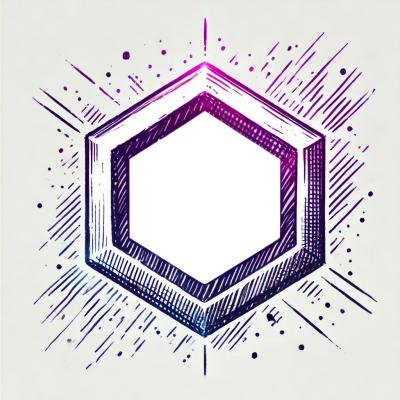
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
peer-wire-protocol
Advanced tools
peer-wire-protocol is a node stream implementation of the peer wire protocol specification. The protocol is the main communication layer when transferring files using BitTorrent and is used by peerflix.
It is available through npm:
npm install peer-wire-protocol
Since the protocol is implemented as a stream all you have to do is pipe some to and from it
var pwp = require('peer-wire-protocol');
var net = require('net');
net.createServer(function(socket) {
var wire = pwp();
// pipe to and from the protocol
socket.pipe(wire).pipe(socket);
wire.on('handshake', function(infoHash, peerId) {
// lets emit a handshake of our own as well
wire.handshake(Buffer.from('my info hash'), Buffer.from('my peer id'));
});
wire.on('unchoke', function() {
console.log('peer is no longer choking us: '+wire.peerChoking);
});
}).listen(6881);
Send and receive a handshake from the peer. This is the first message.
// send a handshake to the peer
wire.handshake(infoHash, peerId, {dht:true});
wire.on('handshake', function(infoHash, peerId, extensions) {
// receive a handshake
});
Both the infoHash
and the peerId
should be 20 bytes
Check if you or the peer is choking
wire.peerChoking; // is the peer choking us?
wire.amChoking; // are we choking the peer?
wire.on('choke', function() {
// the peer is now choking us
});
wire.on('unchoke', function() {
// peer is no longer choking us
});
See if you or the peer is interested
wire.peerInterested; // is the peer interested in us?
wire.amInterested; // are we interested in the peer?
wire.on('interested', function() {
// peer is now interested
});
wire.on('uninterested', function() {
// peer is no longer interested
});
Exchange piece information with the peer
// send a bitfield to the peer
wire.bitfield(buffer);
wire.on('bitfield', function(bitfield) {
// bitfield received from the peer
});
// send a have message indicating that you have a piece
wire.have(pieceIndex);
wire.on('have', function(pieceIndex) {
// peer has sent you a have message
});
You can always see which pieces the peer have
wire.peerPieces[i]; // returns true if peer has piece i
Send and respond to requests for pieces
// request a piece from a peer
wire.request(pieceIndex, offset, length, function(err, block) {
if (err) {
// there was an error (peer has started choking us etc)
return;
}
// got piece
});
// cancel a request to a peer
wire.cancel(pieceIndex, offset, length);
// receive a request from a peer
wire.on('request', function(pieceIndex, offset, length, callback) {
// ... read piece ...
callback(null, piece); // respond back to the peer
});
wire.requests; // list of requests we currently have pending {piece, offset, length}
wire.peerRequests; // list of requests the peer currently have pending {piece, offset, length}
You can set a request timeout if you want to
wire.setTimeout(5000); // head request should take a most 5s to finish
If the timeout is triggered the request callback is called with an error and a timeout
event is emitted.
You can set the extensions flag dht
in the handshake to true
if you participate in the torrent dht.
Afterwards you can send your dht port
// send your port to the peer
wire.port(dhtPort);
wire.on('port', function(dhtPort) {
// peer has sent a port to us
});
You can enable the keep-alive ping (triggered every 60s)
// starts the keep alive
wire.setKeepAlive(true);
wire.on('keep-alive', function() {
// peer sent a keep alive - just ignore it
});
Check how many bytes you have uploaded and download
wire.uploaded; // number of bytes uploaded
wire.downloaded; // number of bytes downloaded
wire.on('download', function(numberOfBytes) {
...
});
wire.on('upload', function(numberOfBytes) {
...
});
MIT
FAQs
Stream implementing the peer wire protocol used in bittorrent
The npm package peer-wire-protocol receives a total of 0 weekly downloads. As such, peer-wire-protocol popularity was classified as not popular.
We found that peer-wire-protocol demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.