Perf-marks



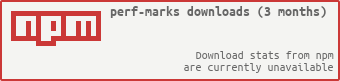

The simplest and lightweight solution for User Timing API in Javascript. Simple how it should be.
Contributing
Please check our contributing.md to know more about setup and how to contribute.
Setup and installation
Make sure that you are using the NodeJS version is the same as .nvmrc
file version. If you don't have this version please use a version manager such as nvm
or n
to manage your local nodejs versions.
Please make sure that you are using NodeJS version 6.10.2
Assuming that you are using nvm
, please run the commands inside this folder:
$ nvm install $(cat .nvmrc);
$ nvm use $(cat .nvmrc);
In Windows, please install NodeJS using one of these options:
Via NVM Windows
package: Dowload via this link. After that, run the commands:
$ nvm install $(cat .nvmrc);
$ nvm use $(cat .nvmrc);
Via Chocolatey:
$ choco install nodejs.install -version 6.10.2
Install yarn
We use yarn
as our package manager instead of npm
Install it following these steps
After that, just navigate to your local repository and run
$ yarn install
Demo
Try out our demo on Stackblitz!

Run the app
$ yarn start
Run the tests
$ yarn test
Run the build
$ yarn build
PerfMarks
This service exposes a few different methods with which you can interact with feature toggle service.
PerfMarks.start(markName)
Adds the user timing api marker instrumentation in your application.
import * as PerfMarks from 'perf-marks';
...
PerfMarks.start('name-of-your-mark');
...
PerfMarks.end(markName)
Returns the results for the specified marker
import * as PerfMarks from 'perf-marks';
...
PerfMarks.start('name-of-your-mark');
...
const markResults: PerfMarks.PerfMarksPerformanceEntry = PerfMarks.end('name-of-your-mark');
PerfMarks.clear(markName)
Removes the specified marker
import * as PerfMarks from 'perf-marks';
...
PerfMarks.start('name-of-your-mark');
...
PerfMarks.clear('name-of-your-mark');
...
PerfMarks.clearAll()
Removes all the marker
import * as PerfMarks from 'perf-marks';
...
PerfMarks.start('name-of-your-mark');
...
PerfMarks.clearAll();
...
PerfMarks.getNavigationMarker()
Gets the marks for navigation
loaded
import * as PerfMarks from 'perf-marks';
...
const markResults: PerfMarksPerformanceNavigationTiming = PerfMarks.getNavigationMarker();
...
PerfMarks.getEntriesByType(markName)
Gets the result for all marks that matches with the given mark name
import * as PerfMarks from 'perf-marks';
...
PerfMarks.start('name-of-your-mark');
PerfMarks.start('another-name-of-your-mark');
...
const markResult: PerfMarksPerformanceNavigationTiming[] = PerfMarks.getEntriesByType('name-of-your-mark');
...
PerfMarks.isUserTimingAPISupported
Boolean with the result of the check if User Timing API is supported for the current browser/NodeJS version
import * as PerfMarks from 'perf-marks';
...
PerfMarks.start('name-of-your-mark');
PerfMarks.start('another-name-of-your-mark');
...
const markResult: PerfMarksPerformanceNavigationTiming[] = PerfMarks.getEntriesByType('name-of-your-mark');
...
Entrypoints
These are entrypoints for specific components to be used carefully by the consumers. If you're using one of these entrypoints we are assuming you know what you are doing. So it means that code-splitting and tree-shaking should be done on the consumer/product side.
Exposed entrypoints
perf-marks/marks
: it has all the methods for marks
perf-marks/entries
: it has all the methods to get entries
getNavigationMarker
getEntriesByType
Publish
this project is using np
package to publish, which makes things straightforward. EX: np <patch|minor|major>
For more details, please check np package on npmjs.com
Author
Wilson Mendes (willmendesneto)