileSync('example.proto'));
const {readExample, writeExample} = compile(proto);
var data = new Pbf(buffer).readFields(readData, {});
function readData(tag, data, pbf) {
if (tag === 1) data.name = pbf.readString();
else if (tag === 2) data.version = pbf.readVarint();
else if (tag === 3) data.layer = pbf.readMessage(readLayer, {});
}
function readLayer(tag, layer, pbf) {
if (tag === 1) layer.name = pbf.readString();
else if (tag === 3) layer.size = pbf.readVarint();
}
var pbf = new Pbf();
writeData(data, pbf);
var buffer = pbf.finish();
function writeData(data, pbf) {
pbf.writeStringField(1, data.name);
pbf.writeVarintField(2, data.version);
pbf.writeMessage(3, writeLayer, data.layer);
}
function writeLayer(layer, pbf) {
pbf.writeStringField(1, layer.name);
pbf.writeVarintField(2, layer.size);
}
Install using NPM with npm install pbf
, then import as a module:
import Pbf from 'pbf';
Or use as a module directly in the browser with jsDelivr:
<script type="module">
import Pbf from 'https://cdn.jsdelivr.net/npm/pbf/+esm';
</script>
Alternatively, there's a browser bundle with a Pbf
global variable:
<script src="https://cdn.jsdelivr.net/npm/pbf"></script>
Create a Pbf
object, optionally given a Buffer
or Uint8Array
as input data:
// parse a pbf file from disk in Node
const pbf = new Pbf(fs.readFileSync('data.pbf'));
// parse a pbf file in a browser after an ajax request with responseType="arraybuffer"
const pbf = new Pbf(new Uint8Array(xhr.response));
Pbf
object properties:
pbf.length; // length of the underlying buffer
pbf.pos; // current offset for reading or writing
Read a sequence of fields:
pbf.readFields((tag) => {
if (tag === 1) pbf.readVarint();
else if (tag === 2) pbf.readString();
else ...
});
It optionally accepts an object that will be passed to the reading function for easier construction of decoded data,
and also passes the Pbf
object as a third argument:
const result = pbf.readFields(readField, {})
function readField(tag, result, pbf) {
if (tag === 1) result.id = pbf.readVarint();
}
To read an embedded message, use pbf.readMessage(fn[, obj])
(in the same way as read
).
Read values:
const value = pbf.readVarint();
const str = pbf.readString();
const numbers = pbf.readPackedVarint();
For lazy or partial decoding, simply save the position instead of reading a value, then later set it back to the saved value and read:
const fooPos = -1;
pbf.readFields((tag) => {
if (tag === 1) fooPos = pbf.pos;
});
...
pbf.pos = fooPos;
pbf.readMessage(readFoo);
Scalar reading methods:
readVarint(isSigned)
(pass true
if you expect negative varints)readSVarint()
readFixed32()
readFixed64()
readSFixed32()
readSFixed64()
readBoolean()
readFloat()
readDouble()
readString()
readBytes()
skip(value)
Packed reading methods:
readPackedVarint(arr, isSigned)
(appends read items to arr
)readPackedSVarint(arr)
readPackedFixed32(arr)
readPackedFixed64(arr)
readPackedSFixed32(arr)
readPackedSFixed64(arr)
readPackedBoolean(arr)
readPackedFloat(arr)
readPackedDouble(arr)
Write values:
pbf.writeVarint(123);
pbf.writeString("Hello world");
Write an embedded message:
pbf.writeMessage(1, writeObj, obj);
function writeObj(obj, pbf) {
pbf.writeStringField(obj.name);
pbf.writeVarintField(obj.version);
}
Field writing methods:
writeVarintField(tag, val)
writeSVarintField(tag, val)
writeFixed32Field(tag, val)
writeFixed64Field(tag, val)
writeSFixed32Field(tag, val)
writeSFixed64Field(tag, val)
writeBooleanField(tag, val)
writeFloatField(tag, val)
writeDoubleField(tag, val)
writeStringField(tag, val)
writeBytesField(tag, buffer)
Packed field writing methods:
writePackedVarint(tag, val)
writePackedSVarint(tag, val)
writePackedSFixed32(tag, val)
writePackedSFixed64(tag, val)
writePackedBoolean(tag, val)
writePackedFloat(tag, val)
writePackedDouble(tag, val)
Scalar writing methods:
writeVarint(val)
writeSVarint(val)
writeSFixed32(val)
writeSFixed64(val)
writeBoolean(val)
writeFloat(val)
writeDouble(val)
writeString(val)
writeBytes(buffer)
Message writing methods:
writeMessage(tag, fn[, obj])
writeRawMessage(fn[, obj])
Misc methods:
realloc(minBytes)
- pad the underlying buffer size to accommodate the given number of bytes;
note that the size increases exponentially, so it won't necessarily equal the size of data writtenfinish()
- make the current buffer ready for reading and return the data as a buffer sliceFor an example of a real-world usage of the library, see vector-tile-js.
If installed globally, pbf
provides a binary that compiles proto
files into JavaScript modules. Usage:
$ pbf <proto_path> [--no-write] [--no-read] [--legacy]
The --no-write
and --no-read
switches remove corresponding code in the output.
The --legacy
switch makes it generate a CommonJS module instead of ESM.
Pbf
will generate read<Identifier>
and write<Identifier>
functions for every message in the schema. For nested messages, their names will be concatenated — e.g. Message
inside Test
will produce readTestMessage
and writeTestMessage
functions.
read(pbf)
- decodes an object from the given Pbf
instance.write(obj, pbf)
- encodes an object into the given Pbf
instance (usually empty).The resulting code is clean and simple, so it's meant to be customized.
FAQs
a low-level, lightweight protocol buffers implementation in JavaScript
The npm package pbf receives a total of 1,358,177 weekly downloads. As such, pbf popularity was classified as popular.
We found that pbf demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 28 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.