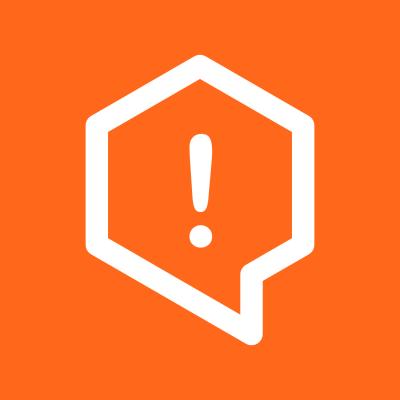
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
A web frontend framework that supports conditional and loop rendering, data binding, monitoring data changes, and automatic rendering. Previously known as QuickUI.
(Formerly known as PDQuickUI, renamed to QuickUI starting from version 0.6.0
)
QuickUI
is a front-end rendering framework derived from PDRenderKit, focusing on enhancing front-end framework features.
By integrating a virtual DOM, it rewrites the rendering logic to improve rendering efficiency, enabling faster data observation and automatic updates.
This project removes the prototype
extensions from PDRenderKit
to ensure compatibility and performance, making it suitable for complex applications.
It provides both module
and non-module
versions and changes the license from GPL-3.0
in PDRenderKit
to MIT
.
20kb
.npm i @pardnchiu/quickui
QuickUI
<!-- Version 0.6.0 and above -->
<script src="https://cdn.jsdelivr.net/npm/@pardnchiu/quickui@[VERSION]/dist/QuickUI.js"></script>
<!-- Version 0.5.4 and below -->
<script src="https://cdn.jsdelivr.net/npm/pdquickui@[VERSION]/dist/PDQuickUI.js"></script>
// Version 0.6.0 and above
import { QUI } from "https://cdn.jsdelivr.net/npm/@pardnchiu/quickui@[VERSION]/dist/QuickUI.esm.js";
// Version 0.5.4 and below
import { QUI } from "https://cdn.jsdelivr.net/npm/pdquickui@[VERSION]/dist/PDQuickUI.module.js";
QUI
const app = new QUI({
id: "", // Specify rendering element
data: {
// Custom DATA
},
event: {
// Custom EVENT
},
when: {
before_render: function () {
// Stop rendering
},
rendered: function () {
// Rendered
},
before_update: function () {
// Stop updating
},
updated: function () {
// Updated
},
before_destroy: function () {
// Stop destruction
},
destroyed: function () {
// Destroyed
}
}
});
Automatic Rendering: Automatically reloads when data changes are detected.
Attribute | Description |
---|---|
{{value}} | Inserts text into HTML tags and automatically updates with data changes. |
:path | Used with the temp tag to load HTML fragments from external files into the current page. |
:html | Replaces the element's innerHTML with text. |
:for | Supports formats like item in items , (item, index) in items , (key, value) in object . Iterates over data collections to generate corresponding HTML elements. |
:if :else-if :elif :else | Displays or hides elements based on specified conditions, enabling branching logic. |
:model | Binds data to form elements (e.g., input ), updating data automatically when input changes. |
:hide | Hides elements based on specific conditions. |
:animation | Specifies transition effects for elements, such as fade-in or expand , to enhance user experience. |
:mask | Controls block loading animations, supporting `true |
:[attr] | Sets element attributes, such as ID , class , image source, etc.Examples: :id /:class /:src /:alt /:href ... |
:[css] | Sets element CSS, such as margin, padding, etc. |
Examples: :background-color , :opacity , :margin , :top , :position ... | |
@[event] | Adds event listeners that trigger specified actions upon activation. Examples: @click /@input /@mousedown ... |
{{value}}
<h1>{{ title }}</h1>
<script>
const app = new QUI({
id: "app",
data: {
title: "test"
}
});
</script>
<body id="app">
<h1>test</h1>
</body>
:html
<section :html="html"></section>
<script>
const app = new QUI({
id: "app",
data: {
html: "<b>innerHtml</b>"
}
});
</script>
<body id="app">
<section>
<b>innerHtml</b>
</section>
</body>
[!NOTE] Ensure to disable local file restrictions in your browser or use a live server when testing.
:path
<h1>path heading</h1>
<p>path content</p>
<body id="app">
<temp :path="./test.html"></temp>
</body>
<script>
const app = new QUI({
id: "app"
});
</script>
<body id="app">
<!-- Directly inserted PATH content -->
<h1>path heading</h1>
<p>path content</p>
</body>
:for
<body id="app">
<ul>
<li :for="(item, index) in ary" :id="item" :index="index">{{ item }} {{ CALC(index + 1) }}</li>
</ul>
</body>
<script>
const app = new QUI({
id: "app",
data: {
ary: ["test1", "test2", "test3"]
}
});
</script>
<body id="app">
<li id="test1" index="0">test1 1</li>
<li id="test2" index="1">test2 2</li>
<li id="test3" index="2">test3 3</li>
</body>
<body id="app">
<ul>
<li :for="(key, val) in obj">
{{ key }}: {{ val.name }}
<ul>
<li :for="item in val.ary">
{{ item.name }}
<ul>
<li :for="(item1, index1) in item.ary1">
{{ CALC(index1 + 1) }}. {{ item1.name }} - ${{ item1.price }}
</li>
</ul>
</li>
</ul>
</li>
</ul>
</body>
<script>
const app = new QUI({
id: "app",
data: {
obj: {
food: {
name: "Food",
ary: [
{
name: 'Snacks',
ary1: [
{ name: 'Potato Chips', price: 10 },
{ name: 'Chocolate', price: 8 }
]
},
{
name: 'Beverages',
ary1: [
{ name: 'Juice', price: 5 },
{ name: 'Tea', price: 3 }
]
}
]
},
home: {
name: 'Home',
ary: [
{
name: 'Furniture',
ary1: [
{ name: 'Sofa', price: 300 },
{ name: 'Table', price: 150 }
]
},
{
name: 'Decorations',
ary1: [
{ name: 'Picture Frame', price: 20 },
{ name: 'Vase', price: 15 }
]
}
]
}
}
}
});
</script>
<body id="app">
<ul>
<li>food: Food
<ul>
<li>Snacks
<ul>
<li>1. Potato Chips - $10</li>
<li>2. Chocolate - $8</li>
</ul>
</li>
<li>Beverages
<ul>
<li>1. Juice - $5</li>
<li>2. Tea - $3</li>
</ul>
</li>
</ul>
</li>
<li>home: Home
<ul>
<li>Furniture
<ul>
<li>1. Sofa - $300</li>
<li>2. Table - $150</li>
</ul>
</li>
<li>Decorations
<ul>
<li>1. Picture Frame - $20</li>
<li>2. Vase - $15</li>
</ul>
</li>
</ul>
</li>
</ul>
</body>
<body id="app">
<h1 :if="heading == 1">{{ title }} {{ heading }}</h1>
<h2 :else-if="isH2">{{ title }} {{ heading }}</h2>
<h3 :else-if="heading == 3">{{ title }} {{ heading }}</h3>
<h4 :else>{{ title }} {{ heading }}</h4>
</body>
<script>
const app = new QUI({
id: "app",
data: {
heading: [Number|null],
isH2: [Boolean|null],
title: "test"
}
});
</script>
heading = 1
<body id="app">
<h1>test 1</h1>
</body>
heading = null && isH2 = true
<body id="app">
<h2>test </h2>
</body>
heading = 3 && isH2 = null
<body id="app">
<h3>test 3</h3>
</body>
heading = null && isH2 = null
<body id="app">
<h4>test </h4>
</body>
<body id="app"></body>
<script>
const test = new QUI({
id: "app",
data: {
hint: "hint 123",
title: "test 123"
},
render: () => {
return `
"{{ hint }}",
h1 {
style: "background: red;",
children: [
"{{ title }}"
]
}`
}
})
</script>
<body id="app">
hint 123
<h1 style="background: red;">test 123</h1>
</body>
<body id="app">
<input type="password" :model="password">
<button @click="show">test</button>
</body>
<script>
const app = new QUI({
id: "app",
data: {
password: null,
},
event: {
show: function(e){
alert("Password:", app.data.password);
}
}
});
</script>
<body id="app">
<button @click="test">test</button>
</body>
<script>
const app = new QUI({
id: "app",
event: {
test: function(e){
alert(e.target.innerText + " clicked");
}
}
});
</script>
[!NOTE] Supports simple settings using :[CSS property], directly binding data to style attributes.
<body id="app">
<button :width="width" :backdround-color="color">test</button>
</body>
<script>
const app = new QUI({
id: "app",
data: {
width: "100px",
color: "red"
}
});
</script>
<body id="app">
<button style="width: 100px; backdround-color: red;">test</button>
</body>
LENGTH()
<body id="app">
<p>Total: {{ LENGTH(array) }}</p>
</body>
<script>
const app = new QUI({
id: "app",
data: {
array: [1, 2, 3, 4]
}
});
</script>
<body id="app">
<p>Total: 4</p>
</body>
CALC()
<body id="app">
<p>calc: {{ CALC(num * 10) }}</p>
</body>
<script>
const app = new QUI({
id: "app",
data: {
num: 1
}
});
</script>
<body id="app">
<p>calc: 10</p>
</body>
UPPER()
/ LOWER()
<body id="app">
<p>{{ UPPER(test1) }} {{ LOWER(test2) }}</p>
</body>
<script>
const app = new QUI({
id: "app",
data: {
test1: "upper",
test2: "LOWER"
}
});
</script>
<body id="app">
<p>UPPER lower</p>
</body>
DATE(num, format)
<body id="app">
<p>{{ DATE(now, YYYY-MM-DD hh:mm:ss) }}</p>
</body>
<script>
const app = new QUI({
id: "app",
data: {
now: Math.floor(Date.now() / 1000)
}
});
</script>
<body id="app">
<p>2024-08-17 03:40:47</p>
</body>
:lazyload
<body id="app">
<img :lazyload="image">
</body>
<script>
const app = new QUI({
id: "app",
data: {
image: "test.jpg"
},
option: {
lazyload: true // Enable image lazy loading: true|false (default: true)
}
});
</script>
<body id="app">
<img src="test.jpg">
</body>
SVG
替換<svg width="24" height="24" viewBox="0 0 24 24" fill="none" xmlns="http://www.w3.org/2000/svg">
<line x1="18" y1="6" x2="6" y2="18" stroke="black" stroke-width="2" stroke-linecap="round"/>
<line x1="6" y1="6" x2="18" y2="18" stroke="black" stroke-width="2" stroke-linecap="round"/>
</svg>
<body id="app">
<temp-svg :src="svg"></temp-svg>
</body>
<script>
const app = new QUI({
id: "app",
data: {
svg: "test.svg",
},
option: {
svg: true // Enable SVG file transformation: true|false (default: true)
}
});
</script>
<body id="app">
<svg width="24" height="24" viewBox="0 0 24 24" fill="none" xmlns="http://www.w3.org/2000/svg">
<line x1="18" y1="6" x2="6" y2="18" stroke="black" stroke-width="2" stroke-linecap="round">
<line x1="6" y1="6" x2="18" y2="18" stroke="black" stroke-width="2" stroke-linecap="round">
</svg>
</body>
[!NOTE] If the format is an object, the multilingual content is directly configured. If the format is a string, the language file is dynamically loaded via fetch.
{
"greeting": "Hello",
"username": "Username"
}
<body id="app">
<h1>{{ i18n.greeting }}, {{ i18n.username }}: {{ username }}</h1>
<button @click="change" data-lang="zh">切換至中文</button>
<button @click="change" data-lang="en">Switch to English</button>
</body>
<script>
const app = new QUI({
id: "app",
data: {
username: "Pardn"
},
i18n: {
zh: {
greeting: "你好",
username: "用戶名"
},
en: "en.json",
},
i18nLang: "zh | en", // Select the displayed language
event: {
change: e => {
const _this = e.target;
const lang = _this.dataset.lang;
app.lang(lang);
},
}
});
</script>
i18nLang = zh
<body id="app">
<h1>你好, 用戶名: Pardn</h1>
<button data-lang="zh">切換至中文</button>
<button data-lang="en">Switch to English</button>
</body>
i18nLang = en
<body id="app">
<h1>Hello, Username: Pardn</h1>
<button data-lang="zh">切換至中文</button>
<button data-lang="en">Switch to English</button>
</body>
<body id="app"></body>
<script>
const app = new QUI({
id: "app",
when: {
before_render: function () {
// Stop rendering
// retuen false
},
rendered: function () {
// Rendered
},
before_update: function () {
// Stop updating
// retuen false
},
updated: function () {
// Updated
},
before_destroy: function () {
// Stop destruction
// retuen false
},
destroyed: function () {
// Destroyed
}
}
});
</script>
<body id="app">
<input type="text" :model="test">
<button @click="get">Test</button>
</body>
<script>
const app = new QUI({
id: "app",
data: {
// Value bound to the input
test: 123
},
event: {
get: _ => {
// Show an alert with the value of test on button click
alert(app.data.test);
},
set: _ => {
let dom = document.createElement("button");
// Assign the button click event to the get function
dom.onclick = app.event.get;
app.body.append(dom);
}
}
});
</script>
This project is licensed under the MIT License.
Contact me for the complete unobfuscated source code.
Feel free to modify and use for commercial purposes with the following licensing options:
Powered by @pardnchiu/quickui
copyright notice: $7,500.©️ 2024 邱敬幃 Pardn Chiu
FAQs
A web frontend framework that supports conditional and loop rendering, data binding, monitoring data changes, and automatic rendering. Previously known as QuickUI.
The npm package pdquickui receives a total of 7 weekly downloads. As such, pdquickui popularity was classified as not popular.
We found that pdquickui demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.