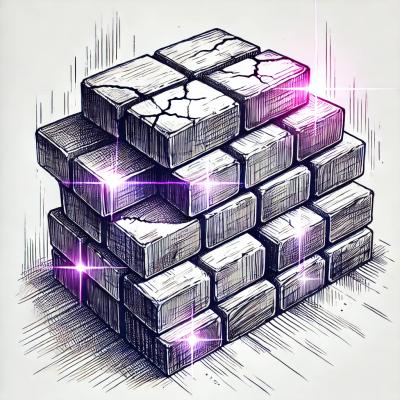
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
pdfkit-table-fx
Advanced tools
PdfKit Table. Helps to draw informations in simple tables using pdfkit. #server-side. Generate pdf tables with javascript (PDFKIT plugin)
Helps to draw informations in simple tables using pdfkit. #server-side.
view pdf example | color pdf | full code example | server example | json example | all
npm install pdfkit-table
// requires
const fs = require("fs");
const PDFDocument = require("pdfkit-table");
// init document
let doc = new PDFDocument({ margin: 30, size: 'A4' });
// save document
doc.pipe(fs.createWriteStream("./document.pdf"));
;(async function createTable(){
// table
const table = {
title: '',
headers: [],
datas: [ /* complex data */ ],
rows: [ /* or simple data */ ],
};
// the magic (async/await)
await doc.table(table, { /* options */ });
// -- or --
// doc.table(table).then(() => { doc.end() }).catch((err) => { })
// if your run express.js server
// to show PDF on navigator
// doc.pipe(res);
// done!
doc.end();
})();
// router - Node + Express.js
app.get('/create-pdf', async (req, res) => {
// ...await table code
// if your run express.js server
// to show PDF on navigator
doc.pipe(res);
// done!
doc.end();
});
;(async function(){
// table
const table = {
title: "Title",
subtitle: "Subtitle",
headers: [ "Country", "Conversion rate", "Trend" ],
rows: [
[ "Switzerland", "12%", "+1.12%" ],
[ "France", "67%", "-0.98%" ],
[ "England", "33%", "+4.44%" ],
],
};
// A4 595.28 x 841.89 (portrait) (about width sizes)
// width
await doc.table(table, {
width: 300,
});
// or columnsSize
await doc.table(table, {
columnsSize: [ 200, 100, 100 ],
});
// done!
doc.end();
})();
;(async function(){
// table
const table = {
title: "Title",
subtitle: "Subtitle",
headers: [
{ label: "Name", property: 'name', width: 60, renderer: null },
{ label: "Description", property: 'description', width: 150, renderer: null },
{ label: "Price 1", property: 'price1', width: 100, renderer: null },
{ label: "Price 2", property: 'price2', width: 100, renderer: null },
{ label: "Price 3", property: 'price3', width: 80, renderer: null },
{ label: "Price 4", property: 'price4', width: 43,
renderer: (value, indexColumn, indexRow, row, rectRow, rectCell) => { return `U$ ${Number(value).toFixed(2)}` }
},
],
// complex data
datas: [
{
name: 'Name 1',
description: 'Lorem ipsum dolor sit amet, consectetur adipiscing elit. Aenean mattis ante in laoreet egestas. ',
price1: '$1',
price3: '$ 3',
price2: '$2',
price4: '4',
},
{
options: { fontSize: 10, separation: true},
name: 'bold:Name 2',
description: 'bold:Lorem ipsum dolor.',
price1: 'bold:$1',
price3: {
label: 'PRICE $3', options: { fontSize: 12 }
},
price2: '$2',
price4: '4',
},
// {...},
],
// simeple data
rows: [
[
"Apple",
"Nullam ut facilisis mi. Nunc dignissim ex ac vulputate facilisis.",
"$ 105,99",
"$ 105,99",
"$ 105,99",
"105.99",
],
// [...],
],
};
// the magic
doc.table(table, {
prepareHeader: () => doc.font("Helvetica-Bold").fontSize(8),
prepareRow: (row, indexColumn, indexRow, rectRow, rectCell) => {
doc.font("Helvetica").fontSize(8);
indexColumn === 0 && doc.addBackground(rectRow, 'blue', 0.15);
},
});
// done!
doc.end();
})();
;(async function(){
// renderer function inside json file
const tableJson = '{
"headers": [
{ "label":"Name", "property":"name", "width":100 },
{ "label":"Age", "property":"age", "width":100 },
{ "label":"Year", "property":"year", "width":100 }
],
"datas": [
{ "name":"bold:Name 1", "age":"Age 1", "year":"Year 1" },
{ "name":"Name 2", "age":"Age 2", "year":"Year 2" },
{ "name":"Name 3", "age":"Age 3", "year":"Year 3",
"renderer": "function(value, i, irow){ return value + `(${(1+irow)})`; }"
}
],
"rows": [
[ "Name 4", "Age 4", "Year 4" ]
],
"options": {
"width": 300
}
}';
// the magic
doc.table(tableJson);
// done!
doc.end();
})();
;(async function(){
// json file
const json = require('./table.json');
// if json file is array
Array.isArray(json) ?
// any tables - array
await doc.tables(json) :
// one table - string
await doc.table(json) ;
// done!
doc.end();
})();
Array.<object>
| JSON
Array.<object>
| Array.[]
String
String
Number
String
String
String
Number
String
String
Number
Function
function( value, indexColumn, indexRow, row, rectRow, rectCell ) { return value }Array.<object>
Array.[]
String
| Object
String
| Object
Properties | Type | Default | Description |
---|---|---|---|
label | String | undefined | description |
property | String | undefined | id |
width | Number | undefined | width of column |
align | String | left | alignment |
valign | String | undefined | vertical alignment. ex: valign: "center" |
headerColor | String | grey or #BEBEBE | color of header |
headerOpacity | Number | 0.5 | opacity of header |
headerAlign | String | left | only header |
columnColor or | String | undefined | color of column |
columnOpacity or | Number | undefined | opacity of column |
renderer | Function | Function | function( value, indexColumn, indexRow, row, rectRow, rectCell ) { return value } |
const table = {
// simple headers only with ROWS (not DATAS)
headers: ['Name', 'Age'],
// simple content
rows: [
['Jack', '32'], // row 1
['Maria', '30'], // row 2
]
};
const table = {
// complex headers work with ROWS and DATAS
headers: [
{ label:"Name", property: 'name', width: 100, renderer: null },
{ label:"Age", property: 'age', width: 100, renderer: (value) => `U$ ${Number(value).toFixed(1)}` },
],
// complex content
datas: [
{ name: 'bold:Jack', age: 32, },
// age is object value with style options
{ name: 'Maria', age: { label: 30 , options: { fontSize: 12 }}, },
],
// simple content (works fine!)
rows: [
['Jack', '32'], // row 1
['Maria', '30'], // row 2
]
};
Properties | Type | Default | Description |
---|---|---|---|
title | String Object | undefined | title |
subtitle | String Object | undefined | subtitle |
width | Number | undefined | width of table |
x | Number | undefined | position x (left). To reset x position set "x: null" |
y | Number | undefined | position y (top) |
divider | Object | undefined | define divider lines |
columnsSize | Array | undefined | define sizes |
columnSpacing | Number | 5 | |
padding | Number Array | 1 or [1, 5] | |
addPage | Boolean | false | add table on new page |
hideHeader | Boolean | false | hide header |
minRowHeight | Number | 0 | min row height |
prepareHeader | Function | Function | () |
prepareRow | Function | Function | (row, indexColumn, indexRow, rectRow, rectCell) => {} |
const options = {
// properties
title: "Title", // { label: 'Title', fontSize: 30, color: 'blue', fontFamily: "./fonts/type.ttf" },
subtitle: "Subtitle", // { label: 'Subtitle', fontSize: 20, color: 'green', fontFamily: "./fonts/type.ttf" },
width: 500, // {Number} default: undefined // A4 595.28 x 841.89 (portrait) (about width sizes)
x: 0, // {Number} default: undefined | To reset x position set "x: null"
y: 0, // {Number} default: undefined |
divider: {
header: { disabled: false, width: 2, opacity: 1 },
horizontal: { disabled: false, width: 0.5, opacity: 0.5 },
},
padding: 5, // {Number} default: 0
columnSpacing: 5, // {Number} default: 5
hideHeader: false,
minRowHeight: 0,
// functions
prepareHeader: () => doc.font("Helvetica-Bold").fontSize(8), // {Function}
prepareRow: (row, indexColumn, indexRow, rectRow, rectCell) => doc.font("Helvetica").fontSize(8), // {Function}
}
{Booleon}
{Number}
{String}
datas: [
// options row
{ name: 'Jack', options: { fontSize: 10, fontFamily: 'Courier-Bold', separation: true } },
]
datas: [
// bold
{ name: 'bold:Jack' },
// size{n}
{ name: 'size20:Maria' },
{ name: 'size8:Will' },
// normal
{ name: 'San' },
]
{Number}
{String}
datas: [
// options cell | value is object | label is string
{ name: { label: 'Jack', options: { fontSize: 10, fontFamily: 'Courier-Bold' } },
]
options: {
minRowHeight: 30, // pixel
}
let localType = "./font/Montserrat-Regular.ttf";
const table = {
title: { label: 'Title Object 2', fontSize: 30, color: 'blue', fontFamily: localType },
}
options: {
hideHeader: true,
}
options: {
// divider lines
divider: {
header: {disabled: false, width: 0.5, opacity: 0.5},
horizontal: {disabled: true, width: 0.5, opacity: 0.5},
},
}
;(async function(){
// create document
const doc = new PDFDocument({ margin: 30, });
// to save on server
doc.pipe(fs.createWriteStream("./my-table.pdf"));
// tables
await doc.table(table, options);
await doc.table(table, options);
await doc.table(table, options);
// done
doc.end();
})();
~~doc.table(table, options, callback)~~;
headers: [
{label:"Name", property:"name", valign: "center", headerAlign:"right", headerColor:"#FF0000", headerOpacity:0.5 }
]
headers: [
{label:"Name", property:"name", headerColor:"#FF0000", headerOpacity:0.5 }
]
headers: [
{label:"Name", property:"name", align:"center"}
]
{Function}
- Add background peer line.
{Function}
{Function}
- Add many tables.
{Boolean}
- Add table on new page.
{String}
{String}
{Object}
{Array}
(${(irow+2)})
; }" }The MIT License.
![]() |
Natan Cabral natancabral@hotmail.com https://github.com/natancabral/ |
FAQs
PdfKit Table. Helps to draw informations in simple tables using pdfkit. #server-side. Generate pdf tables with javascript (PDFKIT plugin)
The npm package pdfkit-table-fx receives a total of 51 weekly downloads. As such, pdfkit-table-fx popularity was classified as not popular.
We found that pdfkit-table-fx demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.