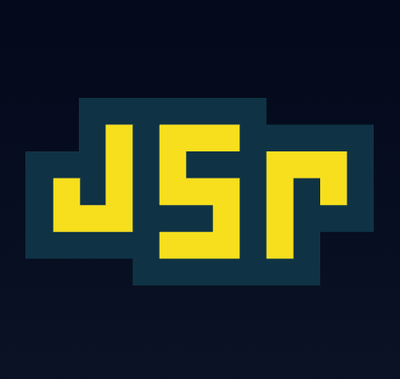
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
peek-readable
Advanced tools
The peek-readable npm package provides utilities for reading from streams and buffers without consuming the data, allowing you to 'peek' at the data before deciding how to process it. This is particularly useful in scenarios where the type of processing depends on the content of the data itself.
Peeking data from a stream
This feature allows you to peek at the first few bytes of a stream to determine its content without consuming those bytes, enabling further processing based on the peeked data.
const {ReadableStreamPeeker} = require('peek-readable');
const fs = require('fs');
const stream = fs.createReadStream('example.txt');
const peeker = new ReadableStreamPeeker(stream);
peeker.peek(10).then(buffer => {
console.log('Peeked data:', buffer.toString());
});
Peeking data from a buffer
This feature enables peeking at data within a buffer, which can be useful for preliminary checks before processing the entire buffer.
const {BufferPeeker} = require('peek-readable');
const buffer = Buffer.from('Hello, world!');
const peeker = new BufferPeeker(buffer);
const peekedData = peeker.peek(5);
console.log('Peeked data:', peekedData.toString());
Similar to peek-readable, buffer-peek-stream allows peeking into streams without consuming the data. It compares by offering a similar API but is specifically tailored for Node.js streams, potentially offering optimizations for stream handling.
A promise based asynchronous stream reader, which makes reading from a stream easy.
Allows to read and peek from a Readable Stream
This module is used by strtok3
The peek-readable
contains one class: StreamReader
, which reads from a stream.Readable.
StreamReader
is used to read from Node.js stream.Readable.WebStreamReader
is used to read from ReadableStreamModule: version 5 migrated from CommonJS to pure ECMAScript Module (ESM). JavaScript is compliant with ECMAScript 2019 (ES10). Requires Node.js ≥ 14.16 engine.
npm install --save peek-readable
Both StreamReader
and WebStreamReader
implement the IStreamReader interface.
IStreamReader
InterfaceThe IStreamReader
interface defines the contract for a stream reader,
which provides methods to read and peek data from a stream into a Uint8Array
buffer.
The methods are asynchronous and return a promise that resolves with the number of bytes read.
peek
functionThis method allows you to inspect data from the stream without advancing the read pointer. It reads data into the provided Uint8Array at a specified offset but does not modify the stream's internal position, allowing you to look ahead in the stream.
peek(uint8Array: Uint8Array, offset: number, length: number): Promise<number>
Parameters:
uint8Array
: Uint8Array
: The buffer into which the data will be peeked.
This is where the peeked data will be stored.offset
: number
: The offset in the Uint8Array where the peeked data should start being written.length
: number
: The number of bytes to peek from the stream.Returns Promise<number>
:
A promise that resolves with the number of bytes actually peeked into the buffer.
This number may be less than the requested length if the end of the stream is reached.
read
functionread(buffer: Uint8Array, offset: number, length: number): Promise<number>
Parameters:
uint8Array
: Uint8Array
: The buffer into which the data will be read.
This is where the read data will be stored.offset
: number
: The offset in the Uint8Array where the read data should start being written.length
: number
: The number of bytes to read from the stream.Returns Promise<number>
:
A promise that resolves with the number of bytes actually read into the buffer.
This number may be less than the requested length if the end of the stream is reached.
abort
functionAbort active asynchronous operation (read
or peak
) before it has completed.
abort(): Promise<void>
In the following example we read the first 16 bytes from a stream and store them in our buffer. Source code of examples can be found here.
import fs from 'node:fs';
import { StreamReader } from 'peek-readable';
(async () => {
const readable = fs.createReadStream('JPEG_example_JPG_RIP_001.jpg');
const streamReader = new StreamReader(readable);
const uint8Array = new Uint8Array(16);
const bytesRead = await streamReader.read(uint8Array, 0, 16);;
// buffer contains 16 bytes, if the end-of-stream has not been reached
})();
End-of-stream detection:
(async () => {
const fileReadStream = fs.createReadStream('JPEG_example_JPG_RIP_001.jpg');
const streamReader = new StreamReader(fileReadStream);
const buffer = Buffer.alloc(16); // or use: new Uint8Array(16);
try {
await streamReader.read(buffer, 0, 16);
// buffer contains 16 bytes, if the end-of-stream has not been reached
} catch(error) {
if (error instanceof EndOfStreamError) {
console.log('End-of-stream reached');
}
}
})();
With peek
you can read ahead:
import fs from 'node:fs';
import { StreamReader } from 'peek-readable';
const fileReadStream = fs.createReadStream('JPEG_example_JPG_RIP_001.jpg');
const streamReader = new StreamReader(fileReadStream);
const buffer = Buffer.alloc(20);
(async () => {
let bytesRead = await streamReader.peek(buffer, 0, 3);
if (bytesRead === 3 && buffer[0] === 0xFF && buffer[1] === 0xD8 && buffer[2] === 0xFF) {
console.log('This is a JPEG file');
} else {
throw Error('Expected a JPEG file');
}
bytesRead = await streamReader.read(buffer, 0, 20); // Read JPEG header
if (bytesRead === 20) {
console.log('Got the JPEG header');
} else {
throw Error('Failed to read JPEG header');
}
})();
FAQs
Read and peek from a readable stream
The npm package peek-readable receives a total of 3,221,405 weekly downloads. As such, peek-readable popularity was classified as popular.
We found that peek-readable demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.