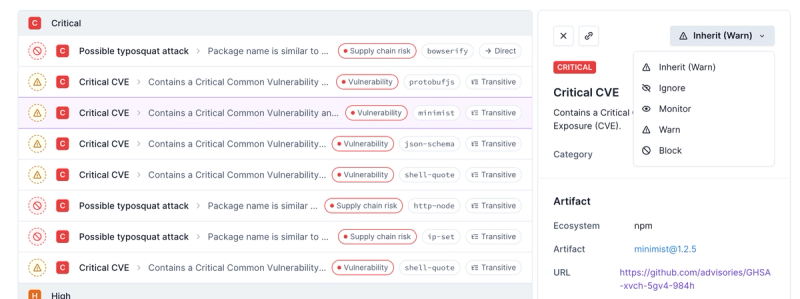
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
pem
Advanced tools
Changelog
Readme
Create private keys and certificates with node.js
Install with npm
npm install pem
Here are some examples for creating an SSL key/cert on the fly, and running an HTTPS server on port 443. 443 is the standard HTTPS port, but requires root permissions on most systems. To get around this, you could use a higher port number, like 4300, and use https://localhost:4300 to access your server.
var https = require('https'),
pem = require('pem');
pem.createCertificate({days:1, selfSigned:true}, function(err, keys){
https.createServer({key: keys.serviceKey, cert: keys.certificate}, function(req, res){
res.end("o hai!")
}).listen(443);
});
var https = require('https'),
pem = require('pem'),
express = require('express');
pem.createCertificate({days:1, selfSigned:true}, function(err, keys){
var app = express();
app.get('/', requireAuth, function(req, res){
res.send("o hai!");
});
https.createServer({key: keys.serviceKey, cert: keys.certificate}, app).listen(443);
});
Use createPrivateKey
for creating private keys
pem.createPrivateKey(keyBitsize, callback)
Where
{key}
Use createCSR
for creating certificate signing requests
pem.createCSR(options, callback)
Where
{csr, clientKey}
Possible options are the following
clientKey
is undefined, bit size to use for generating a new key (defaults to 2048)md5
, sha1
or sha256
, defaults to sha256
)localhost
)Array
) of subjectAltNames in the subjectAltName field (optional)Use createCertificate
for creating private keys
pem.createCertificate(options, callback)
Where
{certificate, csr, clientKey, serviceKey}
Possible options include all the options for createCSR
- in case csr
parameter is not defined and a new
CSR needs to be generated.
In addition, possible options are the following
serviceKey
is not defined, use clientKey
for signingUse getPublicKey
for exporting a public key from a private key, CSR or certificate
pem.getPublicKey(certificate, callback)
Where
{publicKey}
Use readCertificateInfo
for reading subject data from a certificate or a CSR
pem.readCertificateInfo(certificate, callback)
Where
{country, state, locality, organization, organizationUnit, commonName, emailAddress, validity{start, end}, san{dns, ip}? }
? san is only present if the CSR or certificate has SAN entries.
Use getFingerprint
to get the SHA1 fingerprint for a certificate
pem.getFingerprint(certificate, callback)
Where
{fingerprint}
Use getModulus
to get the modulus for a certificate, a CSR or a private key. Modulus can be useful to check that a Private Key Matches a Certificate
pem.getModulus(certificate, callback)
Where
{modulus}
MIT
FAQs
Create private keys and certificates with node.js and io.js
The npm package pem receives a total of 245,609 weekly downloads. As such, pem popularity was classified as popular.
We found that pem demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.