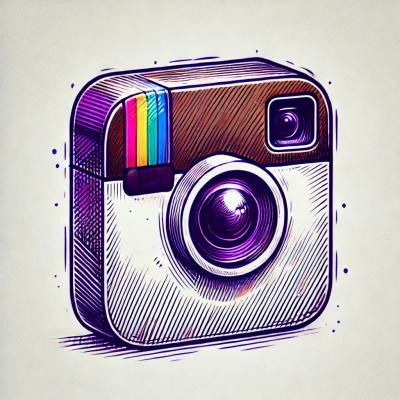
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
pd-redis-model
Advanced tools
To facilitate database operation in Redis
npm install -save pd-redis-model
var User = require('pd-redis-model')('user');
'user' is the saved name of the model, all capital letters are converted into lower-case letters
var profile = {
email : 'myletter@email.com',
password : 'abc123'
};
var creatingPromise = User.create(profile);
The returning value of User.create is a q.Promise The newly created record will have a sequence id which is unique of the type. It can be fetched by using 'then' of the promise as follows
creatingPromise.then(function(sid){
//do something to the returned sid...
});
var readingPromise = User.findBySid('1')
Again the returning value of User.findBySid is a q.Promise. The record information can be read by using 'then' as follows
readingPromise.then(function(rec){
// => rec's content is: { 'pd-sid' : '1', email: 'myletter@email.com' ....}
});
var option = {
latest: (new Date()).getTime(), //the ending time point of list
earliest : 0 //the starting time point of list
}
var listPromise = User.range(option);
It will return all available records in a list in descending order of time. They can be reached as follows
listPromise.then(function(list){
// list's content ==>
// [
// {'pd-sid' : 1 , email : 'myletter1@email.com' ... },
// {'pd-sid' : 2, email: 'myletter2@email.com' ...}
// .....
// ]
});
var amountPromise = User.amount();
amountPromise.then(function(amount){
// amount ==> 1
});
var profile = {
'pd-sid' : 1
password : '123abc',
status : 'online'
};
var updatePromise = User.modify(profile);
The 'pd-sid' which is the auto-increase id field can never be updated but it should be assigned value to specify which record is to be updated.
var removePromise = User.remove('1') //'1' is the user record's sid
For more details, check Base Record
User.setUniqueDef('account-name', ['email']);
var readPromise = User.withUnique('account-name').findBy('myhost@email.com');
check Set unique fields for more details
User.needInputOf(['email', 'password'])
User.eachInputOf('email').mustMatch(function(val){
return require('validator').isEmail(val);
})
User.eachInputOf('password').mustMatch(/^\w{6,30}$/);
check Set non-empty fields for more details
var Posts = require('pd-redis-model')('post');
User.mother(Posts);
var userSid = '1';
var postProfile = {
content : 'Hello'
};
User.PostOwner(userSid).bear(postProfile);
var postSid = '12';
User.PostOwner(userSid).hasKid(postSid);
User.PostOwner(userSid).findKids({
latest: (new Date()).getTime(),
earliest: 0
});
Posts.UserKid(postSid).getParent();
check Set parenthood for more details
FAQs
To facilitate database operation in Redis
The npm package pd-redis-model receives a total of 2 weekly downloads. As such, pd-redis-model popularity was classified as not popular.
We found that pd-redis-model demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.